Creating a Login Form with OTP Verification through Email in PHP Tutorial
In this tutorial, I will show how to create a simple 2 Factor Authentication when logging in using PHP Language. This will help you to create a more secure authentication for your system users in your current or future PHP Projects. The system will generate an OTP or the One-Time-PIN every time the users attempt to login into the system. Here, the OTP will be through an email using GMail SMTP.
The application that we'll be crating in this tutorial has simple functionalities such registration, login, verification, home page, and logout. I hope this will help you.
Getting Started
Download and Install XAMPP as your virtual server in your local machine. Also, download Bootstrap and jQuery for the design of the application that we will be creating.
Setting-Up the XAMPP to send Mail
First, locate your XAMPP's php.ini file and open it with your favorite text editor such as notepad++ or sublime text. Next, in your text editor find the mail configuration by searching it using "[mail function]". Then, follow the configuration shown in the image below.
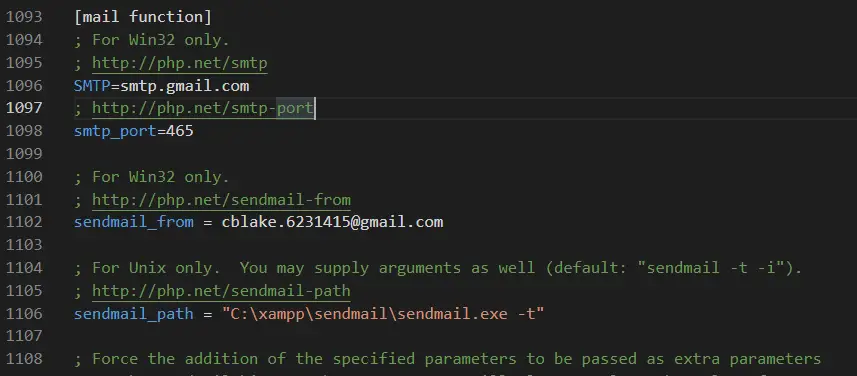
Next, setup the XAMPP's sendmail configuration. Locate and open the sendmail.ini file in your text editor. Then, follow the configuration shown in the image below.
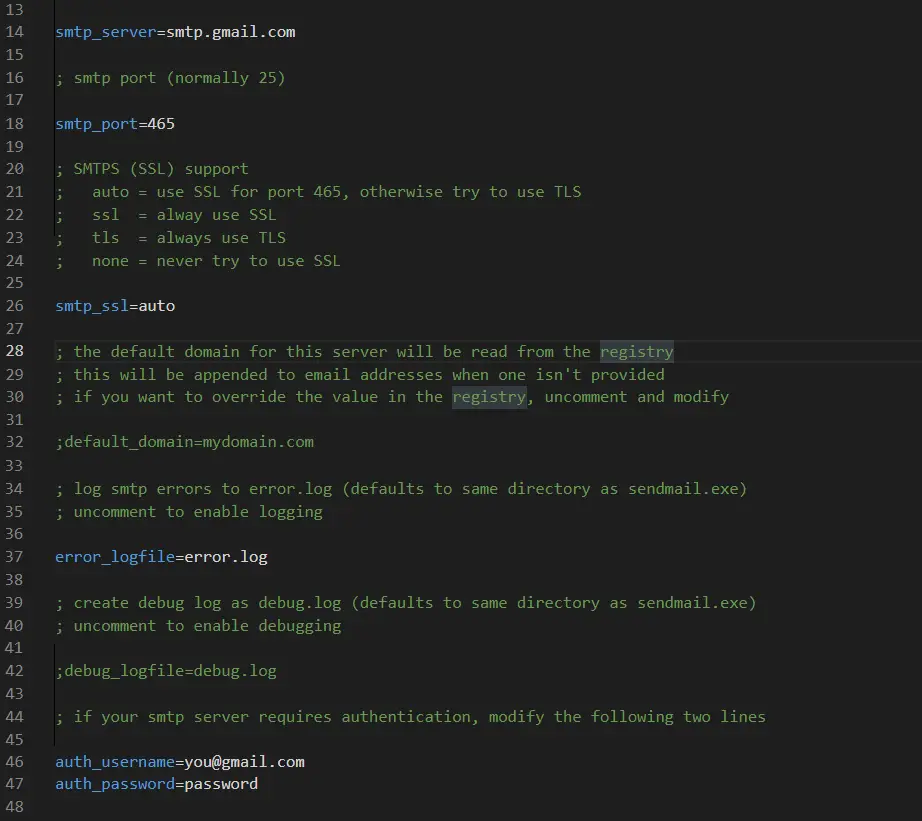
Allowing Less Secure Apps
Next, allow your gmail to be access by less secure apps so you can send mail locally using the app that we'll be creating. Follow the steps below.
Step 1
Open you google account's settings.
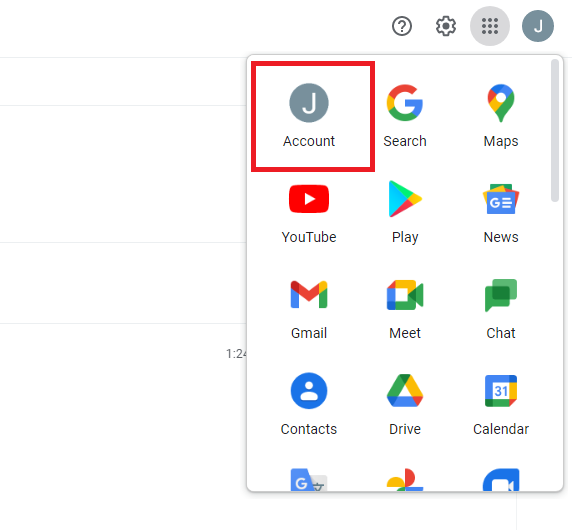
Step 2
Navigate the page into the 'security' and click the link on turning on less secure apps.
