Easy and Simple Edit/Update MySQL Table using PHP
Submitted by nurhodelta_17 on Monday, August 21, 2017 - 23:20.
Language
This tutorial will show and give you knowledge on how to edit/update MySQL table using PHP. Is this tutorial, I'm gonna show you how to edit using 3 methods namely MySQLi Object-oriented, MySQLi Procedural and PDO.
Creating our Database
First, we're going to create a database that contains our data. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as "edit". 3. After creating a database, click the SQL and paste the below code. See image below for detailed instruction.- CREATE TABLE `user` (
- `userid` INT(11) NOT NULL AUTO_INCREMENT,
- `firstname` VARCHAR(30) NOT NULL,
- `lastname` VARCHAR(30) NOT NULL,
- `edit_via` VARCHAR(60) NOT NULL,
- PRIMARY KEY (`userid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
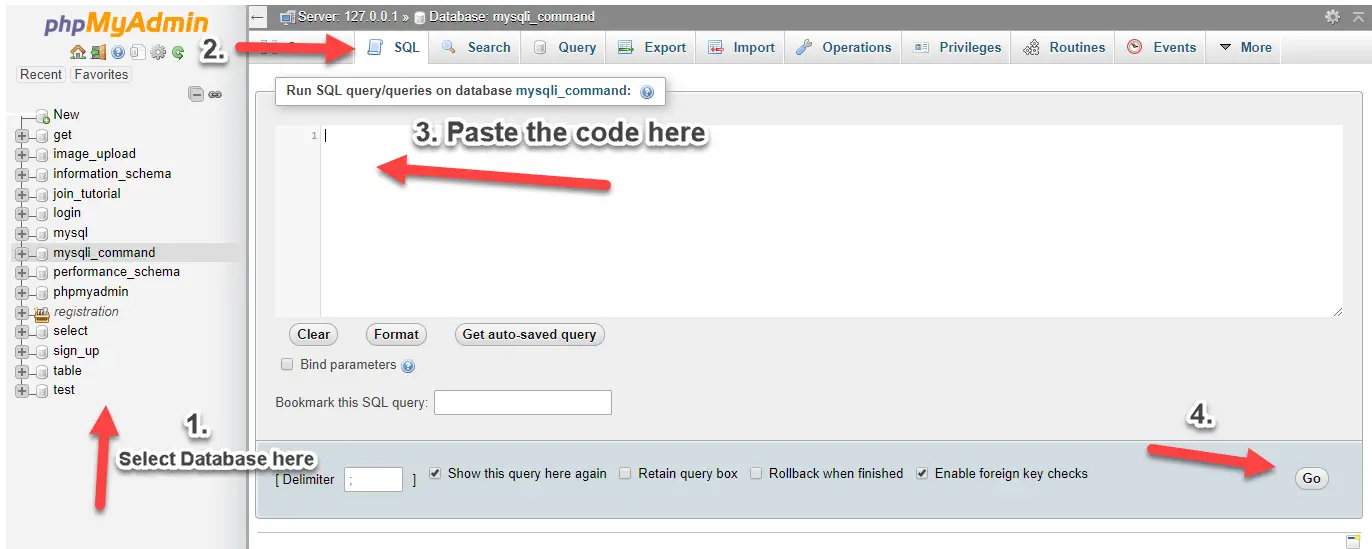
Inserting Data into our Table
Next step is to insert data into our table. This will serve as our reference in editing our rows. 1. Click our database "edit". 2. Click SQL and paste the below code.- INSERT INTO `user` (`firstname`, `lastname`) VALUES
- ('neovic', 'devierte'),
- ('lee', 'ann');
Creating Our Table
Next is to create our table. This table will give us information about the data in our database. We name this table as "index.php". To create the table, open your HTML code editor and paste the code below after the tag.- <!DOCTYPE html>
- <html>
- <head>
- </head>
- <body>
- <div>
- <table border="1">
- <thead>
- </thead>
- <tbody>
- <?php
- $con = mysqli_connect("localhost","root","","edit");
- // Check connection
- if (mysqli_connect_errno())
- {
- echo "Failed to connect to MySQL: " . mysqli_connect_error();
- }
- $query=mysqli_query($con,"select * from `user`");
- while($row=mysqli_fetch_array($query)){
- ?>
- <tr>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- </div>
- </body>
- </html>
Creating our Edit Form and Script
Last step is to create our edit form and our edit script. This will update our selected row in our MySQL Table using the 3 methods. We name this page as "edit.php". To create the page, open your HTML code editor and paste the code below after the tag.- <?php
- $id=$_GET['id'];
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- </head>
- <body>
- <?php
- $conn = mysqli_connect("localhost","root","","edit");
- // Check connection
- if (mysqli_connect_errno())
- {
- echo "Failed to connect to MySQL: " . mysqli_connect_error();
- }
- $query=mysqli_query($conn,"select * from `user` where userid='$id'");
- $row=mysqli_fetch_assoc($query);
- ?>
- <form method="POST">
- <input type="submit" value="MySQLi Object-oriented" name="mysqli_oop">
- <input type="submit" value="MySQLi Procedural" name="mysqli_procedural">
- <input type="submit" value="PDO" name="pdo">
- </form>
- <br>
- <?php
- if (isset($_POST['mysqli_oop'])){
- $firstname=$_POST['firstname'];
- $lastname=$_POST['lastname'];
- // Create connection
- $conn = new mysqli("localhost", "root", "", "edit");
- // Check connection
- if ($conn->connect_error) {
- die("Connection failed: " . $conn->connect_error);
- }
- $sql = "UPDATE `user` SET firstname='$firstname', lastname='$lastname', edit_via='MySQLi Object-oriented' WHERE userid='$id'";
- if ($conn->query($sql) === TRUE) {
- echo "Record updated successfully";
- } else {
- echo "Error updating record: " . $conn->error;
- }
- $conn->close();
- }
- elseif (isset($_POST['mysqli_procedural'])){
- $firstname=$_POST['firstname'];
- $lastname=$_POST['lastname'];
- // Create connection
- $conn = mysqli_connect("localhost", "root", "", "edit");
- // Check connection
- if (!$conn) {
- die("Connection failed: " . mysqli_connect_error());
- }
- $sql = "UPDATE `user` SET firstname='$firstname', lastname='$lastname', edit_via='MySQLi Procedural' WHERE userid='$id'";
- if (mysqli_query($conn, $sql)) {
- echo "Record updated successfully";
- } else {
- echo "Error updating record: " . mysqli_error($conn);
- }
- mysqli_close($conn);
- }
- elseif (isset($_POST['pdo'])){
- $servername = "localhost";
- $username = "root";
- $password = "";
- $dbname = "edit";
- try {
- $conn = new PDO("mysql:host=$servername;dbname=$dbname", $username, $password);
- // set the PDO error mode to exception
- $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- $firstname=$_POST['firstname'];
- $lastname=$_POST['lastname'];
- $sql = "UPDATE `user` SET firstname='$firstname', lastname='$lastname', edit_via='PDO' WHERE userid='$id'";
- // Prepare statement
- $stmt = $conn->prepare($sql);
- // execute the query
- $stmt->execute();
- // echo a message to say the UPDATE succeeded
- echo $stmt->rowCount() . " records UPDATED successfully";
- }
- catch(PDOException $e)
- {
- echo $sql . "<br>" . $e->getMessage();
- }
- $conn = null;
- }
- ?>
- </body>
- </html>
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.