Retrieving and Deleting Data in the Database Using C# and SQL Server 2005
Submitted by janobe on Wednesday, July 27, 2016 - 10:20.
In this tutorial, I will show you how to retrieve and delete the data in the database using C#.net and SQL server 2005. This method has the ability to display the records that has been saved in the database to the Datagridview. It will also delete the existing records in the table of the database. Follow the step by step guide below.
Step 3. Go to the Solution Explorer, click the “View Code” to fire the code editor.
Step 4. Declare all the classes and variables that are needed.
Step 6. Go back to the design view, double-click the Form and do the following codes for establishing the connection between C# and SQL server.
Step 7. Go back to the design view again, double-click a button and do the following codes for deleting Employee's Records in the SQL database.
For all students who need a programmer for your thesis system or anyone who needs a source code in any programming languages. You can contact me @ :
Email – [email protected]
Mobile No. – 09305235027 – TNT
So, let’s get started:
Step 1. Create a database and name it “testdb”. Step 2. Open Microsoft Visual Studio 2008 and create new Windows Form Application. Then, do the Form as follows.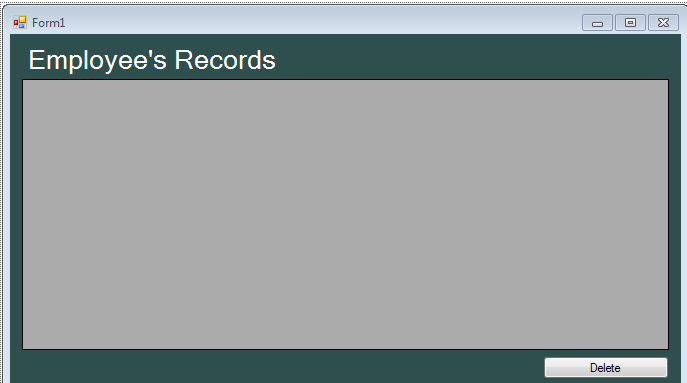
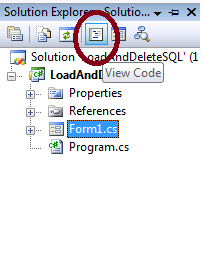
Note: Put "using System.Data.SqlClient;" above the namespace to access sql server library.
Step 5. Create a method for displaying records from the database to the DataGridView.
- private void LoadRecords()
- {
- try
- {
- strquery = "SELECT ID, Firstname, Lastname,Address, ContactNo,EmailAdd FROM tbltest";
- sql_cmd.Connection = strcon;
- sql_cmd.CommandText = strquery;
- sql_da.SelectCommand = sql_cmd;
- sql_da.Fill(dt);
- dataGridView1.DataSource = dt;
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- sql_da.Dispose();
- }
- }
- //establish a connection between sql server 2005 express edition to c#.net
- strcon.ConnectionString = "Data Source=.\\SQLEXPRESS;Database=testdb;trusted_connection=true;";
- //call a method to retrieve it on the first loa of the form
- LoadRecords();
- try
- {
- strcon.Open();
- strquery = "DELETE FROM tbltest WHERE ID=" + dataGridView1.CurrentRow .Cells[0].FormattedValue ;
- sql_cmd.Connection = strcon;
- sql_cmd.CommandText = strquery;
- int result = sql_cmd.ExecuteNonQuery();
- if (res > 0)
- {
- MessageBox.Show("Data has been deleted in the SQL database");
- LoadRecords ();
- }
- else
- {
- MessageBox.Show("SQL QUERY ERROR");
- }
- strcon.Close();
- }
- catch (Exception ex)//catch exeption
- {
- MessageBox.Show(ex.Message);
- }