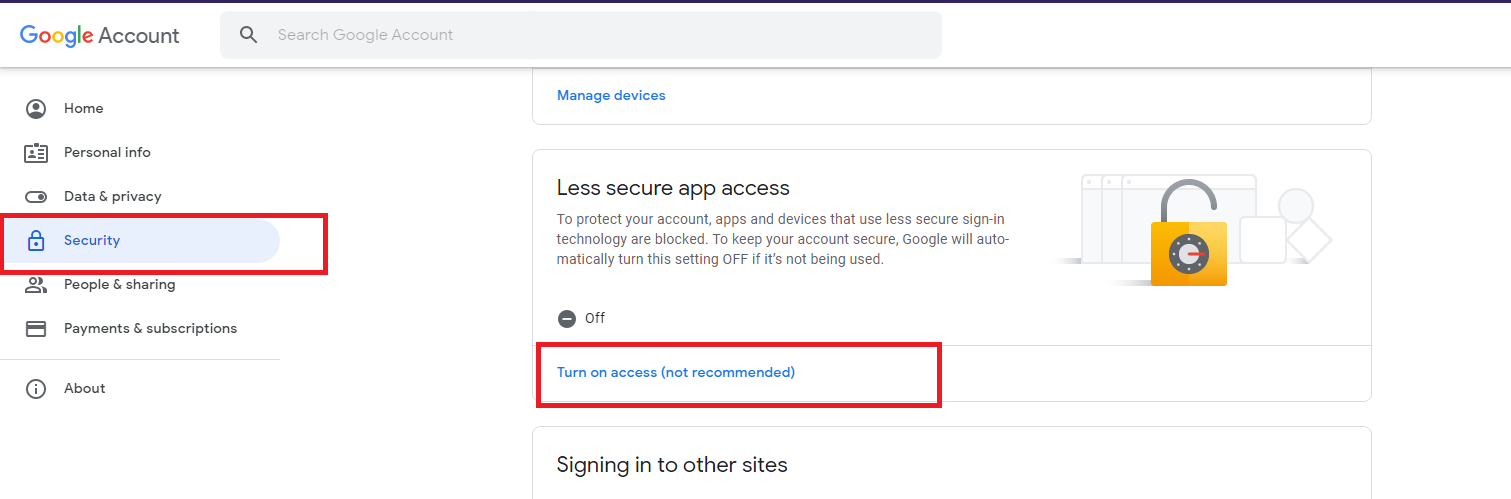
Step 3
Toggle to allow less secure apps.
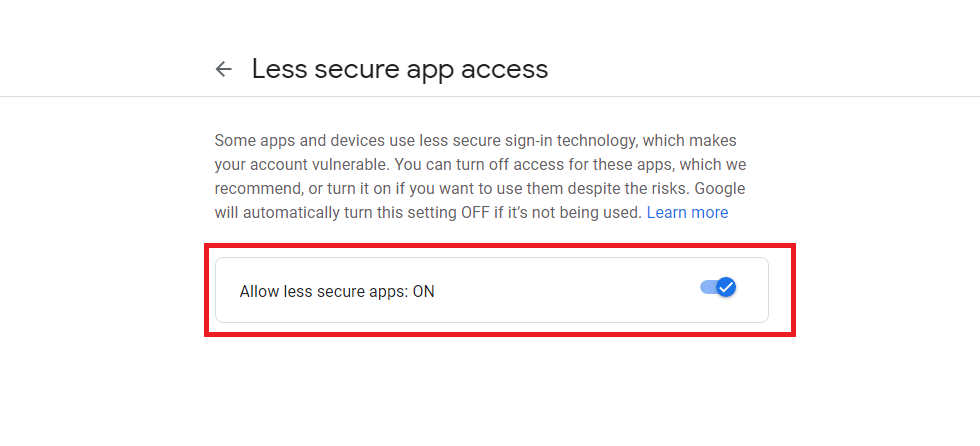
Creating the Database
In your browser, browse the XAMPP's PHPMyAdmin [http://localhost/phpmyadmin] and create a new database naming login_otp_db. Next, navigate the page into the SQL Tab and copy/paste the mysql script below in the provided text field in the page. Then, click the 'GO'button.
Creating the PHP Main Class
The application that we are creating will be using an OOP Approach in writing the back-end scripts of the app. The class includes the database configuration and send mail function. Save the code file below as MainClass.php.
