Inline Shopping Cart System In PHP and MySQL Database Tutorial
A shopping cart is an imperative piece of each eCommerce venture. It causes the client to choose various things to buy and view add up to cost before presenting the request. On the off chance that you need to assemble a basic PHP shopping basket without any preparation, this well-ordered instructional exercise will help you a ton. In this instructional exercise, we'll give the entire guide and content to making a straightforward shopping cart in PHP.
This tutorial exercise is outlined in a way that can be actualized effectively in PHP venture and made straightforward the shopping cart idea. In our case content, we'll utilize PHP and jquery to store the items data in the truck. Once the request is put together by the client, the item data would be embedded into the database utilizing PHP and MySQL.
Prerequisites
For the purpose of this tutorial, I assume that you have PHP powered stack (a LAMP stack would do)on your web server.
To make sure that that I don’t get sidetracked by server-level issues, I decided to host my app on Cloudways PHP MySql hosting because it takes care of server and app setup and offers a powerful dev stack right out of the box.
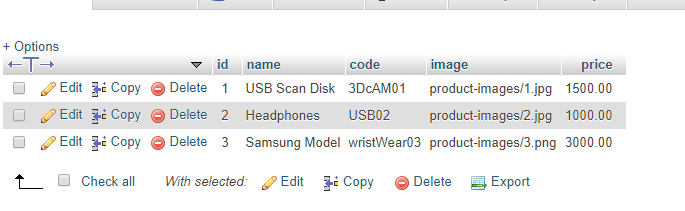
Create Database Tables
First of all, create a new database naming shopping_cart
. Minimum creates two tables like products and cart. Let’s paste the following MySQL code.
Cart Table
- CREATE TABLE `tbl_cart` (
- `id` INT(11) NOT NULL AUTO_INCREMENT,
- `product_id` INT(11) NOT NULL,
- `quantity` INT(11) NOT NULL,
- `member_id` INT(11) NOT NULL
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Products Table
- CREATE TABLE `tbl_product` (
- `id` INT(8) NOT NULL AUTO_INCREMENT,
- `name` VARCHAR(255) NOT NULL,
- `code` VARCHAR(255) NOT NULL,
- `image` text NOT NULL,
- `price` DOUBLE(10,2) NOT NULL
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
- INSERT INTO `tbl_product` (`id`, `name`, `code`, `image`, `price`) VALUES
- (1, '3D Camera', '3DcAM01', 'product-images/camera.jpg', 1500.00),
- (2, 'External Hard Drive', 'USB02', 'product-images/external-hard-drive.jpg', 800.00),
- (3, 'Wrist Watch', 'wristWear03', 'product-images/watch.jpg', 300.00);
DB Connection
Create the DBController.php
file and paste following code.
- <?php
- <?php
- class DBController
- {
- private $host = "localhost";
- private $user = "root";
- private $password = "";
- private $database = "shopping_cart";
- private $conn;
- function __construct()
- {
- }
- public static function getConnection()
- {
- new Database();
- }
- }
- {
- $sql_statement = $this->conn->prepare($query);
- $this->bindParams($sql_statement, $params);
- }
- $sql_statement->execute();
- $result = $sql_statement->get_result();
- if ($result->num_rows > 0) {
- while ($row = $result->fetch_assoc()) {
- $resultset[] = $row;
- }
- }
- return $resultset;
- }
- }
- {
- $sql_statement = $this->conn->prepare($query);
- $this->bindParams($sql_statement, $params);
- }
- $sql_statement->execute();
- }
- function bindParams($sql_statement, $params)
- {
- $param_type = "";
- foreach ($params as $query_param) {
- $param_type .= $query_param["param_type"];
- }
- $bind_params[] = & $param_type;
- foreach ($params as $k => $query_param) {
- $bind_params[] = & $params[$k]["param_value"];
- }
- $sql_statement,
- 'bind_param'
- ), $bind_params);
- }
- }
Creating Template Files
I’m going to creating template files . Let’s create index.php
file and paste the following code.
