Selecting MySQL Table Using Datatable in PHP
Submitted by nurhodelta_17 on Monday, August 21, 2017 - 22:48.
Language
This tutorial will show you how to select mysql table with the use of data table. Datatable is an organize presentation on database table. It is often used in websites and php program because it has an in-built function like the search and pagination.
Creating our Database
First, we're going to create a database that contains the user data. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as "datatable". 3. After creating a database, click the SQL and paste the below code. See image below for detailed instruction.- CREATE TABLE `user` (
- `userid` INT(11) NOT NULL AUTO_INCREMENT,
- `firstname` VARCHAR(30) NOT NULL,
- `lastname` VARCHAR(30) NOT NULL,
- `address` VARCHAR(150) NOT NULL,
- `birthdate` DATE NOT NULL,
- PRIMARY KEY (`userid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
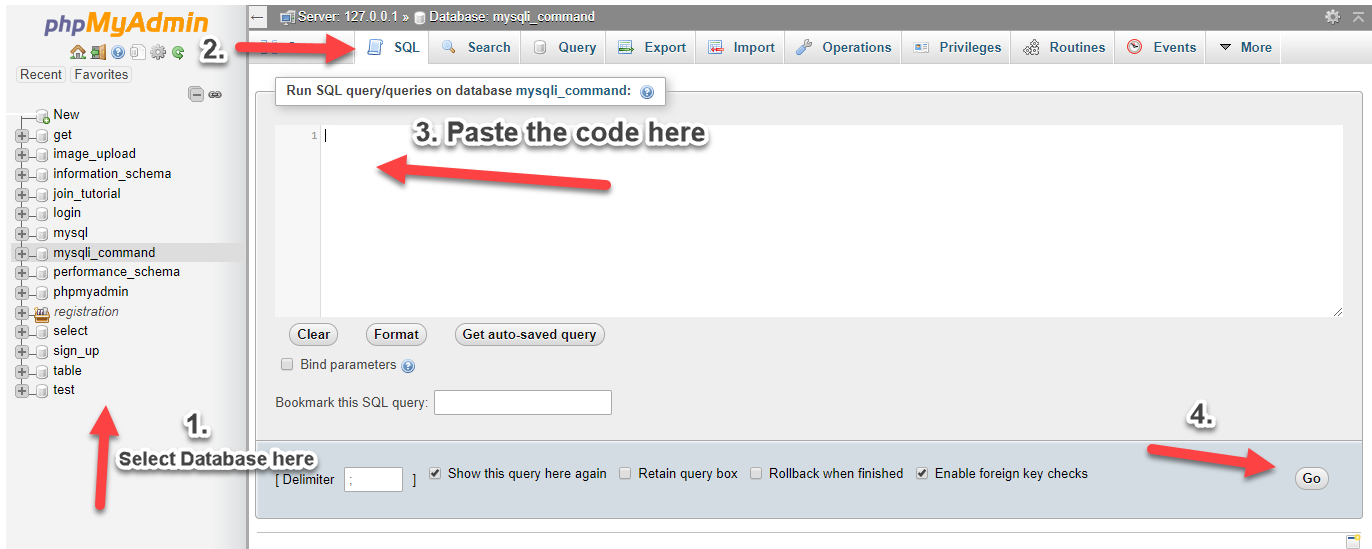
Inserting Data into our Database
Next, we insert data into our database. These data will show as we select our table using datatable. 1. Click our database "datatable". 2. Click SQL and paste the below code.- INSERT INTO `user` (`firstname`, `lastname`, `address`, `birthdate`) VALUES
- ('neovic', 'devierte', 'silay city', '1992-06-23'),
- ('lee', 'ann', 'silay city', '1990-01-01'),
- ('dee', 'dee', 'talisay city', '1996-08-09'),
- ('julyn', 'divinagracia', 'bacolod city', '1992-04-09');
Creating our Connection
Next step is to create a database connection and save it as "conn.php". This file will serve as our bridge between our page and our database. To create the file, open your HTML code editor and paste the code below after the tag.- <?php
- // Check connection
- {
- }
- ?>
Creating our Table
Lastly, we create our table using the datatable. We save this as "index.php". To create the table, open your HTML code editor and paste the code below after the tag.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <link href="bootstrap.min.css" rel="stylesheet">
- <link href="dataTables.bootstrap.css" rel="stylesheet">
- <link href="dataTables.responsive.css" rel="stylesheet">
- <style>
- .mytable{
- margin-left:50px;
- margin-top:30px;
- width:1000px;
- }
- </style>
- </head>
- <body>
- <br>
- <div class="mytable">
- <table width="100%" class="table table-striped table-bordered table-hover" id="dataTables-example">
- <thead>
- <tr>
- </tr>
- </thead>
- <tbody>
- <?php
- include('conn.php');
- $query=mysqli_query($conn,"select * from `user`");
- while($row=mysqli_fetch_array($query)){
- ?>
- <tr>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- </div>
- <script>
- $(document).ready(function() {
- $('#dataTables-example').DataTable({
- responsive: true
- });
- });
- </script>
- </body>
- </html>
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Add new comment
- 1473 views