- <?php
- if(session_status() === PHP_SESSION_NONE)
- Class MainClass{
- protected $db;
- function __construct(){
- $this->db = new mysqli('localhost','root','','login_otp_db');
- if(!$this->db){
- }
- }
- function db_connect(){
- return $this->db;
- }
- public function register(){
- foreach($_POST as $k => $v){
- $$k = $this->db->real_escape_string($v);
- }
- $password = password_hash($password, PASSWORD_DEFAULT);
- $check = $this->db->query("SELECT * FROM `users` where `email`= '$email}' ")->num_rows;
- if($check > 0){
- $resp['status'] = 'failed';
- $_SESSION['flashdata']['type']='danger';
- $_SESSION['flashdata']['msg'] = ' Email already exists.';
- }else{
- $sql = "INSERT INTO `users` (firstname,middlename,lastname,email,`password`) VALUES ('$firstname','$middlename','$lastname','$email','$password')";
- $save = $this->db->query($sql);
- if($save){
- $resp['status'] = 'success';
- }else{
- $resp['status'] = 'failed';
- $resp['err'] = $this->db->error;
- $_SESSION['flashdata']['type']='danger';
- $_SESSION['flashdata']['msg'] = ' An error occurred.';
- }
- }
- }
- public function login(){
- $sql = "SELECT * FROM `users` where `email` = ? ";
- $stmt = $this->db->prepare($sql);
- $stmt->bind_param('s',$email);
- $stmt->execute();
- $result = $stmt->get_result();
- if($result->num_rows > 0){
- $data = $result->fetch_array();
- $pass_is_right = password_verify($password,$data['password']);
- $has_code = false;
- $update_sql = "UPDATE `users` set otp_expiration = '{$expiration}', otp = '{$otp}' where id='{$data['id']}' ";
- $update_otp = $this->db->query($update_sql);
- if($update_otp){
- $has_code = true;
- $resp['status'] = 'success';
- $_SESSION['otp_verify_user_id'] = $data['id'];
- $this->send_mail($data['email'],$otp);
- }else{
- $resp['status'] = 'failed';
- $_SESSION['flashdata']['type'] = 'danger';
- $_SESSION['flashdata']['msg'] = ' An error occurred while loggin in. Please try again later.';
- }
- }else if(!$pass_is_right){
- $resp['status'] = 'failed';
- $_SESSION['flashdata']['type'] = 'danger';
- $_SESSION['flashdata']['msg'] = ' Incorrect Password';
- }
- }else{
- $resp['status'] = 'failed';
- $_SESSION['flashdata']['type'] = 'danger';
- $_SESSION['flashdata']['msg'] = ' Email is not registered.';
- }
- }
- public function get_user_data($id){
- $sql = "SELECT * FROM `users` where `id` = ? ";
- $stmt = $this->db->prepare($sql);
- $stmt->bind_param('i',$id);
- $stmt->execute();
- $result = $stmt->get_result();
- $dat=[];
- if($result->num_rows > 0){
- $resp['status'] = 'success';
- foreach($result->fetch_array() as $k => $v){
- $data[$k] = $v;
- }
- }
- $resp['data'] = $data;
- }else{
- $resp['status'] = 'false';
- }
- }
- public function resend_otp($id){
- $update_sql = "UPDATE `users` set otp_expiration = '{$expiration}', otp = '{$otp}' where id = '{$id}' ";
- $update_otp = $this->db->query($update_sql);
- if($update_otp){
- $resp['status'] = 'success';
- $email = $this->db->query("SELECT email FROM `users` where id = '{$id}'")->fetch_array()[0];
- $this->send_mail($email,$otp);
- }else{
- $resp['status'] = 'failed';
- $resp['error'] = $this->db->error;
- }
- }
- public function otp_verify(){
- $sql = "SELECT * FROM `users` where id = ? and otp = ?";
- $stmt = $this->db->prepare($sql);
- $stmt->bind_param('is',$id,$otp);
- $stmt->execute();
- $result = $stmt->get_result();
- if($result->num_rows > 0){
- $resp['status'] = 'success';
- $this->db->query("UPDATE `users` set otp = NULL, otp_expiration = NULL where id = '{$id}'");
- $_SESSION['user_login'] = 1;
- foreach($result->fetch_array() as $k => $v){
- $_SESSION[$k] = $v;
- }
- }else{
- $resp['status'] = 'failed';
- $_SESSION['flashdata']['type'] = 'danger';
- $_SESSION['flashdata']['msg'] = ' Incorrect OTP.';
- }
- }
- function send_mail($to="",$pin=""){
- try{
- $headers = 'From:' .$email . '\r\n'. 'Reply-To:' .
- $email. "\r\n" .
- $headers .= "MIME-Version: 1.0" . "\r\n";
- $headers .= "Content-type:text/html;charset=UTF-8" . "\r\n";
- // the message
- $msg = "
- <html>
- <body>
- <h2>You are Attempting to Login in XYZ Simple PHP Web Application</h2>
- <p>Here is yout OTP (One-Time PIN) to verify your Identity.</p>
- <h3><b>".$pin."</b></h3>
- </body>
- </html>
- ";
- // send email
- // die("ERROR<br>".$headers."<br>".$msg);
- }catch(Exception $e){
- $_SESSION['flashdata']['type']='danger';
- $_SESSION['flashdata']['msg'] = ' An error occurred while sending the OTP. Error: '.$e->getMessage();
- }
- }
- }
- function __destruct(){
- $this->db->close();
- }
- }
- $class = new MainClass();
- $conn= $class->db_connect();
Creating the System Authentication Check Script
The code below is a PHP Script that checks if the user is allowed to access the application page. This will make the user to redirect to the login page if they haven't logged in yet. Save the file as auth.php.
