File Handling – Create and Retrieve Log File in C#.
Submitted by janobe on Friday, June 21, 2019 - 17:34.
In this tutorial, I will teach you how to create and retrieve a log file in c#. Logs are very important because it records every transaction that the system has executed. In this way, you can track the error made by the system. Let’s get started.
Double click a Button and add the following code for inserting/appending the text message logs and retrieving automatically when the button is clicked
The complete source code is included. You can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.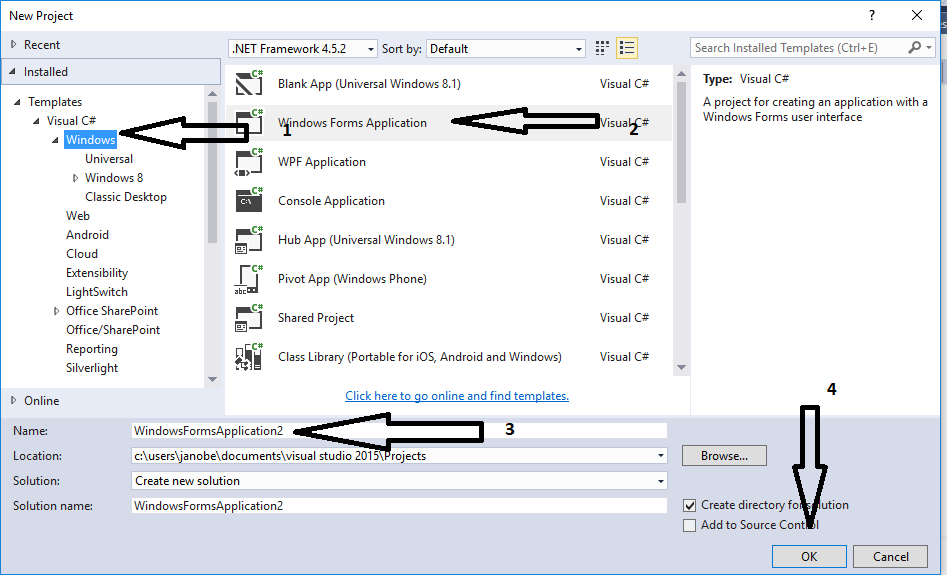
Step 2
Add two RichTextBoxes, Label and a Button inside the Form just like shown below.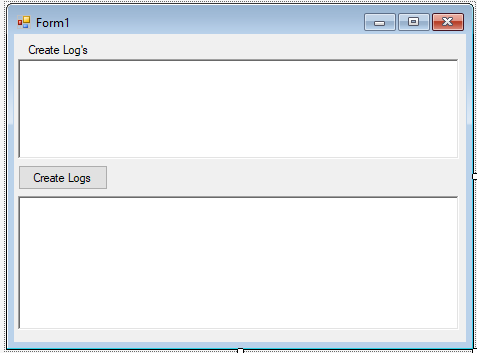
Step 3
Press F7 to open the code editor. In the code editor, create a method for creating a Log File.- public static void Write_Log(string logMSG)
- {
- string string_Path = Application.StartupPath + "\\" + System.DateTime.Today.ToString("MM-dd-yyyy") + "." + "txt";
- if (!dir_info.Exists) dir_info.Create();
- {
- {
- log.WriteLine(logMSG);
- }
- }
- }s
Step 4
Create a method for retrieving a Log File.- public static void show_Logs(RichTextBox txt)
- {
- string strPath = Application.StartupPath + "\\" + System.DateTime.Today.ToString("MM-dd-yyyy") + "." + "txt";
- if (File.Exists(strPath))
- {
- txt.Text = sReader.ReadToEnd();
- sReader.Close();
- }
- else
- {
- MessageBox.Show("File not exist.", "error", MessageBoxButtons.OK, MessageBoxIcon.Error);
- }
- }
Step 5
Add the following code to retrieve a Log File and display it in the RichTextBox in the first load of the form.- private void Form1_Load(object sender, EventArgs e)
- {
- show_Logs(richTextBox2);
- }
- Write_Log(richTextBox1.Text);
- richTextBox1.Clear();
- show_Logs(richTextBox2);