How to Live Resize Background Image in JavaScript
How to Live Resize Background Image in JavaScript
Introduction
In this tutorial we will create a How to Live Resize Background Image in JavaScript. This tutorial purpose is to teach you how you can resize the set background image. This will cover all the important function that will resize your background image. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any system if you want to add a feature that can resize your images. I will give my best to provide you the easiest way of creating this program Live Resize Image. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will only display only images and textboxes. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1" />
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <center>
- <div class="form-inline">
- <input type="number" class="form-control" onkeyup="resizeHeight(this.value);"/>
- <input type="number" class="form-control" onkeyup="resizeWidth(this.value);"/>
- </div>
- </center>
- <hr style="border-top:1px solid #000;"/>
- <center><img src="image.png" id="result" height="300" width="500"/></center>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will let you to resize your background images. To do this just copy and write these block of codes inside the text editor and save it as script.js.- function resizeHeight(val){
- if(val > 600){
- val = 600;
- alert("Height Limit is 300");
- }
- document.getElementById('result').style.height=val+"px";
- }
- function resizeWidth(val){
- if(val > 800){
- val = 800;
- alert("Width Limit is 800");
- }
- document.getElementById('result').style.width=val+"px";
- }
In this code we just simply create two methods that we will use to resize our image. To allow our image to be resize live in html content, we just simply bind the texboxes value with the image actual sizes. Every time you enter a value in the textboxes it will also resize the image size dynamically.
Output:
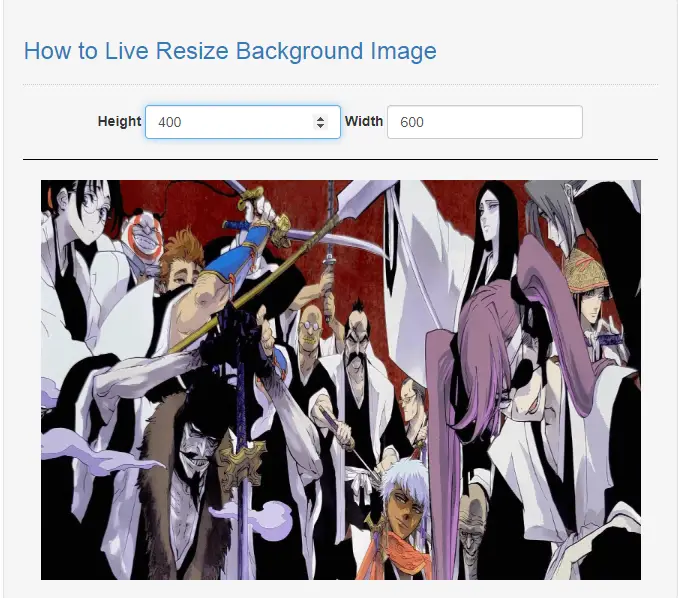
The How to Live Resize Background Image in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Live Resize Background Image in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language
Add new comment
- 320 views