- <?php
- if(session_status() === PHP_SESSION_NONE)
- $link = $_SERVER['PHP_SELF'];
- echo "<script>location.replace('./login.php');</script>";
- }
- echo "<script>location.replace('./login.php');</script>";
- }
- echo "<script>location.replace('./');</script>";
- }
Creating the Interfaces
Next, create the login page of the application. Copy/Paste the code below and configure it the way you want the UI looks. Save the file as login.php.
- <?php
- require_once('auth.php');
- require_once('MainClass.php');
- if($_SERVER['REQUEST_METHOD'] == 'POST'){
- $login = json_decode($class->login());
- if($login->status == 'success'){
- }
- }
- ?>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <meta http-equiv="X-UA-Compatible" content="IE=edge">
- <meta name="viewport" content="width=device-width, initial-scale=1.0">
- <link rel="stylesheet" href="./Font-Awesome-master/css/all.min.css">
- <link rel="stylesheet" href="./css/bootstrap.min.css">
- <style>
- html,body{
- height:100%;
- width:100%;
- }
- main{
- height:calc(100%);
- width:calc(100%);
- display:flex;
- flex-direction:column;
- align-items:center;
- justify-content:center;
- }
- </style>
- </head>
- <body class="bg-dark bg-gradient">
- <main>
- <div class="col-lg-7 col-md-9 col-sm-12 col-xs-12 mb-4">
- </div>
- <div class="col-lg-3 col-md-8 col-sm-12 col-xs-12">
- <div class="card shadow rounded-0">
- <div class="card-header py-1">
- </div>
- <div class="card-body py-4">
- <div class="container-fluid">
- <form action="./login.php" method="POST">
- <?php
- if(isset($_SESSION['flashdata'])):
- ?>
- <div class="dynamic_alert alert alert-<?php echo $_SESSION['flashdata']['type'] ?> my-2 rounded-0">
- <div class="d-flex align-items-center">
- <div class="col-1 text-end">
- </div>
- </div>
- </div>
- </div>
- <?php unset($_SESSION['flashdata']) ?>
- <?php endif; ?>
- <div class="form-group">
- <input type="email" name="email" id="email" class="form-control rounded-0" value="<?= isset($_POST['email']) ? $_POST['email'] : '' ?>" autofocus required>
- </div>
- <div class="form-group">
- <input type="password" name="password" id="password" class="form-control rounded-0" value="<?= isset($_POST['password']) ? $_POST['password'] : '' ?>" required>
- </div>
- <div class="form-group text-end">
- </div>
- <div class="form-group text-cneter">
- </div>
- </form>
- </div>
- </div>
- </div>
- </div>
- </main>
- </body>
- </html>
Output
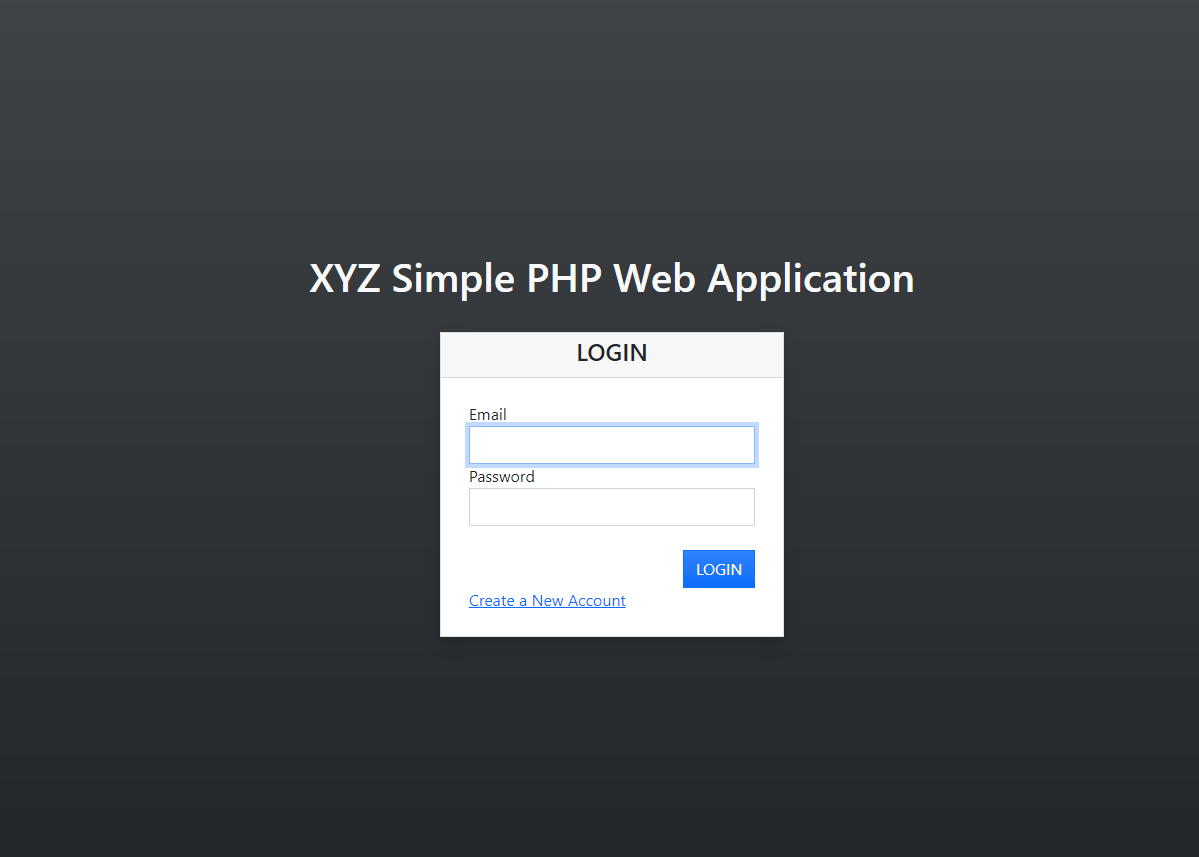
Next, create the registration page of the application. Copy/Paste the code below and configure it the way you want the UI looks. Save the file as registration.php.
