How to Sort Array Based on Multiple Fields in JavaScript
What is JavaScript Array?
In JavaScript, an array is somewhat you call an ordered list of values. Each value is called an element with a specific index: A JavaScript array has different characteristics: First, an array can hold values of mixed types. It can have an array that stores elements with the types number, string, boolean, and null.Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will display an interface that will append the array object later. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css" />
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="container-fluid">
- <div class="row">
- <div class="col-md-6 well">
- <hr style="border-top: 1px SOLID #8c8b8b;"/>
- </div>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will code will dynamically sort the array object with a conditional method. To do this just copy and write these block of codes after the body closing tag.- <script>
- var data = [
- {
- "name": "John Smith",
- "age": "21",
- "status": "Non-Regular"},
- {
- "name": "Claire Temple",
- "age": "20",
- "status": "Regular"},
- {
- "name": "Sophia Jose",
- "age": "19",
- "status": "Regular"},
- ];
- function reset(){
- window.location="index.html";
- }
- function sort(){
- let result = data.sort((a,b) =>{
- if(a.status=='Regular'){
- return -1
- }
- return 1
- })
- document.getElementById("display").innerHTML=JSON.stringify(result, null, 2);
- }
- document.getElementById("display").innerHTML=JSON.stringify(data, null, 2);
- </script>
In this code we display first the array object using the JSON.stringify() function. After that when bind the button in onclick function. To sort the array object we use the sort() function that include the sorting option of how we will sort the data. In my case I want to sort out the status object where the Regular value will display at the top first. I use a conditional if to target the status object where the value is Regular sort it on top list.
Output:
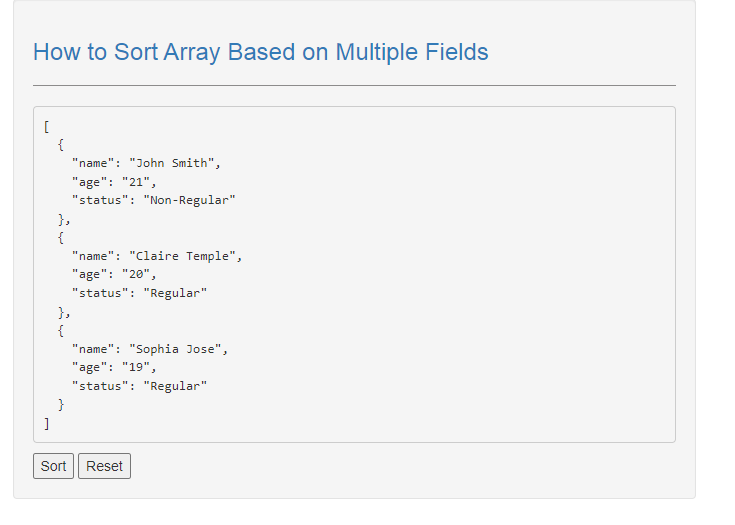
The How to Sort Array Based on Multiple Fields in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Sort Array Based on Multiple Fields in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language