Creating an AngularJS CRUD App with Search, Sort and Pagination with PHP/MySQLi
This is a CRUD(Create, Read, Update and Delete) using Angular JS with the help of PHP and MySQLi to handle our database. Also includes how to filter/search data, sort data and paginate data from our MySQL Database. Angular JS is a javascript framework maintained by Google and is capable of creating Single-Paged Applications.
Getting Started
I've used CDN for Bootstrap, Font-awesome, and Angular JS so you need an internet connection for them to work.
Creating our Database
First, let's create our database and if you want, you can insert sample given data as well.
- Open PHPMyAdmin.
- Click databases, create a database and name it as angular.
- After creating a database, click the SQL and paste the below codes. See the image below for detailed instructions.
This is our sample data:
- (1, 'Neovic', 'Devierte', 'Silay City'),
- (2, 'Julyn', 'Divinagracia', 'E.B. Magalona'),
- (3, 'Gemalyn', 'Cepe', 'Bohol'),
- (4, 'Matet', 'Devierte', 'Silay City'),
- (5, 'Tintin', 'Devierte', 'Silay City'),
- (6, 'Bien', 'Devierte', 'Cebu City'),
- (7, 'Cherry', 'Ambayec', 'Cebu City'),
- (8, 'Jubilee', 'Limsiaco', 'Silay City'),
- (9, 'Janna ', 'Atienza', 'Talisay City'),
- (10, 'Desire', 'Osorio', 'Bacolod City'),
- (11, 'Debbie', 'Osorio', 'Talisay City'),
- (12, 'Nipoy ', 'Polondaya', 'Victorias City'),
- (13, 'Johnedel', 'Balino', 'Cauyan, Negros'),
- (14, 'Nereca', 'Tajonera', 'Cauayan, Negros'),
- (15, 'Jerome', 'Robles', 'Cebu City');
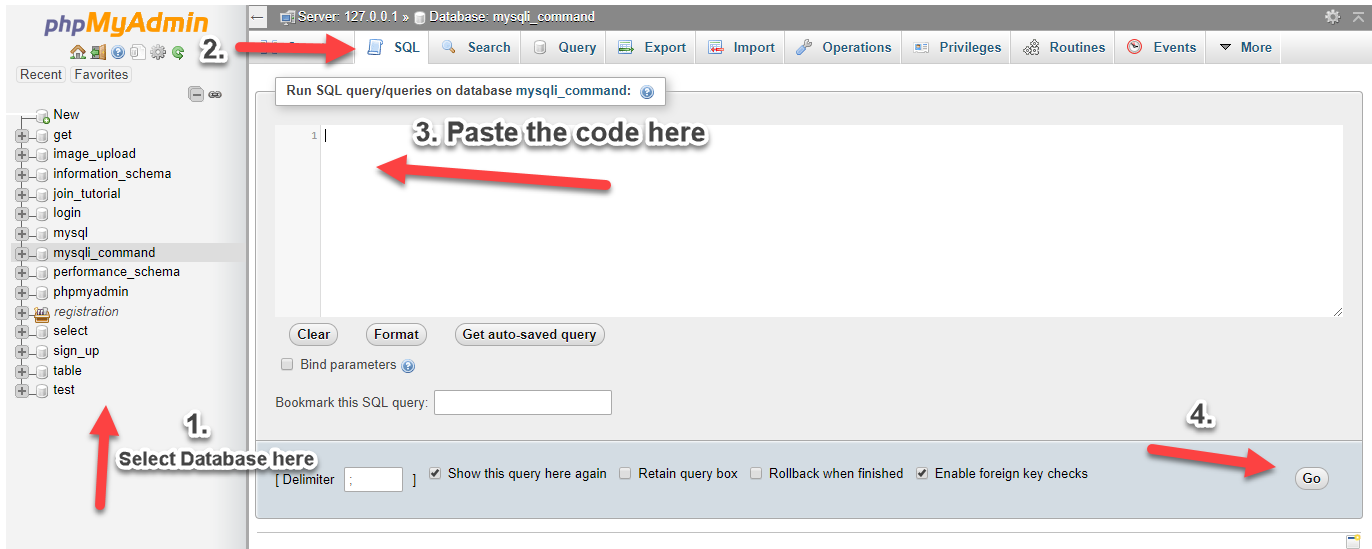
Creating our Connection
Next, we're gonna create our connection to our database which we are going to call every time we have business with the database. We name this as conn.php
.