- <?php
- require_once('auth.php');
- require_once('MainClass.php');
- if($_SERVER['REQUEST_METHOD'] == 'POST'){
- $register = json_decode($class->register());
- if($register->status == 'success'){
- $_SESSION['flashdata']['type']='success';
- $_SESSION['flashdata']['msg'] = ' Account has been registered successfully.';
- exit;
- }else{
- }
- }
- ?>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <meta http-equiv="X-UA-Compatible" content="IE=edge">
- <meta name="viewport" content="width=device-width, initial-scale=1.0">
- <link rel="stylesheet" href="./Font-Awesome-master/css/all.min.css">
- <link rel="stylesheet" href="./css/bootstrap.min.css">
- <style>
- html,body{
- height:100%;
- width:100%;
- }
- main{
- height:calc(100%);
- width:calc(100%);
- display:flex;
- flex-direction:column;
- align-items:center;
- justify-content:center;
- }
- </style>
- </head>
- <body class="bg-dark bg-gradient">
- <main>
- <div class="col-lg-7 col-md-9 col-sm-12 col-xs-12 mb-4">
- </div>
- <div class="col-lg-3 col-md-8 col-sm-12 col-xs-12">
- <div class="card shadow rounded-0">
- <div class="card-header py-1">
- </div>
- <div class="card-body py-4">
- <div class="container-fluid">
- <form action="./registration.php" method="POST">
- <?php
- if(isset($_SESSION['flashdata'])):
- ?>
- <div class="dynamic_alert alert alert-<?php echo $_SESSION['flashdata']['type'] ?> my-2 rounded-0">
- <div class="d-flex align-items-center">
- <div class="col-1 text-end">
- </div>
- </div>
- </div>
- </div>
- <?php unset($_SESSION['flashdata']) ?>
- <?php endif; ?>
- <div class="form-group">
- <input type="text" name="firstname" id="firstname" class="form-control rounded-0" value="<?= isset($_POST['firstname']) ? $_POST['firstname'] : '' ?>" autofocus required>
- </div>
- <div class="form-group">
- <input type="text" name="middlename" id="middlename" class="form-control rounded-0" value="<?= isset($_POST['middlename']) ? $_POST['middlename'] : '' ?>" required>
- </div>
- <div class="form-group">
- <input type="text" name="lastname" id="lastname" class="form-control rounded-0" value="<?= isset($_POST['lastname']) ? $_POST['lastname'] : '' ?>" required>
- </div>
- <div class="form-group">
