C# - Simple CRUD Application With SQLite
Submitted by razormist on Thursday, April 19, 2018 - 21:19.
In this tutorial we will create a Simple CRUD Application With SQLite using C#. C# is a general-purpose, object-oriented programming language. C# is expected to make it faster and less expensive to develop new application. The potential of C# is great when it comes in developing desktop application. It has a friendly environment for all new developers. So let's do the coding...
Then go to the browse and search sqlite, after that install it and wait until the process is completed.
Next go to the Updates and update the needed framework to make sqlite work properly.
Note: Update only the framework if there is an available new update.
or also you create the layout by dragging the proper tools to the forms.
Try to run the application and see if it works.
There you go we successfully created a Simple CRUD Application With SQLite using C#. I hope that this tutorial help you understand on how to develop an application using C#. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!
Getting Started
First you will have to download & install the Visual Studio. Visual Studios is an open source development feel free to create any application that you want. Here's the link for the Visual Studio https://www.visualstudio.com/. Here's the link for the SQLite Browser http://sqlitebrowser.org/.Setting up SQLite
SQLite is very carefully tested prior to every release and relevant to use in some way. SQLite is very usable in any environments especially in embedded devices. First all you need to do is to install the components of the SQLIte database, by right clicking in the Main project title in the solution explorer then selecting the Manage NuGet Packages.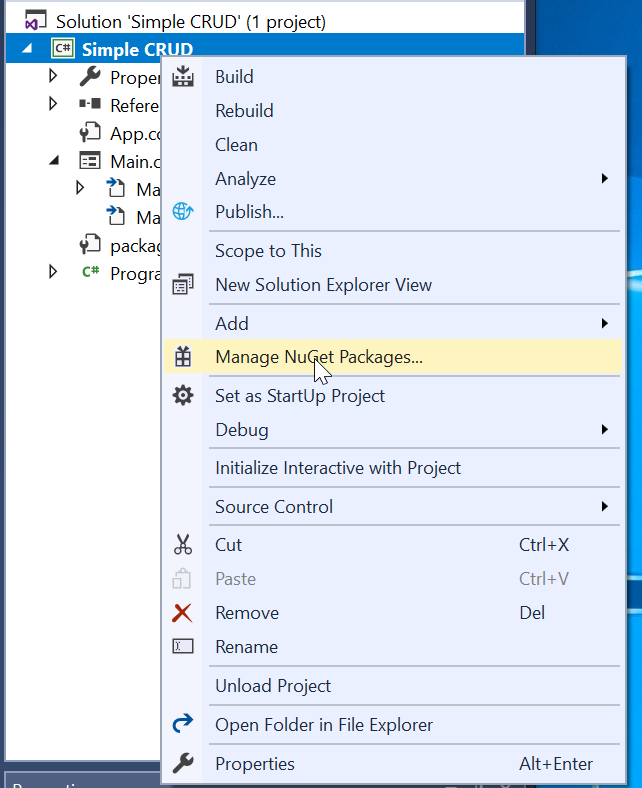
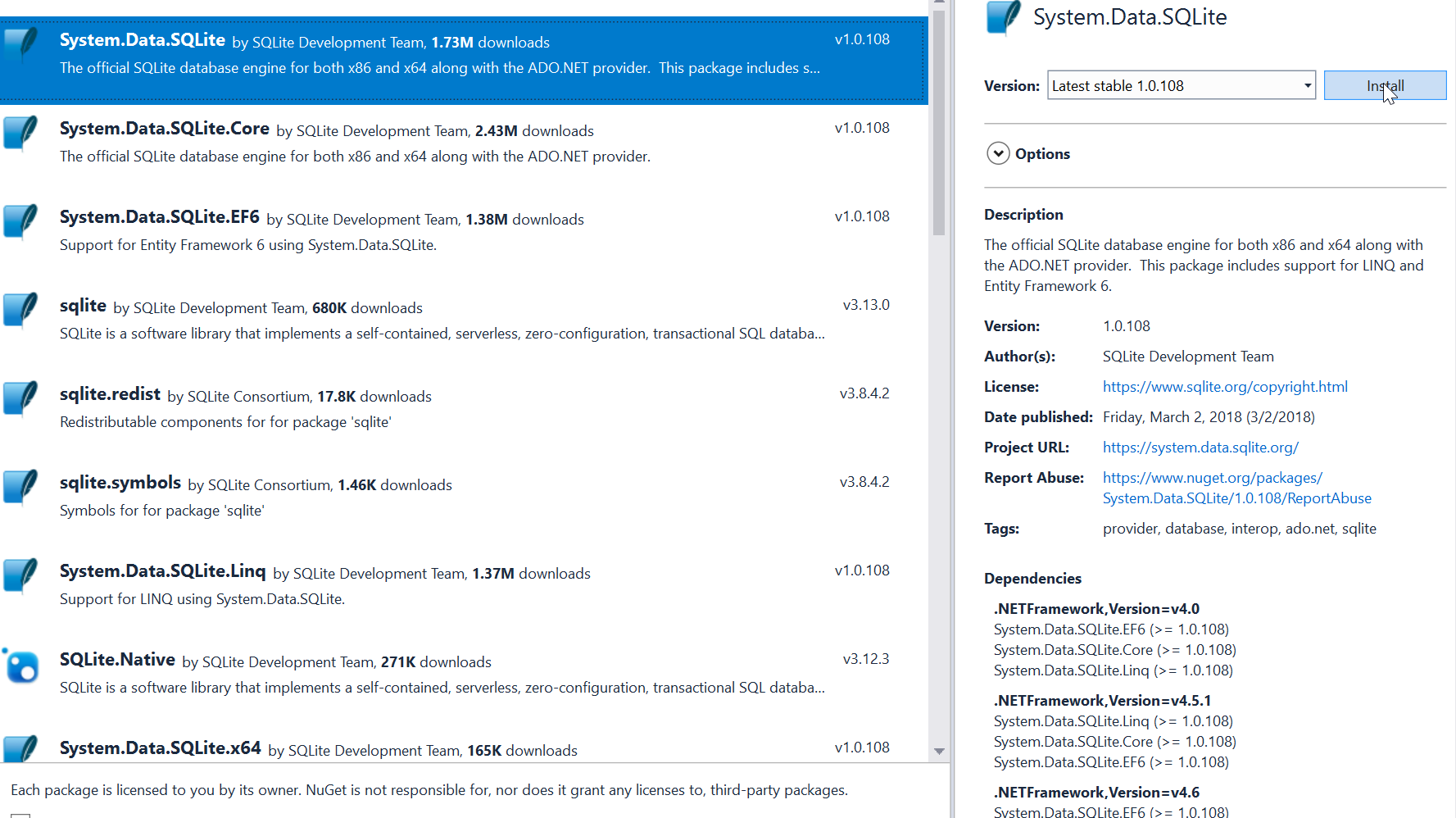
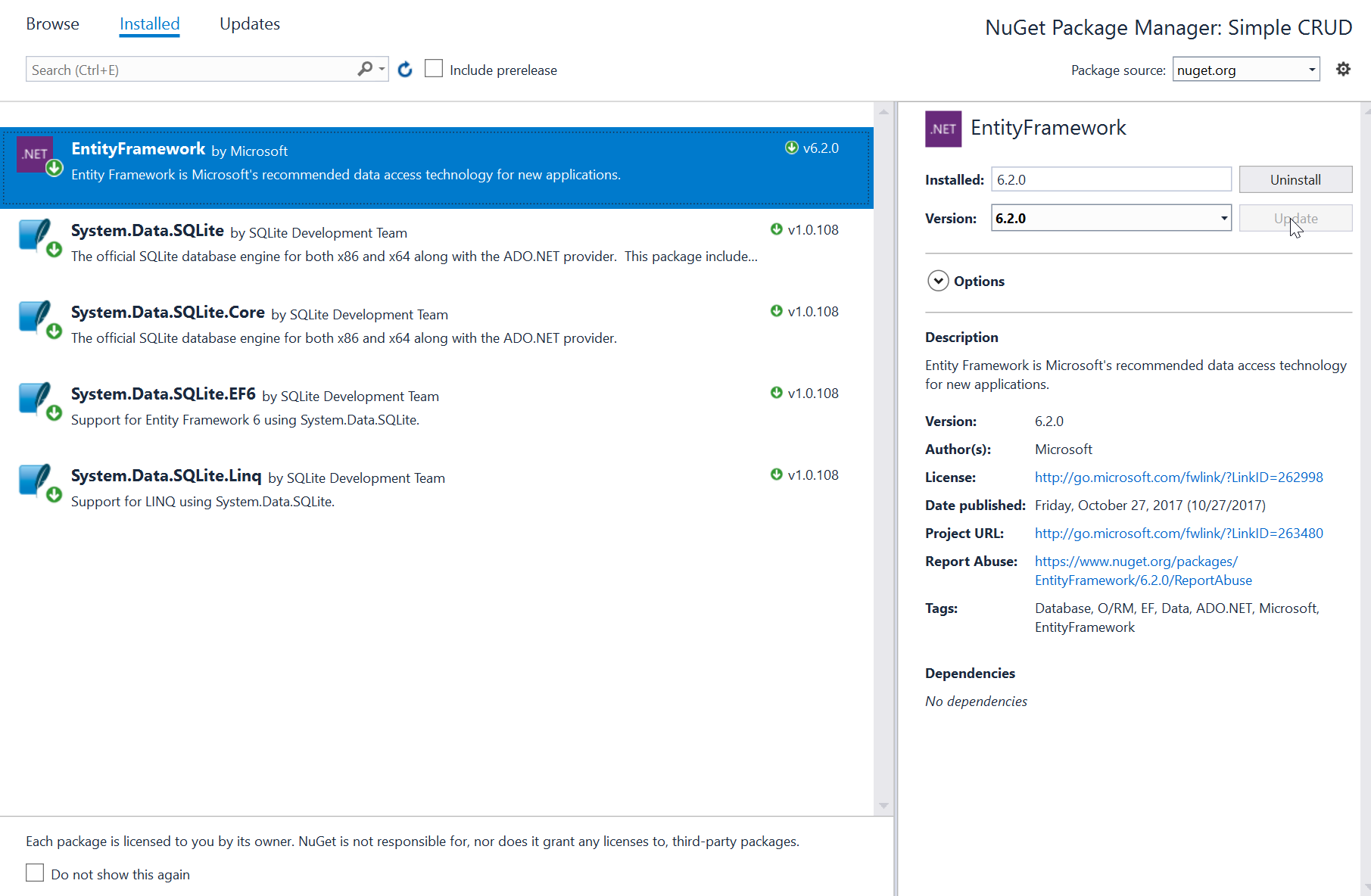
Application Design
We will now create the design for the application, first locate the designer file called form1.Designer.cs, this is the default name when you create a new windows form. Rename the form as Main.cs and then write these codes inside your designer file.- namespace Simple_CRUD
- {
- partial class Main
- {
- /// <summary>
- /// Required designer variable.
- /// </summary>
- private System.ComponentModel.IContainer components = null;
- /// <summary>
- /// Clean up any resources being used.
- /// </summary>