- <?php
- $conn = new mysqli('localhost', 'root', '', 'angular');
- if ($conn->connect_error) {
- }
- ?>
index.php
Next, we're gonna create our index which contains our table.
- <!DOCTYPE html>
- <html lang="en" ng-app="app">
- <head>
- <meta charset="utf-8">
- <title>AngularJS CRUD with Search, Sort and Pagination</title>
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- <link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet">
- <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
- <link rel="stylesheet" type="text/css" href="style.css">
- </head>
- <body ng-controller="memberdata" ng-init="fetch()">
- <div class="container">
- <h1 class="page-header text-center">AngularJS CRUD with Search, Sort and Pagination</h1>
- <div class="row">
- <div class="col-md-8 col-md-offset-2">
- <div class="alert alert-success text-center" ng-show="success">
- <button type="button" class="close" ng-click="clearMessage()"><span aria-hidden="true">×</span></button>
- <i class="fa fa-check"></i> {{ successMessage }}
- </div>
- <div class="alert alert-danger text-center" ng-show="error">
- <button type="button" class="close" ng-lick="clearMessage()"><span aria-hidden="true">×</span></button>
- <i class="fa fa-warning"></i> {{ errorMessage }}
- </div>
- <div class="row">
- <div class="col-md-12">
- <button href="" class="btn btn-primary" ng-click="showAdd()"><i class="fa fa-plus"></i> New Member</button>
- <span class="pull-right">
- <input type="text" ng-model="search" class="form-control" placeholder="Search">
- </span>
- </div>
- </div>
- <table class="table table-bordered table-striped" style="margin-top:10px;">
- <thead>
- <tr>
- <th ng-click="sort('firstname')" class="text-center">First Name
- <span class="pull-right">
- <i class="fa fa-sort gray" ng-show="sortKey!='firstname'"></i>
- <i class="fa fa-sort" ng-show="sortKey=='firstname'" ng-class="{'fa fa-sort-asc':reverse,'fa fa-sort-desc':!reverse}"></i>
- </span>
- </th>
- <th ng-click="sort('lastname')" class="text-center">Last Name
- <span class="pull-right">
- <i class="fa fa-sort gray" ng-show="sortKey!='lastname'"></i>
- <i class="fa fa-sort" ng-show="sortKey=='lastname'" ng-class="{'fa fa-sort-asc':reverse,'fa fa-sort-desc':!reverse}"></i>
- </span>
- </th>
- <th ng-click="sort('address')" class="text-center">Address
- <span class="pull-right">
- <i class="fa fa-sort gray" ng-show="sortKey!='address'"></i>
- <i class="fa fa-sort" ng-show="sortKey=='address'" ng-class="{'fa fa-sort-asc':reverse,'fa fa-sort-desc':!reverse}"></i>
- </span>
- </th>
- <th class="text-center">Action</th>
- </tr>
- </thead>
- <tbody>
- <tr dir-paginate="member in members|orderBy:sortKey:reverse|filter:search|itemsPerPage:5">
- <td>{{ member.firstname }}</td>
- <td>{{ member.lastname }}</td>
- <td>{{ member.address }}</td>
- <td>
- <button type="button" class="btn btn-success" ng-click="showEdit(); selectMember(member);"><i class="fa fa-edit"></i> Edit</button>
- <button type="button" class="btn btn-danger" ng-click="showDelete(); selectMember(member);"> <i class="fa fa-trash"></i> Delete</button>
- </td>
- </tr>
- </tbody>
- </table>
- <div class="pull-right" style="margin-top:-30px;">
- <dir-pagination-controls
- max-size="5"
- direction-links="true"
- boundary-links="true" >
- </dir-pagination-controls>
- </div>
- </div>
- </div>
- <?php include('modal.php'); ?>
- </div>
- <script src="https://rawgit.com/michaelbromley/angularUtils-pagination/master/dirPagination.js"></script>
- <script src="angular.js"></script>
- </body>
- </html>
angular.js
This contains our angular JS codes.
