PHP - OOP CRUD Operation using Angular.js
Submitted by nurhodelta_17 on Thursday, November 2, 2017 - 21:33.
Language
Getting Started
Please take note that CSS and angular.js used in this tutorial are hosted so you need internet connection for them to work.Creating our Database
1. Open phpMyAdmin. 2. Click databases, create a database and name it as crud_angular. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.- CREATE TABLE `member` (
- `memberid` INT(11) NOT NULL AUTO_INCREMENT,
- `firstname` VARCHAR(50) NOT NULL,
- `lastname` VARCHAR(50) NOT NULL,
- PRIMARY KEY(`memberid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
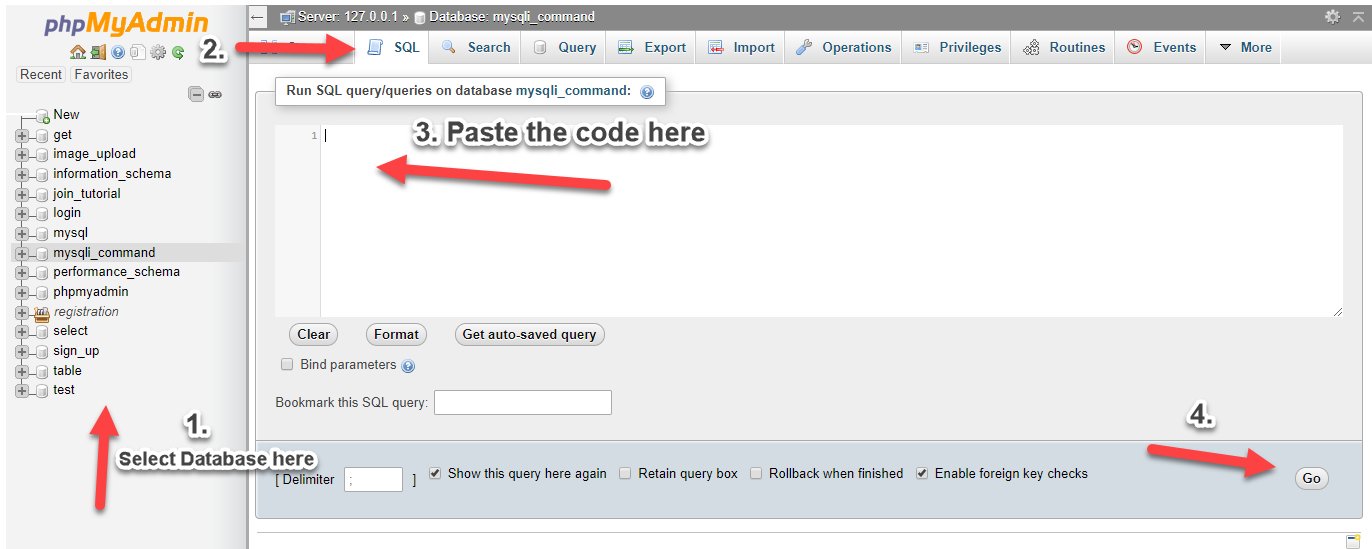
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as conn.php.- <?php
- $conn = new mysqli("localhost", "root", "", "crud_angular");
- if ($conn->connect_error) {
- }
- ?>
index.php
This is our index which contains our form and our table.- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="UTF-8">
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- </head>
- <body>
- <div class="container">
- <div class="row">
- <div ng-app="mem_app" ng-controller="controller" ng-init="showdata()">
- <div class="col-md-4">
- <form ng-submit="myFunc()">
- <hr>
- <div class="form-group">
- <input type="text" class="form-control" id="firstname" name="firstname" ng-model="firstname" placeholder="Enter Firstname">
- </div>
- <div class="form-group">
- <input type="text" class="form-control" id="lastname" name="lastname" ng-model="lastname" placeholder="Enter Lastname">
- </div>
- <hr>
- </form>
- </div>
- <div class="col-md-8">
- <table class="table table-bordered table-striped">
- <thead>
- </thead>
- <tbody>
- <tr ng-repeat="mem in member">
- <input type="hidden" value="{{mem.memberid}}">
- <td>
- </td>
- </tr>
- </tbody>
- </table>
- </div>
- </div>
- </div>
- </div>
- </body>
- </html>
myangular.js
This contains our angular js codes.- var app = angular.module("mem_app", []);
- app.controller("controller", function($scope, $http) {
- $scope.btnName = "Save";
- $scope.icon = "glyphicon glyphicon-floppy-disk";
- $scope.btnClass = "btn btn-primary";
- $scope.insert = function() {
- if ($scope.firstname == null) {
- alert("Please input Firstname");
- }
- else if ($scope.lastname == null) {
- alert("Please input Lastname");
- }
- else {
- $http.post(
- "action.php", {
- 'firstname': $scope.firstname,
- 'lastname': $scope.lastname,
- 'btnName': $scope.btnName,
- 'memberid': $scope.memberid,
- }
- ).success(function(data) {
- alert(data);
- $scope.firstname = null;
- $scope.lastname = null;
- $scope.btnName = "Save";
- $scope.icon = "glyphicon glyphicon-floppy-disk";
- $scope.btnClass = "btn btn-primary";
- $scope.showdata();
- });
- }
- }
- $scope.showdata = function() {
- $http.get("fetch.php")
- .success(function(data) {
- $scope.member = data;
- });
- }
- $scope.update = function(memberid, firstname, lastname) {
- $scope.memberid = memberid;
- $scope.firstname = firstname;
- $scope.lastname = lastname;
- $scope.icon = "glyphicon glyphicon-check";
- $scope.btnClass = "btn btn-success";
- $scope.btnName = "Update";
- }
- $scope.delete= function(memberid) {
- if (confirm("Are you sure you want to delete member?")) {
- $http.post("delete.php", {
- 'memberid': memberid
- })
- .success(function(data) {
- alert(data);
- $scope.showdata();
- });
- } else {
- return false;
- }
- }
- });
fetch.php
This is our PHP code in fetching data from our database to show in our table.- <?php
- include('conn.php');
- $query = $conn->query("select * from member");
- if ($query->num_rows > 0) {
- while ($row = $query->fetch_array()) {
- $output[] = $row;
- }
- }
- ?>
action.php
This contains our insert and update PHP code.- <?php
- include('conn.php');
- $btn_name = $info->btnName;
- if ($btn_name == "Save") {
- if ($conn->query("insert into member (firstname, lastname) values ('$firstname', '$lastname')")) {
- echo "Member Added Successfully";
- } else {
- echo 'Failed';
- }
- }
- if ($btn_name == "Update") {
- $id = $info->memberid;
- if ($conn->query("update member set firstname='$firstname', lastname='$lastname' where memberid='$id'")) {
- echo 'Member Updated Successfully';
- } else {
- echo 'Failed';
- }
- }
- }
- ?>
delete.php
Lastly, this is our PHP delete code.- <?php
- include('conn.php');
- $id = $data->memberid;
- if ($conn->query("delete from member where memberid='$id'")) {
- echo 'Member Deleted Successfully';
- } else {
- echo 'Failed';
- }
- }
- ?>
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.