- /// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
- protected override void Dispose(bool disposing)
- {
- if (disposing && (components != null))
- {
- components.Dispose();
- }
- base.Dispose(disposing);
- }
- #region Windows Form Designer generated code
- /// <summary>
- /// Required method for Designer support - do not modify
- /// the contents of this method with the code editor.
- /// </summary>
- private void InitializeComponent()
- {
- ((System.ComponentModel.ISupportInitialize)(this.dataGridView1)).BeginInit();
- this.SuspendLayout();
- //
- // btn_add
- //
- this.btn_add.Name = "btn_add";
- this.btn_add.TabIndex = 0;
- this.btn_add.Text = "ADD";
- this.btn_add.UseVisualStyleBackColor = true;
- //
- // dataGridView1
- //
- this.dataGridView1.AllowUserToAddRows = false;
- this.dataGridView1.AllowUserToDeleteRows = false;
- this.dataGridView1.ColumnHeadersHeightSizeMode = System.Windows.Forms.DataGridViewColumnHeadersHeightSizeMode.AutoSize;
- this.dataGridView1.Name = "dataGridView1";
- this.dataGridView1.ReadOnly = true;
- this.dataGridView1.RowTemplate.Height = 28;
- this.dataGridView1.TabIndex = 7;
- this.dataGridView1.CellClick += new System.Windows.Forms.DataGridViewCellEventHandler(this.GetIdToDelete);
- this.dataGridView1.CellDoubleClick += new System.Windows.Forms.DataGridViewCellEventHandler(this.Edit);
- //
- // label1
- //
- this.label1.AutoSize = true;
- this.label1.Font = new System.Drawing.Font("Arial", 12F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
- this.label1.Name = "label1";
- this.label1.TabIndex = 8;
- this.label1.Text = "Firstname";
- //
- // txt_firstname
- //
- this.txt_firstname.Font = new System.Drawing.Font("Arial", 12F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
- this.txt_firstname.Name = "txt_firstname";
- this.txt_firstname.TabIndex = 9;
- //
- // txt_lastname
- //
- this.txt_lastname.Font = new System.Drawing.Font("Arial", 12F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
- this.txt_lastname.Name = "txt_lastname";
- this.txt_lastname.TabIndex = 11;
- //
- // label2
- //
- this.label2.AutoSize = true;
- this.label2.Font = new System.Drawing.Font("Arial", 12F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
- this.label2.Name = "label2";
- this.label2.TabIndex = 10;
- this.label2.Text = "Lastname";
- //
- // txt_address
- //
- this.txt_address.Font = new System.Drawing.Font("Arial", 12F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
