How to Count Cases in a String using JavaScript
How to Count Cases in a String using JavaScript
Introduction
In this tutorial we will create a How to Count Cases in a String using JavaScript. This tutorial purpose is teach you how to count the total lowercase and uppercase in a string. This will cover all the basic function will change your background I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any application that need to count in a certain string. I will give my best to provide you the easiest way of creating this program Count Uppercase and Lowercase in a string. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface to our application. This code will only display textbox and button for submission. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css" />
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-5">
- <div class="form-group">
- <input type="text" id="text" class="form-control" />
- </div>
- </div>
- <div class="col-md-7">
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will count the total cases within a string when submitted. To do this just copy and write these block of codes inside the text editor and save it as script.js.- function countCases() {
- let str = document.getElementById('text').value;
- let upper=0
- let lower=0;
- let len=str.length;
- for(var i=0;i<len;i++){
- if(/[A-Z]/.test(str.charAt(i))){
- upper++;
- }else{
- lower++;
- }
- }
- document.getElementById('result').innerHTML = "<h1 style='font-size:20px;'>UPPER CASE: <span class='text-primary' style='font-size:30px:'>"+upper+"</span></h1><h1 style='font-size:20px;'>LOWER CASE: <span class='text-primary' style='font-size:30px:'>"+lower+"</span></h1>";
- }
- function clearStr(){
- document.getElementById('text').value = "";
- document.getElementById('result').innerHTML = "";
In the code above we just simply create a method called countCase(), this function will count the total Uppercase and Lowecase within a string. The trick here is that we just need to look up for all the Uppercase letter in a string. We just simply use a conditional statement with an argument that regex the matching for all available Uppercase in the string. Otherwise the match didn't return anything this will display the result for the Lowercase.
Output:
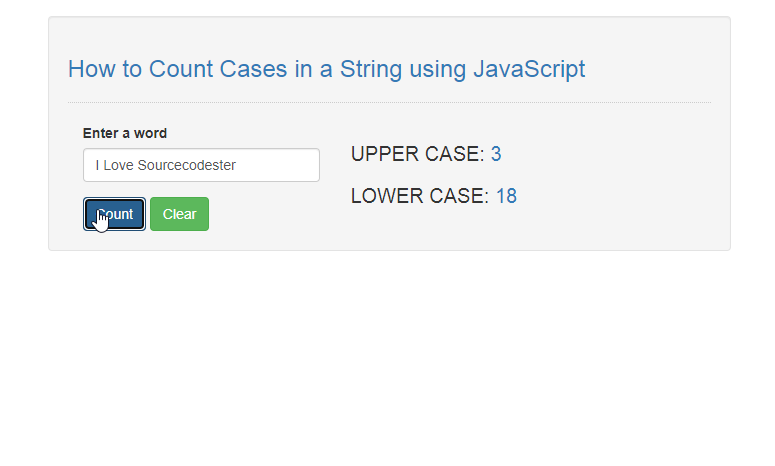
The How to Count Cases in a String using JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Count Cases in a String using JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language