User Navigation in C# - (Next and Previous Buttons)
Submitted by janobe on Friday, May 24, 2019 - 17:06.
This time, I will teach you how to navigate user details using C# and MySQL Database. I create a simple navigation method that can be easily learned by a newbie in programming. It has a “Next” and “Previous” button that can navigate multiple records back and forth, all you have to do is follow the procedure below.
The complete source code is included. You can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Database
Create a database named "db_user". Write the following codes for creating and adding data in the table.- --
- -- Dumping data for table `tbl_user`
- --
- (1, 'Janno Palacios', 'janobe', 'admin', 'Administrator'),
- (2, 'Jeanniebe Nillos', 'jean', 'janobe', 'Staff');
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.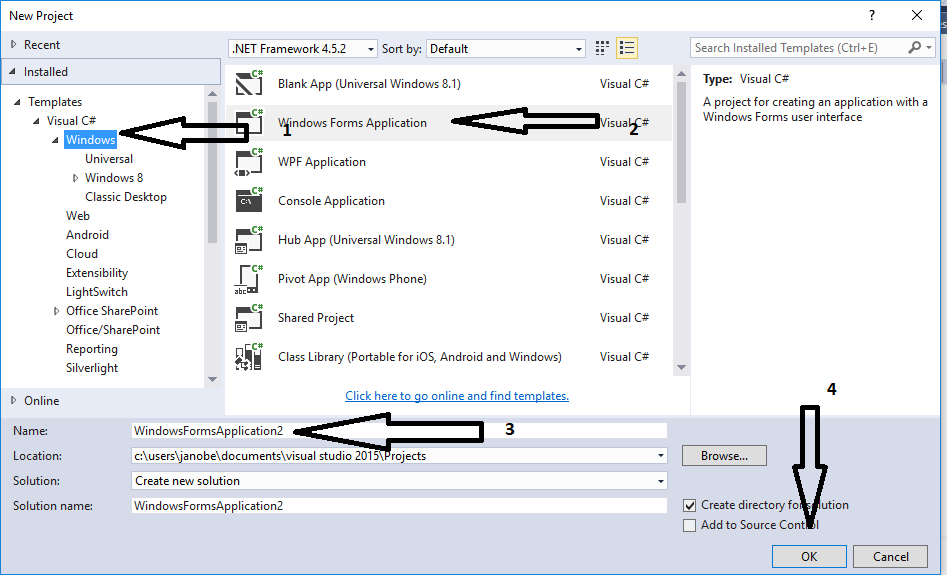
Step 2
Do the form just like shown below.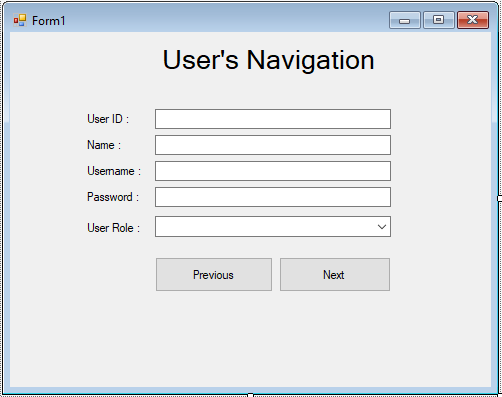
Step 3
Press F7 to open the code editor. In the code editor, add a namespace to access MySQL libraries. Note : AddMySQL.Data.dll
as your references. Visit this tutorial to know how to add MySQL.Data.dll
- using MySql.Data.MySqlClient;
Step 4
Declare all the classes and variables that are needed.- MySqlConnection con = new MySqlConnection("server=localhost;user id=root;password=;database=db_user;sslMode=none");
- MySqlDataAdapter da;
- MySqlCommand cmd;
- DataTable dt;
- string sql;
- int maxrow, inc;s
Step 5
Create a method to navigate the records.- private void navigate_records(string sql, int inc)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- maxrow = dt.Rows.Count - 1;
- txtUserID.Text = dt.Rows[inc].Field<int>(0).ToString();
- txtName.Text = dt.Rows[inc].Field<string>(1);
- txtUsername.Text = dt.Rows[inc].Field<string>(2);
- txtPass.Text = dt.Rows[inc].Field<string>(3);
- cboRole.Text = dt.Rows[inc].Field<string>(4);
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message, "Exception Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
- }
- finally
- {
- con.Close();
- da.Dispose();
- }
- }
Step 6
Write the following code to retrieve the first record in a specific field in the first load of the form.- private void Form1_Load(object sender, EventArgs e)
- {
- inc = 0;
- sql = "SELECT * FROM `tbl_user`";
- navigate_records(sql, inc);
- }
Step 7
Write this code for the next record.- private void btnNex_Click(object sender, EventArgs e)
- {
- if(inc != maxrow)
- {
- inc = inc + 1;
- sql = "SELECT * FROM `tbl_user`";
- navigate_records(sql, inc);
- }
- else
- {
- MessageBox.Show("No more rows.", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
- }
- }
Step 8
Write this code for the previous record.- private void btnPrev_Click(object sender, EventArgs e)
- {
- if (inc > 0)
- {
- inc = inc - 1;
- sql = "SELECT * FROM `tbl_user`";
- navigate_records(sql, inc);
- }
- else if(inc == 0)
- {
- MessageBox.Show("First Records", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
- }
- }