- var app = angular.module('app', ['angularUtils.directives.dirPagination']);
- app.controller('memberdata',function($scope, $http, $window){
- $scope.AddModal = false;
- $scope.EditModal = false;
- $scope.DeleteModal = false;
- $scope.errorFirstname = false;
- $scope.showAdd = function(){
- $scope.firstname = null;
- $scope.lastname = null;
- $scope.address = null;
- $scope.errorFirstname = false;
- $scope.errorLastname = false;
- $scope.errorAddress = false;
- $scope.AddModal = true;
- }
- $scope.fetch = function(){
- $http.get("fetch.php").success(function(data){
- $scope.members = data;
- });
- }
- $scope.sort = function(keyname){
- $scope.sortKey = keyname;
- $scope.reverse = !$scope.reverse;
- }
- $scope.clearMessage = function(){
- $scope.success = false;
- $scope.error = false;
- }
- $scope.addnew = function(){
- $http.post(
- "add.php", {
- 'firstname': $scope.firstname,
- 'lastname': $scope.lastname,
- 'address':$scope.address,
- }
- ).success(function(data) {
- if(data.firstname){
- $scope.errorFirstname = true;
- $scope.errorLastname = false;
- $scope.errorAddress = false;
- $scope.errorMessage = data.message;
- $window.document.getElementById('firstname').focus();
- }
- else if(data.lastname){
- $scope.errorFirstname = false;
- $scope.errorLastname = true;
- $scope.errorAddress = false;
- $scope.errorMessage = data.message;
- $window.document.getElementById('lastname').focus();
- }
- else if(data.address){
- $scope.errorFirstname = false;
- $scope.errorLastname = false;
- $scope.errorAddress = true;
- $scope.errorMessage = data.message;
- $window.document.getElementById('address').focus();
- }
- else if(data.error){
- $scope.errorFirstname = false;
- $scope.errorLastname = false;
- $scope.errorAddress = false;
- $scope.error = true;
- $scope.errorMessage = data.message;
- }
- else{
- $scope.AddModal = false;
- $scope.success = true;
- $scope.successMessage = data.message;
- $scope.fetch();
- }
- });
- }
- $scope.selectMember = function(member){
- $scope.clickMember = member;
- }
- $scope.showEdit = function(){
- $scope.EditModal = true;
- }
- $scope.updateMember = function(){
- $http.post("edit.php", $scope.clickMember)
- .success(function(data) {
- if(data.error){
- $scope.error = true;
- $scope.errorMessage = data.message;
- $scope.fetch();
- }
- else{
- $scope.success = true;
- $scope.successMessage = data.message;
- }
- });
- }
- $scope.showDelete = function(){
- $scope.DeleteModal = true;
- }
- $scope.deleteMember = function(){
- $http.post("delete.php", $scope.clickMember)
- .success(function(data) {
- if(data.error){
- $scope.error = true;
- $scope.errorMessage = data.message;
- }
- else{
- $scope.success = true;
- $scope.successMessage = data.message;
- $scope.fetch();
- }
- });
- }
- });
fetch.php
This is our PHP code/api that fetches data from our MySQL Table.
- <?php
- include('conn.php');
- $sql = "SELECT * FROM members";
- $query=$conn->query($sql);
- while($row=$query->fetch_array()){
- $output[] = $row;
- }
- ?>
modal.php
This contains our customize modal for inserting, editing, and deleting data in our MySQL Table.
- <!-- Add Modal -->
- <div class="myModal" ng-show="AddModal">
- <div class="modalContainer">
- <div class="modalHeader">
- </div>
- <div class="modalBody">
- <div class="form-group">
- <input type="text" class="form-control" ng-model="firstname" id="firstname">
- </div>
- <div class="form-group">
- <input type="text" class="form-control" ng-model="lastname" id="lastname">
- </div>
- <div class="form-group">
- <input type="text" class="form-control" ng-model="address" id="address">
- </div>
- </div>
- <hr>
- <div class="modalFooter">
- <div class="footerBtn pull-right">
- </div>
- </div>
- </div>
- </div>
- <!-- Edit Modal -->
- <div class="myModal" ng-show="EditModal">
- <div class="modalContainer">
- <div class="editHeader">
- </div>
- <div class="modalBody">
- <div class="form-group">
- <input type="text" class="form-control" ng-model="clickMember.firstname">
- </div>
- <div class="form-group">
- <input type="text" class="form-control" ng-model="clickMember.lastname">
- </div>
- <div class="form-group">
- <input type="text" class="form-control" ng-model="clickMember.address">
- </div>
- </div>
- <hr>
- <div class="modalFooter">
- <div class="footerBtn pull-right">
- </div>
- </div>
- </div>
- </div>
- <!-- Delete Modal -->
- <div class="myModal" ng-show="DeleteModal">
- <div class="modalContainer">
- <div class="deleteHeader">
- </div>
- <div class="modalBody">
- </div>
- <hr>
- <div class="modalFooter">
- <div class="footerBtn pull-right">
- </div>
- </div>
- </div>
- </div>
style.css
This contains our custom CSS, especially for our custom modal.
