Get day, day of week, day of month, day of year, month, and year values from the current date using Java
Submitted by donbermoy on Thursday, May 29, 2014 - 21:45.
In this tutorial, we will create a program that gets day, day of week, day of month, day of year, month, and year values from the current date using Java. I have already discussed how to get the date and time, but here in this program, we will breakdown and get the complete information on the current date.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of subString.java.
2. Import java.util package because in this library, Calendar abstract class is there.
3. In your Main, instantiate variable clndr as a Calendar class to get an instance.
To display the current date with the time:
To display the day from the current date:
To display the month from the current date:
To display the year from the current date:
To display the day of the week from the current date:
To display the day of the month from the current date:
To display the day of the year from the current date:
Press F5 to run it.
Output:
Full source code:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.util.*;
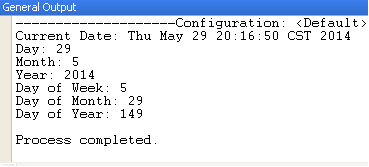
- import java.util.*;
- public class dateValues
- {
- {
- }
- }