How to Create Login and Logout Page with Session and Cookies in PHP
In my previous tutorial, I've shown you How to Set up Cookie. This tutorial will give you an idea of how to use the stored cookie to login and I've added a "logout" function that destroys both session and cookie.
Creating our Database
First, we're going to create a database that contains our data.
- Open phpMyAdmin.
- Click databases, create a database and name it as "cookie".
- After creating a database, click the SQL and paste the below code. See image below for detailed instruction.
- CREATE TABLE `user` (
- `userid` INT(11) NOT NULL AUTO_INCREMENT,
- `username` VARCHAR(30) NOT NULL,
- `password` VARCHAR(30) NOT NULL,
- `fullname` VARCHAR(60) NOT NULL,
- PRIMARY KEY (`userid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
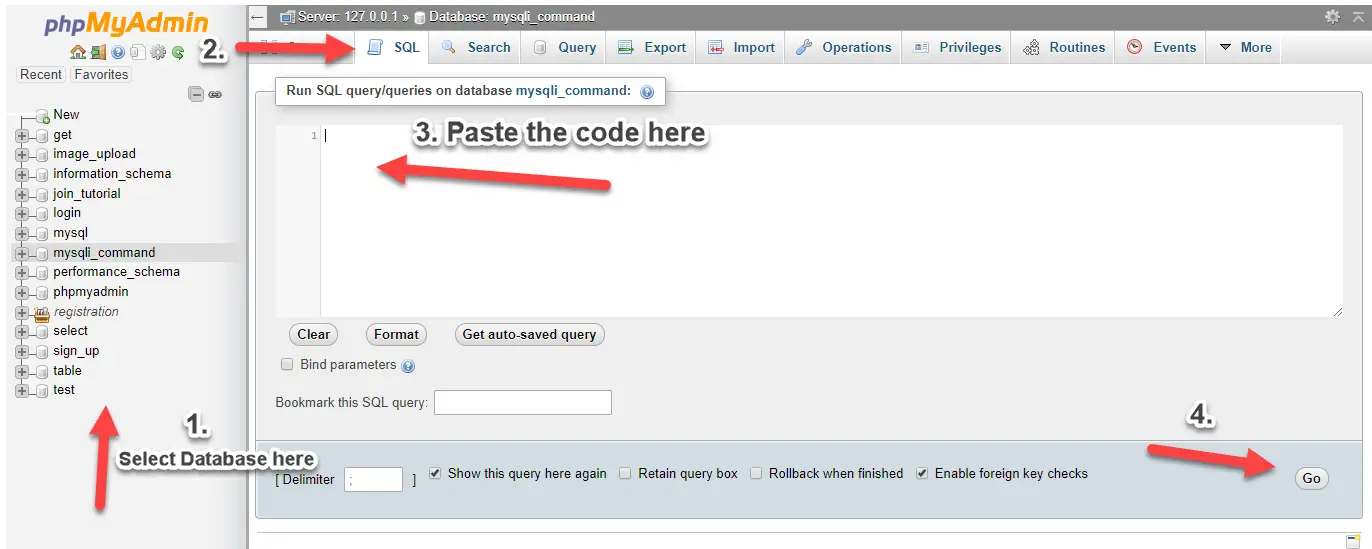
Inserting Data into our Database
Next, we insert users into our database. This will be our reference when we log in.
- Click the database we created earlier.
- Click SQL and paste the code below.
- INSERT INTO `user` (`username`, `password`, `fullname`) VALUES
- ('neovic', 'devierte', 'neovic devierte'),
- ('lee', 'ann', 'lee ann');
Creating our Connection
The next step is to create a database connection and save it as "conn.php"
. This file will serve as our bridge between our form and our database. To create the file, open your HTML code editor and paste the code below after the tag.
- <?php
- // Check connection
- {
- }
- ?>
Creating our Login Form
Next is to create our login form. In this form, I've added a code that if ever there's a cookie stored, it will show in the login inputs. To create the form, open your HTML code editor and paste the code below after the tag. We name this"index.php"
.
- <?php
- include('conn.php');
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>Login Using Cookie with Logout</title>
- </head>
- <body>
- <h2>Login Form</h2>
- <form method="POST" action="login.php">
- <label>Username:</label> <input type="text" value="<?php if (isset($_COOKIE["user"])){echo $_COOKIE["user"];}?>" name="username">
- <label>Password:</label> <input type="password" value="<?php if (isset($_COOKIE["pass"])){echo $_COOKIE["pass"];}?>" name="password"><br><br>
- <input type="submit" value="Login" name="login">
- </form>
- <span>
- <?php
- echo $_SESSION['message'];
- }
- ?>
- </span>
- </body>
- </html>
Creating our Login Script
The next step is creating our login script. We name this script as "login.php"
.
- <?php
- include('conn.php');
- $username=$_POST['username'];
- $password=$_POST['password'];
- $query=mysqli_query($conn,"select * from `user` where username='$username' && password='$password'");
- $_SESSION['message']="Login Failed. User not Found!";
- }
- else{
- //set up cookie
- }
- $_SESSION['id']=$row['userid'];
- }
- }
- else{
- $_SESSION['message']="Please Login!";
- }
- ?>
Creating our Login Success Page
Next, we create a go-to page if login is successful. This page will show the user that login successfully. We name this page as "success.php"
. On this page, I've added the logout link to destroy both the session and cookie.
- <?php
- }
- include('conn.php');
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>Setting Up Cookie on User Login</title>
- </head>
- <body>
- <h2>Login Success</h2>
- <?php echo $row['fullname']; ?>
- <br>
- <a href="logout.php">Logout</a>
- </body>
- </html>
Creating our Logout Script
Last is our logout script. This script destroys both our session and the cookie then redirects us back to our login page. We name the script as "logout.php"
.
- <?php
- }
- ?>
And that's the end of this tutorial. I hope this will help you and for your future projects. Feel free to download the working sample source code and test it.
Explore more on this website for tutorials and free source code.
Enjoy :)Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Comments
Add new comment
- Add new comment
- 21972 views