- .gray{
- color:gray;
- }
- .input-error{
- font-size:12px;
- color:#f44336;
- }
- .myModal{
- position:fixed;
- top:0;
- left:0;
- right:0;
- bottom:0;
- background: rgba(0, 0, 0, 0.4);
- z-index:100;
- }
- .modalContainer{
- width: 555px;
- background: #FFFFFF;
- margin:auto;
- margin-top:10px;
- }
- .modalHeader{
- padding:10px;
- background: #008CBA;
- color: #FFFFFF;
- height:50px;
- font-size:20px;
- padding-left:15px;
- }
- .editHeader{
- padding:10px;
- background: #4CAF50;
- color: #FFFFFF;
- height:50px;
- font-size:20px;
- padding-left:15px;
- }
- .deleteHeader{
- padding:10px;
- background: #f44336;
- color: #FFFFFF;
- height:50px;
- font-size:20px;
- padding-left:15px;
- }
- .modalBody{
- padding:30px;
- }
- .modalFooter{
- height:36px;
- }
- .footerBtn{
- margin-right:10px;
- margin-top:-9px;
- }
- .closeBtn{
- background: #008CBA;
- color: #FFFFFF;
- border:none;
- }
- .closeEditBtn{
- background: #4CAF50;
- color: #FFFFFF;
- border:none;
- }
- .closeDelBtn{
- background: #f44336;
- color: #FFFFFF;
- border:none;
- }
add.php
This is our PHP code/api in inserting data into our database.
- <?php
- include('conn.php');
- $firstname = $data->firstname;
- $lastname = $data->lastname;
- $address = $data->address;
- $out['firstname'] = true;
- $out['message'] = 'Firstname is required';
- }
- $out['lastname'] = true;
- $out['message'] = 'Lastname is required';
- }
- $out['address'] = true;
- $out['message'] = 'Address is required';
- }
- else{
- $sql = "INSERT INTO members (firstname, lastname, address) VALUES ('$firstname', '$lastname', '$address')";
- $query = $conn->query($sql);
- if($query){
- $out['message'] = 'Member Added Successfully';
- }
- else{
- $out['error'] = true;
- $out['message'] = 'Cannot Add Member';
- }
- }
- ?>
edit.php
This is our PHP code/api is updating our MySQL Table row.
- <?php
- include('conn.php');
- $firstname = $data->firstname;
- $lastname = $data->lastname;
- $address = $data->address;
- $memid = $data->memid;
- $sql = "UPDATE members SET firstname = '$firstname', lastname = '$lastname', address = '$address' WHERE memid = '$memid'";
- $query = $conn->query($sql);
- if($query){
- $out['message'] = 'Member updated Successfully';
- }
- else{
- $out['error'] = true;
- $out['message'] = 'Cannot update Member';
- }
- ?>
delete.php
Lastly, this is our PHP code/API in deleting the MySQL Table row.
- <?php
- include('conn.php');
- $memid = $data->memid;
- $sql = "DELETE FROM members WHERE memid = '$memid'";
- $query = $conn->query($sql);
- if($query){
- $out['message'] = 'Member deleted Successfully';
- }
- else{
- $out['error'] = true;
- $out['message'] = 'Cannot delete Member';
- }
- ?>
That's the end of our tutorial. I hope this Angular JS CRUD APP with Search, Sort, and Pagination With PHP/MySQLi tutorial will help you with what you are looking for, and hopefully, this will add up to your knowledge of developing an Angular JS App.
Explore more on this website for more tutorials and free source code.
Enjoy :)Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Comments
Add new comment
- Add new comment
- 5557 views