- <input type="email" name="email" id="email" class="form-control rounded-0" value="<?= isset($_POST['email']) ? $_POST['email'] : '' ?>" required>
- </div>
- <div class="form-group">
- <input type="password" name="password" id="password" class="form-control rounded-0" value="<?= isset($_POST['password']) ? $_POST['password'] : '' ?>" required>
- </div>
- <div class="form-group text-end">
- </div>
- </form>
- </div>
- </div>
- </div>
- </div>
- </main>
- </body>
- </html>
Output
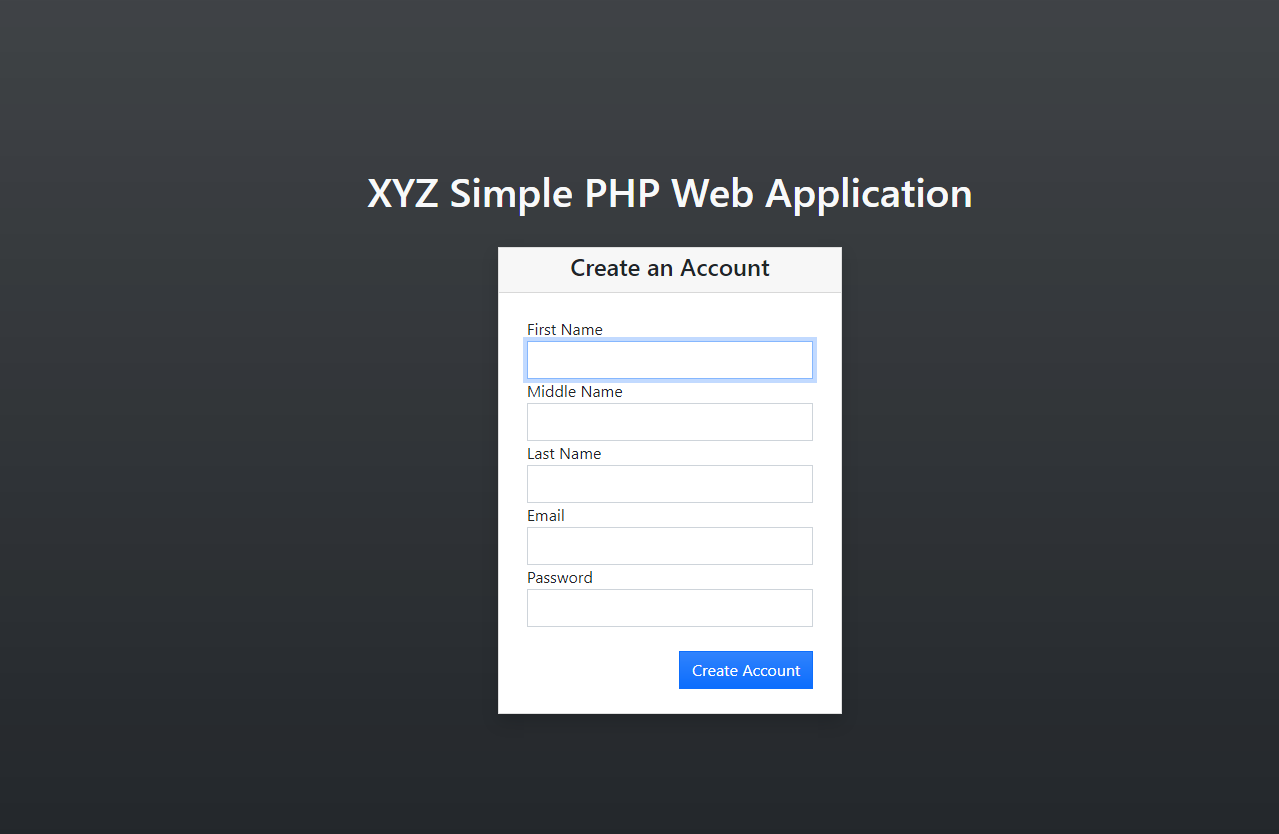
Next, create the verification page of the application. This page will the one where the user will be redirected after successfully login the correct registered user credentials. This is where user will submit the OTP to confirm his/her identity. Copy/Paste the code below and configure it the way you want the UI looks. Save the file as login_verification.php.
- <?php
- require_once('auth.php');
- require_once('MainClass.php');
- $user_data = json_decode($class->get_user_data($_SESSION['otp_verify_user_id']));
- if($user_data->status){
- foreach($user_data->data as $k => $v){
- $$k = $v;
- }
- }
- if(isset($_GET['resend']) && $_GET['resend'] == 'true'){
- $resend = json_decode($class->resend_otp($_SESSION['otp_verify_user_id']));
- if($resend->status == 'success'){
- }else{
- $_SESSION['flashdata']['type']='danger';
- $_SESSION['flashdata']['msg']=' Resending OTP has failed.';
- }
- }
- if($_SERVER['REQUEST_METHOD'] == 'POST'){
- $verify = json_decode($class->otp_verify());
- if($verify->status == 'success'){
- }
- }
- ?>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <meta http-equiv="X-UA-Compatible" content="IE=edge">
- <meta name="viewport" content="width=device-width, initial-scale=1.0">
- <link rel="stylesheet" href="./Font-Awesome-master/css/all.min.css">
- <link rel="stylesheet" href="./css/bootstrap.min.css">
- <style>
- html,body{
- height:100%;
- width:100%;
- }
- main{
- height:calc(100%);
- width:calc(100%);
- display:flex;
- flex-direction:column;
- align-items:center;
- justify-content:center;
- }
- a.disabled {
- pointer-events: none;
- cursor: default;
- }
- </style>
- </head>
- <body class="bg-dark bg-gradient">