- this.txt_address.Name = "txt_address";
- this.txt_address.TabIndex = 13;
- //
- // label3
- //
- this.label3.AutoSize = true;
- this.label3.Font = new System.Drawing.Font("Arial", 12F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
- this.label3.Name = "label3";
- this.label3.TabIndex = 12;
- this.label3.Text = "Address";
- //
- // btn_update
- //
- this.btn_update.Name = "btn_update";
- this.btn_update.TabIndex = 14;
- this.btn_update.Text = "UPDATE";
- this.btn_update.UseVisualStyleBackColor = true;
- //
- // btn_delete
- //
- this.btn_delete.Name = "btn_delete";
- this.btn_delete.TabIndex = 15;
- this.btn_delete.Text = "DELETE";
- this.btn_delete.UseVisualStyleBackColor = true;
- //
- // btn_clear
- //
- this.btn_clear.Name = "btn_clear";
- this.btn_clear.TabIndex = 16;
- this.btn_clear.Text = "CLEAR";
- this.btn_clear.UseVisualStyleBackColor = true;
- //
- // Main
- //
- this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
- this.Controls.Add(this.btn_clear);
- this.Controls.Add(this.btn_delete);
- this.Controls.Add(this.btn_update);
- this.Controls.Add(this.txt_address);
- this.Controls.Add(this.label3);
- this.Controls.Add(this.txt_lastname);
- this.Controls.Add(this.label2);
- this.Controls.Add(this.txt_firstname);
- this.Controls.Add(this.label1);
- this.Controls.Add(this.dataGridView1);
- this.Controls.Add(this.btn_add);
- this.Name = "Main";
- this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
- this.Text = "Main";
- ((System.ComponentModel.ISupportInitialize)(this.dataGridView1)).EndInit();
- this.ResumeLayout(false);
- this.PerformLayout();
- }
- #endregion
- private System.Windows.Forms.Button btn_add;
- private System.Windows.Forms.DataGridView dataGridView1;
- private System.Windows.Forms.Label label1;
- private System.Windows.Forms.TextBox txt_firstname;
- private System.Windows.Forms.TextBox txt_lastname;
- private System.Windows.Forms.Label label2;
- private System.Windows.Forms.TextBox txt_address;
- private System.Windows.Forms.Label label3;
- private System.Windows.Forms.Button btn_update;
- private System.Windows.Forms.Button btn_delete;
- private System.Windows.Forms.Button btn_clear;
- }
- }
Creating the Script
We will now create the script to make things work. To do that go to the csharp script called Main.cs then right click and select view code, this will force you to go to the text editor. Then write these block of codes inside the Class of the form.- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.IO;
