Simple Dropdown Sort Using PHP
Submitted by razormist on Tuesday, May 19, 2020 - 23:49.
In this article we will create Simple Dropdown Sort using PHP. The program will dynamically sort the table row base on the selected options data. You are free to modify this, and use as you own it. To learn more about this tutorial, just follow the step below.
There you have it we successfully created Simple Dropdown Sort using PHP. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP https://www.apachefriends.org/index.html And, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/Creating Database
Open your database web server then create a database name in it db_dropdown_sort after that click Import then locate the database file inside the folder of the application then click ok.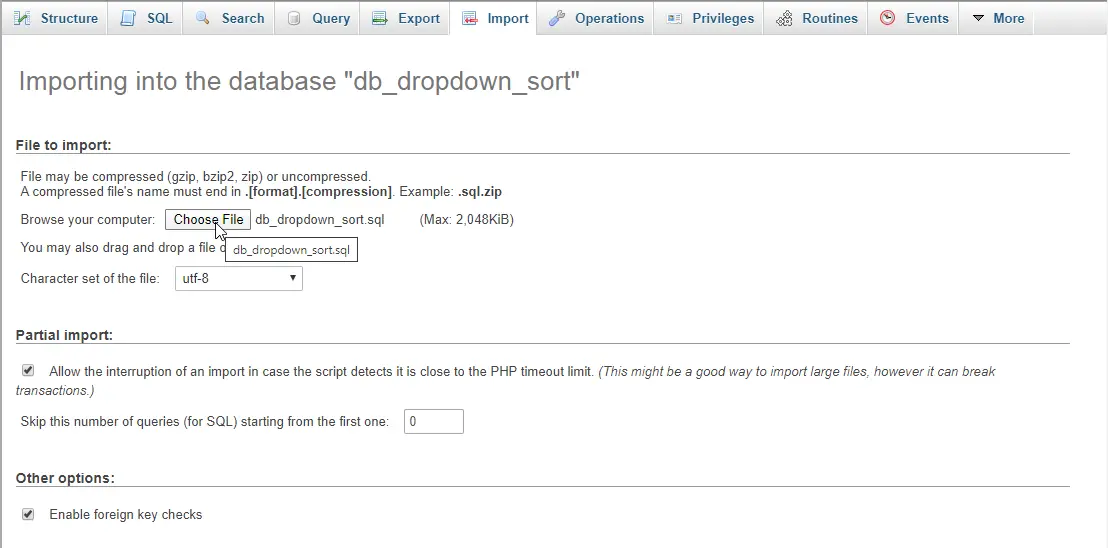
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php- <?php
- if(!$conn){
- }
- ?>
Creating the Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">Simple Dropdown Sort Using PHP</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-8">
- <form method="POST" action="">
- <div class="form-inline">
- <label>Group:</label>
- <select class="form-control" name="group">
- <option value="Reptile">Reptile</option>
- <option value="Mammal">Mammal</option>
- </select>
- <button class="btn btn-primary" name="sort">Sort</button>
- <button class="btn btn-success" name="reset">Reset</button>
- </div>
- </form>
- <br /><br />
- <table class="table table-bordered">
- <thead class="alert-info">
- <th>Group</th>
- <th>Animal</th>
- </thead>
- <thead>
- <?php include'sort.php'?>
- </thead>
- </table>
- </div>
- </div>
- </body>
- </html>
Creating the Main Function
This code contains the main function of the application. The code will display a data base on the selected option To make this just copy and write these block of codes inside the text editor, then save it as sort.php- <?php
- require'conn.php';
- $group=$_POST['group'];
- $query=mysqli_query($conn, "SELECT * FROM `animals` WHERE `n_group`='$group'") or die(mysqli_error());
- echo"<tr><td>".$fetch['n_group']."</td><td>".$fetch['name']."</td></tr>";
- }
- echo"<tr><td>".$fetch['n_group']."</td><td>".$fetch['name']."</td></tr>";
- }
- }else{
- echo"<tr><td>".$fetch['n_group']."</td><td>".$fetch['name']."</td></tr>";
- }
- }
- ?>
Add new comment
- 1282 views