ColorDialog in C#
Submitted by janobe on Wednesday, May 22, 2019 - 17:48.
In this tutorial, I will teach you how to use a ColorDialog in C#. This method has the ability to select a color in a textbox. It shows a form with different colors that you can select to change your text and background color. See the procedure below to see how it works.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.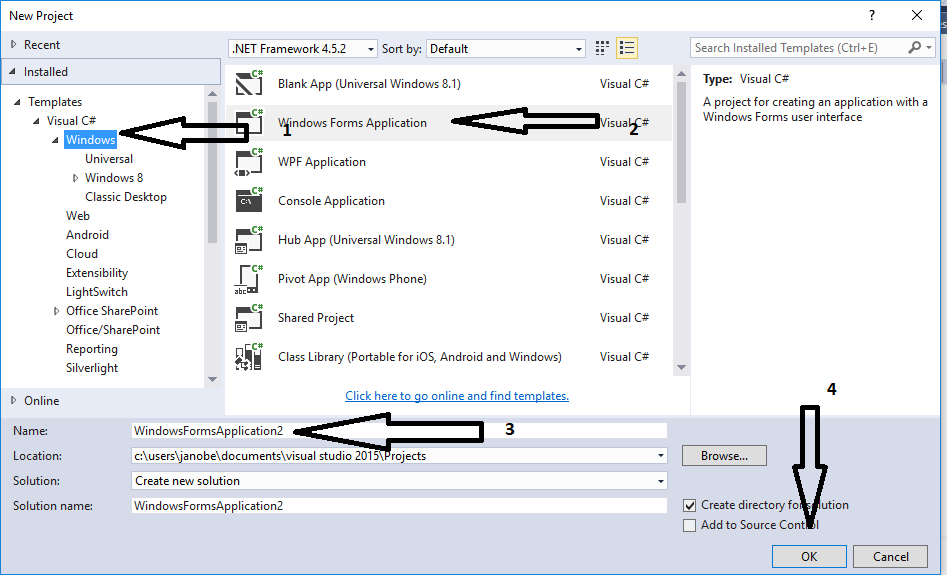
Step 2
Add a Textbox, button and a colordialog into the form. Do the form just like shown below.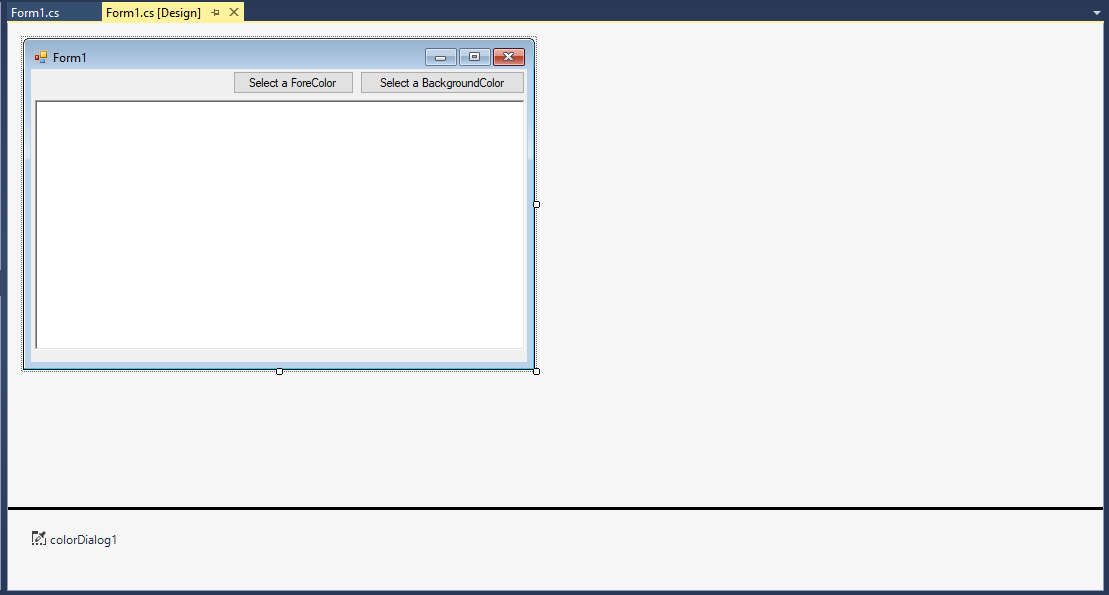
Step 3
Press F7 to open the code editor. In the code editor, create a method for changing the colors of your text and background inside the textbox.- private void change_forecolor()
- {
- // 'SET THE COLORS THAT YOU HAVE SELECTED TO MATCH
- // 'THE CURRENTLY COLORS THAT WAS USED IN THE RICHTEXTBOX.
- colorDialog1.Color = richTextBox1.ForeColor;
- // 'IT ALLOWS YOU TO CREATE CUSTOM COLORS.
- colorDialog1.AllowFullOpen = true;
- // 'THE BASIC COLORS WILL DISPLAY.
- colorDialog1.AnyColor = true;
- // 'THE DIALOG BOX WILL DISPLAY WITH THE CUSTOM COLOR SETTINGS, SIDE OPEN, AS WELL.
- colorDialog1.FullOpen = false;
- // 'SOLID COLORS ARE ALLOWED.
- colorDialog1.SolidColorOnly = true;
- if (colorDialog1.ShowDialog() == DialogResult.OK)
- {
- richTextBox1.ForeColor = colorDialog1.Color;
- }
- //'RESET ALL THE COLORS IN THE DIALOG BOX.
- colorDialog1.Reset();
- }
- private void background_color()
- {
- // 'SET THE COLORS THAT YOU HAVE SELECTED TO MATCH
- // 'THE CURRENTLY COLORS THAT WAS USED IN THE RICHTEXTBOX.
- colorDialog1.Color = richTextBox1.BackColor;
- // 'IT ALLOWS YOU TO CREATE CUSTOM COLORS.
- colorDialog1.AllowFullOpen = true;
- // 'THE BASIC COLORS WILL DISPLAY.
- colorDialog1.AnyColor = true;
- // 'THE DIALOG BOX WILL DISPLAY WITH THE CUSTOM COLOR SETTINGS, SIDE OPEN, AS WELL.
- colorDialog1.FullOpen = false;
- // 'SOLID COLORS ARE ALLOWED.
- colorDialog1.SolidColorOnly = true;
- if (colorDialog1.ShowDialog() == DialogResult.OK)
- {
- richTextBox1.BackColor = colorDialog1.Color;
- }
- // 'RESET ALL THE COLORS IN THE DIALOG BOX.
- colorDialog1.Reset();
- }
Step 4
Write the following code to execute the method that you have created when the button is clicked.- private void button1_Click(object sender, EventArgs e)
- {
- change_forecolor();
- }
- private void button2_Click(object sender, EventArgs e)
- {
- background_color();
- }