- using System.Drawing;
- using System.Linq;
- using System.Text;
- using System.Threading.Tasks;
- using System.Windows.Forms;
- using System.Data.SQLite;
- namespace Simple_CRUD
- {
- public partial class Main : Form
- {
- SQLiteConnection conn;
- SQLiteCommand cmd;
- SQLiteDataAdapter adapter;
- int id;
- bool isDoubleClick = false;
- String connectString;
- public Main()
- {
- InitializeComponent();
- connectString = @"Data Source=" + Application.StartupPath + @"\Database\crud.db;version=3";
- GenerateDatabase();
- }
- private void Add(object sender, EventArgs e) {
- if (txt_firstname.Text != "" || txt_lastname.Text != "" || txt_address.Text != "")
- {
- try
- {
- cmd.CommandText = @"INSERT INTO member (firstname, lastname, address) VALUES(@firstname, @lastname, @address)";
- cmd.Connection = conn;
- conn.Open();
- int i = cmd.ExecuteNonQuery();
- if (i == 1)
- {
- MessageBox.Show("Successfully Created!");
- txt_firstname.Text = "";
- txt_lastname.Text = "";
- txt_address.Text = "";
- ReadData();
- }
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- }
- else {
- MessageBox.Show("Required Field!");
- }
- }
- private void GenerateDatabase() {
- String path = Application.StartupPath + @"\Database\crud.db";
- if (!File.Exists(path))
- {
- conn.Open();
- string sql = "CREATE TABLE member (ID INTEGER PRIMARY KEY AUTOINCREMENT, firstname TEXT, lastname TEXT, address TEXT)";
- cmd.ExecuteNonQuery();
- conn.Close();
- }
- }
- private void ReadData() {
- try
- {
- conn.Open();
- String sql = "SELECT * FROM member";
- ds.Reset();
- adapter.Fill(ds);
- dt = ds.Tables[0];
- dataGridView1.DataSource = dt;
- conn.Close();
- dataGridView1.Columns[1].HeaderText = "Firstname";
- dataGridView1.Columns[2].HeaderText = "Lastname";
- dataGridView1.Columns[3].HeaderText = "Address";
- dataGridView1.Columns[0].Visible = false;
- dataGridView1.Columns[1].AutoSizeMode = DataGridViewAutoSizeColumnMode.AllCells;
- dataGridView1.Columns[2].AutoSizeMode = DataGridViewAutoSizeColumnMode.AllCells;
- dataGridView1.Columns[3].AutoSizeMode = DataGridViewAutoSizeColumnMode.Fill;
- dataGridView1.SelectionMode = DataGridViewSelectionMode.FullRowSelect;
- }
- catch (Exception ex) {
- MessageBox.Show(ex.Message);
- }
- }
- private void Main_Load(object sender, EventArgs e)
- {
- ReadData();
- }
