How to Create a Drag and Drop Counter in JavaScript
How to Create a Drag and Drop Counter in JavaScript
Introduction
In this tutorial we will create a How to Create a Drag and Drop Counter in JavaScript. This tutorial purpose is to teach you way to count an object with the use of drag and drop. This will cover all the important function that will drag and drop an object. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any system if you want to add some counter using the drag and drop feature. I will give my best to provide you the easiest way of creating this program Drag and drop counter. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will only display only images and the table data. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="row">
- <div class="col-md-6" style="">
- <center>
- <img src="images/image1.png" id="dog" draggable="true" height="70" width="70" ondragstart="drag(event)" />
- <img src="images/image2.png" id="elephant" draggable="true" height="70" width="70" ondragstart="drag(event)" />
- <img src="images/image3.png" id="lion" draggable="true" height="70" width="70" ondragstart="drag(event)" />
- </center>
- <center><img src="images/image4.png" style="margin-top:60px;" ondrop="drop(event)" ondragover="dragOver(event)" height="100" width="100"/></center>
- </div>
- <div class="col-md-5">
- <table class="table table-bordered">
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- </table>
- </div>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will count the object that you been dropped in the targeted location To do this just copy and write these block of codes inside the text editor and save it as script.js.- let dog = 0;
- let elephant = 0;
- let lion = 0;
- function dragOver(e){
- e.preventDefault();
- }
- function drop(e){
- e.preventDefault();
- let data = e.dataTransfer.getData("data");
- switch(data){
- case "dog":
- dog++;
- document.getElementById('dog_count').innerHTML = dog;
- break;
- case "elephant":
- elephant++;
- document.getElementById('elephant_count').innerHTML = elephant;
- break;
- case "lion":
- lion++;
- document.getElementById('lion_count').innerHTML = lion;
- break;
- }
- }
- function drag(e){
- e.dataTransfer.setData("data", e.target.id);
- }
In the first line of code we just simply set three global variables that we will use you to count. The first method we will create a method called dragOver(), this will prevent the web browser to default the dragging of an object. Next is the drop() method, this function will receive the object data and use it to increment a counter for each dropped object. And last method is what we call drag() method, this function will carry over the object data while dragging it.
Output:
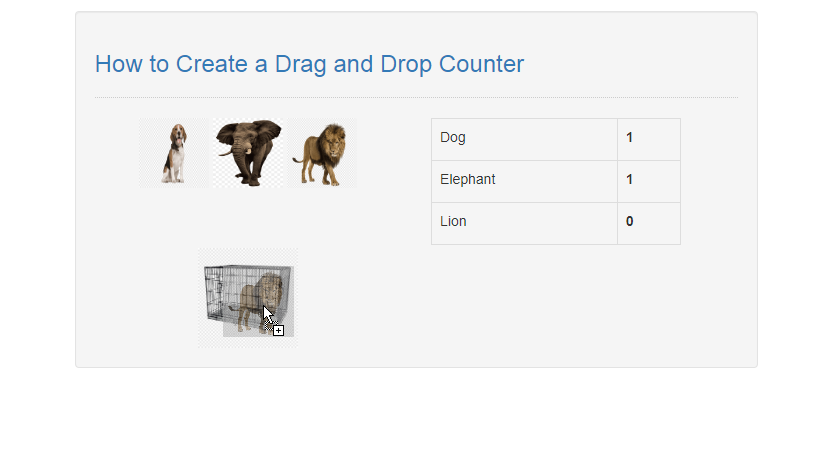
The How to Create a Drag and Drop Counter in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Create a Drag and Drop Counter in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language