- <main>
- <div class="col-lg-7 col-md-9 col-sm-12 col-xs-12 mb-4">
- </div>
- <div class="col-lg-3 col-md-8 col-sm-12 col-xs-12">
- <div class="card shadow rounded-0">
- <div class="card-header py-1">
- </div>
- <div class="card-body py-4">
- <div class="container-fluid">
- <form action="./login_verification.php" method="POST">
- <input type="hidden" name="id" value="<?= isset($id) ? $id : '' ?>">
- <?php
- if(isset($_SESSION['flashdata'])):
- ?>
- <div class="dynamic_alert alert alert-<?php echo $_SESSION['flashdata']['type'] ?> my-2 rounded-0">
- <div class="d-flex align-items-center">
- <div class="col-1 text-end">
- </div>
- </div>
- </div>
- </div>
- <?php unset($_SESSION['flashdata']) ?>
- <?php endif; ?>
- <div class="form-group">
- </div>
- <div class="form-group">
- <input type="otp" name="otp" id="otp" class="form-control rounded-0" value="" maxlength="6" pattern="{0-9}+" autofocus required>
- </div>
- <div class="form-group text-end">
- <a class="btn btn-secondary bg-gradient rounded-0 <?= time() < strtotime($otp_expiration) ? 'disabled' : '' ?>" data-stat="<?= time() < strtotime($otp_expiration) ? 'countdown' : '' ?>" href="./login_verification.php?resend=true" id="resend"><?= time() < strtotime($otp_expiration) ? 'Resend in '.(strtotime($otp_expiration) - time()).'s' : 'Resend OTP' ?></a>
- </div>
- </form>
- </div>
- </div>
- </div>
- </div>
- </main>
- </body>
- <script>
- $(function(){
- var is_countdown_resend = $('#resend').attr('data-stat') == 'countdown';
- if(is_countdown_resend){
- var sec = '<?= time() < strtotime($otp_expiration) ? (strtotime($otp_expiration) - time()) : 0 ?>';
- var countdown = setInterval(() => {
- if(sec > 0){
- sec--;
- $('#resend').text("Resend in "+(sec)+'s')
- }else{
- $('#resend').attr('data-stat','')
- .removeClass('disabled').text('Resend OTP')
- clearInterval(countdown)
- }
- }, 1000);
- }
- })
- </script>
- </html>
Output
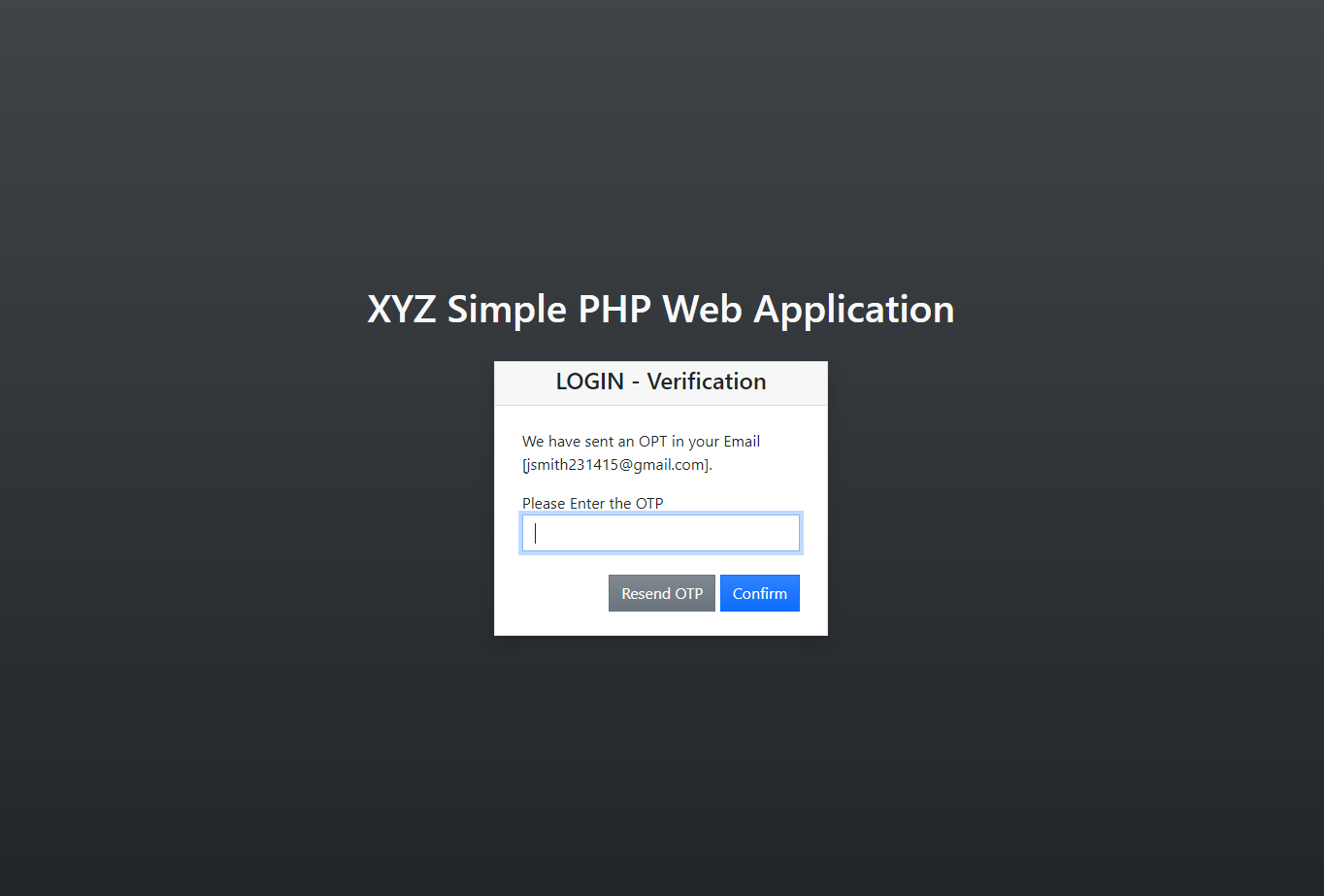
Next, create the index page of the application where the user will be redirected after the successful 2 Factor Authentication. Copy/Paste the code below and configure it the way you want the UI looks. Save the file as index.php.
