Validate Phone Number using C#
Submitted by donbermoy on Friday, July 18, 2014 - 18:55.
Today in C#, I will teach you how to create a program that will validate an inputted phone number using C#.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio 2010: Go to File, click New Project, and choose Windows Application.
2. Next, add one TextBox named TextBox1 which will be used to input the phone number. Add also one Button named Button1 to know if the phone number inputted is valid or not. Design your interface like this one below:
3. Import this namespace and put this at the top most part of your code tab:
The namespace above provides regular expression functionality that may be used from any platform or language that runs within the Microsoft .NET Framework and it determines whether a particular string conforms to a regular expression pattern.
4. Put this code for the Button1_Click. This will trigger to decide whether the inputted phone number is valid or not.
We initialized phonenumber to hold the values of our regular expression ("\d{4}-\d{3}-\d{4}") which means it has a digit of 4 - digit of 3 - and digit of 4. The "d" there indicates the number of digits inside the expression.
Next, we use the IsMatch function to compare the value inputted in our textbox to the regular expression of the phonenumber variable. It has to match with the expression of 0000-000-0000 format. If match, it will display "Valid phone number!" and otherwise "Not Valid phone number!".
Invalid phone number
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
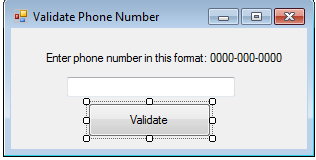
- using System.Text.RegularExpressions;
- private void Button1_Click(System.Object sender, System.EventArgs e)
- {
- if (phonenumber.IsMatch(TextBox1.Text))
- {
- MessageBox.Show("Valid phone number!");
- }
- else
- {
- MessageBox.Show("Not Valid phone number!");
- }
- }
Output:
Valid phone number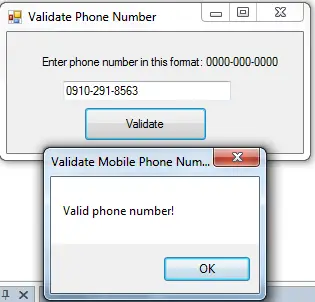
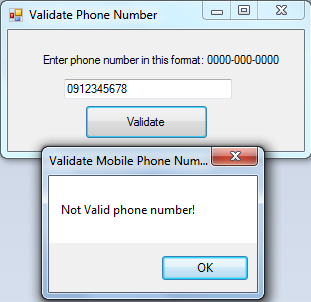
Add new comment
- 2669 views