- <?php
- <?php
- require_once "ShoppingCart.php";
- $member_id = 2; // you can your integerate authentication module here to get logged in member
- $shoppingCart = new ShoppingCart();
- switch ($_GET["action"]) {
- case "add":
- $productResult = $shoppingCart->getProductByCode($_GET["code"]);
- $cartResult = $shoppingCart->getCartItemByProduct($productResult[0]["id"], $member_id);
- // Update cart item quantity in database
- $newQuantity = $cartResult[0]["quantity"] + $_POST["quantity"];
- $shoppingCart->updateCartQuantity($newQuantity, $cartResult[0]["id"]);
- } else {
- // Add to cart table
- $shoppingCart->addToCart($productResult[0]["id"], $_POST["quantity"], $member_id);
- }
- }
- break;
- case "remove":
- // Delete single entry from the cart
- $shoppingCart->deleteCartItem($_GET["id"]);
- break;
- case "empty":
- // Empty cart
- $shoppingCart->emptyCart($member_id);
- break;
- }
- }
- ?>
- <HTML>
- <HEAD>
- <TITLE>Shopping Cart in PHP</TITLE>
- <meta name="viewport" content="width=device-width, initial-scale=1">
- <link href="style.css" type="text/css" rel="stylesheet" />
- <script src="jquery-3.2.1.min.js"></script>
- <script>
- function increment_quantity(cart_id, price) {
- var inputQuantityElement = $("#input-quantity-"+cart_id);
- var newQuantity = parseInt($(inputQuantityElement).val())+1;
- var newPrice = newQuantity * price;
- save_to_db(cart_id, newQuantity, newPrice);
- }
- function decrement_quantity(cart_id, price) {
- var inputQuantityElement = $("#input-quantity-"+cart_id);
- if($(inputQuantityElement).val() > 1)
- {
- var newQuantity = parseInt($(inputQuantityElement).val()) - 1;
- var newPrice = newQuantity * price;
- save_to_db(cart_id, newQuantity, newPrice);
- }
- }
- function save_to_db(cart_id, new_quantity, newPrice) {
- var inputQuantityElement = $("#input-quantity-"+cart_id);
- var priceElement = $("#cart-price-"+cart_id);
- $.ajax({
- url : "update_cart_quantity.php",
- data : "cart_id="+cart_id+"&new_quantity="+new_quantity,
- type : 'post',
- success : function(response) {
- $(inputQuantityElement).val(new_quantity);
- $(priceElement).text("$"+newPrice);
- var totalQuantity = 0;
- $("input[id*='input-quantity-']").each(function() {
- var cart_quantity = $(this).val();
- totalQuantity = parseInt(totalQuantity) + parseInt(cart_quantity);
- });
- $("#total-quantity").text(totalQuantity);
- var totalItemPrice = 0;
- $("div[id*='cart-price-']").each(function() {
- var cart_price = $(this).text().replace("$","");
- totalItemPrice = parseInt(totalItemPrice) + parseInt(cart_price);
- });
- $("#total-price").text(totalItemPrice);
- }
- });
- }
- </script>
- </HEAD>
- <BODY>
- <?php
- $cartItem = $shoppingCart->getMemberCartItem($member_id);
- $item_quantity = 0;
- $item_price = 0;
- foreach ($cartItem as $item) {
- $item_quantity = $item_quantity + $item["quantity"];
- $item_price = $item_price + ($item["price"] * $item["quantity"]);
- }
- }
- ?>
- <div id="shopping-cart">
- <div class="txt-heading">
- <div class="txt-heading-label"><h1>Shopping Cart</h1></div>
- <a id="btnEmpty" href="index.php?action=empty"><img
- src="empty-cart.png" alt="empty-cart" title="Empty Cart"
- class="float-right" /></a>
- <div class="cart-status">
- <div>Total Quantity: <span id="total-quantity"><?php echo $item_quantity; ?></span></div>
- <div>Total Price: <span id="total-price"><?php echo $item_price; ?></span></div>
- </div>
- </div>
- <?php
- ?>
- <div class="shopping-cart-table">
- <div class="cart-item-container header">
- <div class="cart-info title">Title</div>
- <div class="cart-info">Quantity</div>
- <div class="cart-info price">Price</div>
- </div>
- <?php
- foreach ($cartItem as $item) {
- ?>
- <div class="cart-item-container">
- <div class="cart-info title">
- <?php echo $item["name"]; ?>
- </div>
- <div class="cart-info quantity">
- <div class="btn-increment-decrement" onClick="decrement_quantity(<?php echo $item["cart_id"]; ?>, '<?php echo $item["price"]; ?>')">-</div><input class="input-quantity"
- id="input-quantity-<?php echo $item["cart_id"]; ?>" value="<?php echo $item["quantity"]; ?>"><div class="btn-increment-decrement"
- onClick="increment_quantity(<?php echo $item["cart_id"]; ?>, '<?php echo $item["price"]; ?>')">+</div>
- </div>
- <div class="cart-info price" id="cart-price-<?php echo $item["cart_id"]; ?>">
- <?php echo "$". ($item["price"] * $item["quantity"]); ?>
- </div>
- <div class="cart-info action">
- <a
- href="index.php?action=remove&id=<?php echo $item["cart_id"]; ?>"
- class="btnRemoveAction"><img
- src="icon-delete.png" alt="icon-delete"
- title="Remove Item" /></a>
- </div>
- </div>
- <?php
- }
- ?>
- </div>
- </div>
- <?php
- }
- ?>
- </div>
- <?php require_once "product-list.php"; ?>
- </BODY>
- </HTML>
Displaying Products
The below code is template to display that product list. Save the below code as product-list.php
.