- <?php
- require_once('auth.php');
- require_once('MainClass.php');
- ?>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <meta http-equiv="X-UA-Compatible" content="IE=edge">
- <meta name="viewport" content="width=device-width, initial-scale=1.0">
- <link rel="stylesheet" href="./Font-Awesome-master/css/all.min.css">
- <link rel="stylesheet" href="./css/bootstrap.min.css">
- <style>
- html,body{
- height:100%;
- width:100%;
- }
- main{
- height:100%;
- display:flex;
- flex-flow:column;
- }
- </style>
- </head>
- <body>
- <main>
- <nav class="navbar navbar-expand-lg navbar-dark bg-dark bg-gradient" id="topNavBar">
- <div class="container">
- <a class="navbar-brand" href="#">
- XYZ Simple PHP Web Application
- </a>
- </div>
- </nav>
- <div class="container py-3" id="page-container">
- <div class="row justify-content-center">
- <div class="col-lg-5 col-md-8 col-sm-12 col-xs-12">
- <div class="card shadow rounded-0">
- <div class="card-body py-4">
- <hr>
- <div class="text-end">
- </div>
- </div>
- </div>
- </div>
- </div>
- </div>
- </main>
- </body>
- </html>
Output
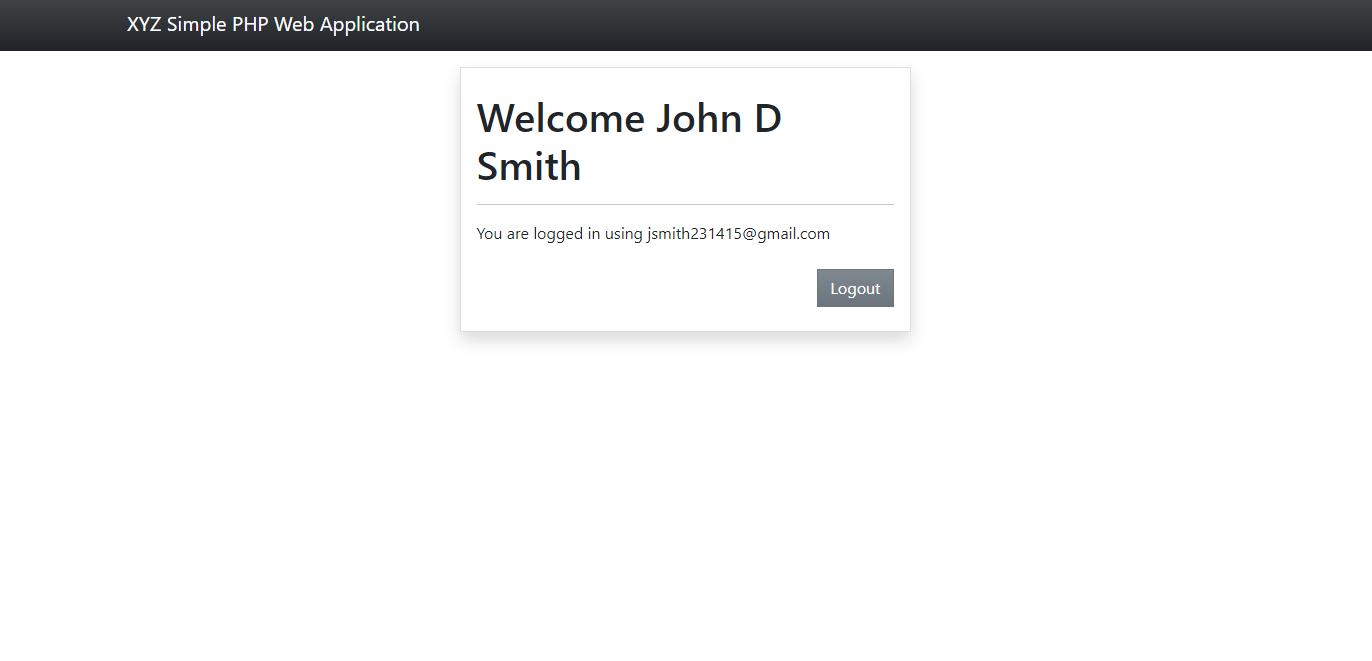
Here's the Sample Snapshot of the OTP Mail
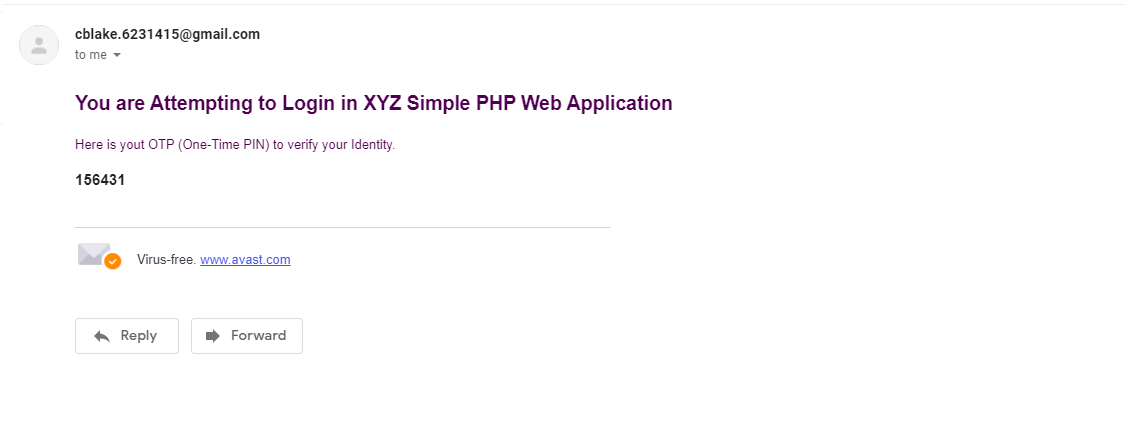
DEMO VIDEO
There you go. You can now test the application on your end and check if it is working properly and achieves the goal I stated above. If there's an error occurred on your end, review your codes with the codes I provided above. You can also download the source code I created for this tutorial. The download link/button is located after this article.
That ends this tutorial. I hope this will help you with what you are looking for and you'll find it useful with your current or future projects.
Explore more on this website for more Tutorials and Free Source Codes.
Related Links: