How to Get the Last Index of an Array using JavaScript
How to Get the Last Index of an Array using JavaScript
Introduction
In this tutorial we will create a How to Get the Last Index of an Array using JavaScript. This tutorial purpose is teach you how to get the last data from an array. This will cover all the basic function will change your background I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any application if you want to apply an array object to you system. I will give my best to provide you the easiest way of creating this program Get Last index of an array. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface to our application. This code will only table with data list and button. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-4">
- <table class="table table-bordered">
- <thead>
- <tr class="alert-info">
- </tr>
- </thead>
- <tbody class="alert-success" id="result">
- </tbody>
- </table>
- </div>
- <div class="col-md-8">
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will get the last data from an array when the button is clicked. To do this just copy and write these block of codes inside the text editor and save it as script.js.- let myArray = ["John Smith", "Jake Cyrus", "Steve Roger", "Tony Stark", "Bruce Banner", "Clark Thompson"];
- displayData(myArray);
- function displayData(myArray){
- let html="";
- for(var i=0; i<myArray.length; i++){
- html +="<tr>";
- html +="<td>"+myArray[i]+"</td>";
- html +="</tr>";
- }
- document.getElementById('result').innerHTML=html;
- }
- function getLastIndex(myArray){
- let data = myArray[myArray.length - 1];
- document.getElementById('display').innerHTML="<center><label>The last index of an Array is: <span class='text-primary'>"+data+"</span></label></center>";
- }
- document.getElementById("get").addEventListener("click", getLastIndex.bind(this, myArray));
In the code above we first create an array object called myArray(). Next we will create a method that will display the array data into html table called displayData(). And last we will created a method called getLastIndex(), this function will try to get only the last data from an array object. The trick here is that we will check the position of the last data of an array by subtracting the length of the array.
Output:
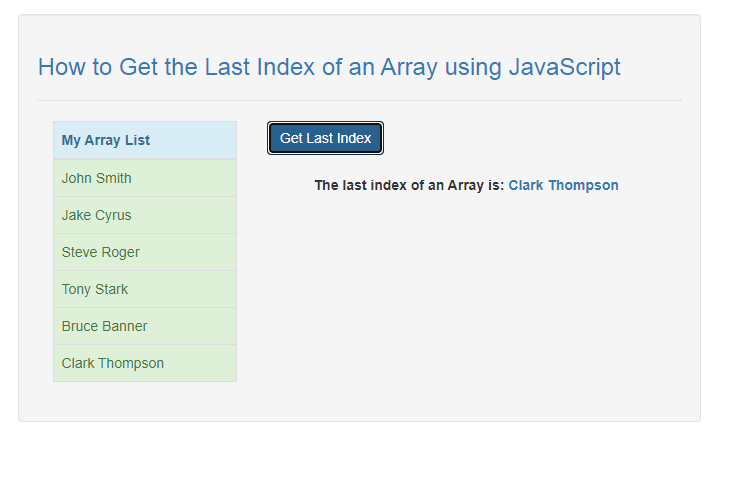
The How to Get the Last Index of an Array using JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Get the Last Index of an Array using JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language