JToggleButton Component in Java
Submitted by donbermoy on Wednesday, November 19, 2014 - 22:07.
This is a tutorial in which we will going to create a program that has the JToggleButton Component using Java. The JToggleButton is a button which implements of a two-state button; an on or off button.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jToggleButtonComponent.java.
2. Import the following packages:
3. Initialize your variable in your Main, variable frame for creating JFrame, and variable toggleBtn for JToggleButton.
4. Now, we will add an ActionListener to the toggle button. This trigger to have an ActionEvent when clicking the toggle button. Have this code below:
As you have seen the code above, we have created again a JToggleButton having a variable named of tBtn. We used the method getSource to get the action out of the JToggleButton. When clicking the JToggleButton to determine if it's on or off, we will use isSelected method. When we click first the toggle button, it will prompt to the user that the button is on using the JOptionPane. When clicking again the button, it will display that the button is off.
5. Now, add the JToggleButton variable to the frame using the default BorderLayout of north position of the getContentPane method. Lastly, set the size, visibility, and the close operation of the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.event.*; // used to access the ActionEvent and ActionListener class
- import javax.swing.*; // used to access the JFrame, JOptionPane, JToggleButton
- if (tBtn.isSelected()) {
- } else {
- }
- }
- });
- frame.getContentPane().add(toggleBtn, "North");
- frame.setSize(300, 125);
- frame.setVisible(true);
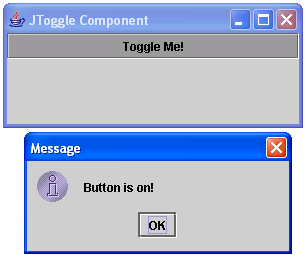
- import java.awt.event.*; // used to access the ActionEvent and ActionListener class
- import javax.swing.*; // used to access the JFrame, JOptionPane, JToggleButton
- public class jToggleButtonComponent {
- if (tBtn.isSelected()) {
- } else {
- }
- }
- });
- frame.getContentPane().add(toggleBtn, "North");
- frame.setSize(300, 125);
- frame.setVisible(true);
- }
- }
Add new comment
- 36 views