- private void Edit(object sender, DataGridViewCellEventArgs e) {
- id = Convert.ToInt32(dataGridView1.SelectedRows[0].Cells[0].Value);
- txt_firstname.Text = dataGridView1.SelectedRows[0].Cells[1].Value.ToString();
- txt_lastname.Text = dataGridView1.SelectedRows[0].Cells[2].Value.ToString();
- txt_address.Text = dataGridView1.SelectedRows[0].Cells[3].Value.ToString();
- isDoubleClick = true;
- }
- private void GetIdToDelete(object sender, DataGridViewCellEventArgs e) {
- id = Convert.ToInt32(dataGridView1.SelectedRows[0].Cells[0].Value);
- isDoubleClick = false;
- txt_firstname.Text = "";
- txt_lastname.Text = "";
- txt_address.Text = "";
- }
- private void Update(object sender, EventArgs e) {
- if(isDoubleClick) {
- try {
- conn.Open();
- cmd.CommandText = @"UPDATE member set firstname=@firstname, lastname=@lastname, address=@address WHERE ID='"+ id +"'";
- cmd.Connection = conn;
- cmd.Parameters.AddWithValue("@firstname", txt_firstname.Text);
- cmd.Parameters.AddWithValue("@lastname", txt_lastname.Text);
- cmd.Parameters.AddWithValue("@address", txt_address.Text);
- int i = cmd.ExecuteNonQuery();
- if (i == 1)
- {
- MessageBox.Show("Successfully Updated!");
- txt_firstname.Text = "";
- txt_lastname.Text = "";
- txt_address.Text = "";
- ReadData();
- id = 0;
- dataGridView1.ClearSelection();
- dataGridView1.CurrentCell = null;
- isDoubleClick = false;
- }
- conn.Close();
- }
- catch (Exception ex) {
- MessageBox.Show(ex.Message);
- }
- }
- }
- private void Delete(object sender, EventArgs e) {
- DialogResult dialogResult = MessageBox.Show("Do you to delete this record?", "Warning", MessageBoxButtons.YesNo, MessageBoxIcon.Warning);
- if (dialogResult == DialogResult.Yes)
- {
- try
- {
- conn.Open();
- cmd.CommandText = @"DELETE FROM member WHERE ID='" + id + "'";
- cmd.Connection = conn;
- int i = cmd.ExecuteNonQuery();
- if (i == 1)
- {
- MessageBox.Show("Successfully Deleted!");
- id = 0;
- dataGridView1.ClearSelection();
- dataGridView1.CurrentCell = null;
- ReadData();
- dataGridView1.ClearSelection();
- dataGridView1.CurrentCell = null;
- }
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- }
- else if (dialogResult == DialogResult.No)
- {
- }
- }
- private void Clear(object sender, EventArgs e)
- {
- id = 0;
- txt_firstname.Text = "";
- txt_lastname.Text = "";
- txt_address.Text = "";
- dataGridView1.ClearSelection();
- dataGridView1.CurrentCell = null;
- isDoubleClick = false;
- }
- }
- }