- <div id="product-grid">
- <div class="txt-heading">
- <div class="txt-heading-label">Products</div>
- </div>
- <?php
- $query = "SELECT * FROM tbl_product";
- $product_array = $shoppingCart->getAllProduct($query);
- foreach ($product_array as $key => $value) {
- ?>
- <div class="product-item">
- <form method="post"
- action="index.php?action=add&code=<?php echo $product_array[$key]["code"]; ?>">
- <div class="product-image">
- <img src="<?php echo $product_array[$key]["image"]; ?>">
- <div class="product-title">
- <?php echo $product_array[$key]["name"]; ?>
- </div>
- </div>
- <div class="product-footer">
- <div class="float-right">
- <input type="text" name="quantity" value="1"
- size="2" class="input-cart-quantity" /><input type="image"
- src="add-to-cart.png" class="btnAddAction" />
- </div>
- <div class="product-price float-left" id="product-price-<?php echo $product_array[$key]["code"]; ?>"><?php echo "$".$product_array[$key]["price"]; ?></div>
- </div>
- </form>
- </div>
- <?php
- }
- }
- ?>
- </div>
Shopping Cart Class
I am going to create a shopping cart just create the file name ShoppingCart.php
and paste the following code. This Class called Shopping Cart and different functions like add, delete, update.
- <?php
- require_once "DBController.php";
- class ShoppingCart extends DBController
- {
- function getAllProduct()
- {
- $query = "SELECT * FROM tbl_product";
- $productResult = $this->getDBResult($query);
- return $productResult;
- }
- function getMemberCartItem($member_id)
- {
- $query = "SELECT tbl_product.*, tbl_cart.id as cart_id,tbl_cart.quantity FROM tbl_product, tbl_cart WHERE
- tbl_product.id = tbl_cart.product_id AND tbl_cart.member_id = ?";
- "param_type" => "i",
- "param_value" => $member_id
- )
- );
- $cartResult = $this->getDBResult($query, $params);
- return $cartResult;
- }
- function getProductByCode($product_code)
- {
- $query = "SELECT * FROM tbl_product WHERE code=?";
- "param_type" => "s",
- "param_value" => $product_code
- )
- );
- $productResult = $this->getDBResult($query, $params);
- return $productResult;
- }
- function getCartItemByProduct($product_id, $member_id)
- {
- $query = "SELECT * FROM tbl_cart WHERE product_id = ? AND member_id = ?";
- "param_type" => "i",
- "param_value" => $product_id
- ),
- "param_type" => "i",
- "param_value" => $member_id
- )
- );
- $cartResult = $this->getDBResult($query, $params);
- return $cartResult;
- }
- function addToCart($product_id, $quantity, $member_id)
- {
- $query = "INSERT INTO tbl_cart (product_id,quantity,member_id) VALUES (?, ?, ?)";
- "param_type" => "i",
- "param_value" => $product_id
- ),
- "param_type" => "i",
- "param_value" => $quantity
- ),
- "param_type" => "i",
- "param_value" => $member_id
- )
- );
- $this->updateDB($query, $params);
- }
- function updateCartQuantity($quantity, $cart_id)
- {
- $query = "UPDATE tbl_cart SET quantity = ? WHERE id= ?";
- "param_type" => "i",
- "param_value" => $quantity
- ),
- "param_type" => "i",
- "param_value" => $cart_id
- )
- );
- $this->updateDB($query, $params);
- }
- function deleteCartItem($cart_id)
- {
- $query = "DELETE FROM tbl_cart WHERE id = ?";
- "param_type" => "i",
- "param_value" => $cart_id
- )
- );
- $this->updateDB($query, $params);
- }
- function emptyCart($member_id)
- {
- $query = "DELETE FROM tbl_cart WHERE member_id = ?";
- "param_type" => "i",
- "param_value" => $member_id
- )
- );
- $this->updateDB($query, $params);
- }
- }
Update cart quantity
The code below is the PHP script that serves as the update operation of each product's quantity in the cart when adding or decreasing button is triggered. Save the file as update_cart_quantity.php
.
- <?php
- require_once "ShoppingCart.php";
- $member_id = 2; // you can your integerate authentication module here to get logged in member
- $shoppingCart = new ShoppingCart();
- $shoppingCart->updateCartQuantity($_POST["new_quantity"], $_POST["cart_id"]);
- ?>
Demo
Conclusion
We trust this guide will enable you to comprehend the essential shopping to truck usefulness in PHP with session and MySQL. Utilizing this procedure and content you'll have the capacity to execute a shopping basket in your web application in a flash. Additionally, you can update it with cutting-edge usefulness in view of your shopping basket prerequisite.
That's the end of this tutorial and I hope this will help you with what you are looking for. The working source sample that I have created for this tutorial is included, kindly click the "download" button below to download the source code zip file.
Enjoy :)Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Add new comment
- 5402 views