Adding and Saving Records to Access Database using VB.NET Tutorial
Submitted by donbermoy on Wednesday, December 2, 2020 - 10:08.
This is a continuation of my other tutorials entitled Search and Retrieve a Record from Access Database and VB.NET. But here, we will focus on adding and saving a record into an access database.
Now, let's start this tutorial!
1. Create an access file with the table and entities like the image below. Name your access database as sample.mdb(2003 format).
2. Create a Windows Form Application in VB.NET for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
3. Next, add another Button for adding records (Assume that we are done with the search button here as you will open the link I have write above.) named btnAdd and labeled it as "Add". This will add and save the inputted texts in all our textboxes. Insert 4 textbox named txtSearch for the searching of student number as this is the primary key, txtName for Student Name, txtCourse for Student course, and txtSection for student section. You must design your interface like this:
4. Create a module in your project and named it, modConnection.
Import
Now, create a method named connection to have the connection string. This will locate the sample.mdb access database that we have created earlier.
Full code of the modConnection module.
5. Back to our form, put this code for the form_load. We will call the connetion() method that we have created in our module. Because we all know that module is access throughout the entire program.
6. For the btnAdd as adding and saving records to database button, put this code below.
We will first create a try and catch method to have the exception handling. Here, we will use the OleDbCommand with the method of connection, CommandType.Text, and CommandText, with the OleDbDataReader to execute the CommandText. Note: The sql syntax "INSERT INTO tblStudent (Snum,Sname,Scourse,Ssection) VALUES (@Snum,@Sname,@Scourse,@Ssection)" is for adding and saving of records to the database. Snum,Sname,Scourse, and Ssection are our entities and @Snum,@Sname,@Scourse,@Ssection are our parameters.
Now, have your parameters hold the value inputted in your textboxes that you have created earlier in the interface.
To run the command of our parameters put this code below.
Execute the non query and have a message of "Record Saved" after execution.
Clear all the textboxes after saving the records. And don't forget the Exit Sub command at the end of your Try method. This will terminate the sub process of trying to add again another record.
Then to catch the error, put this in your catch method.
Here is the full source code of our tutorial for adding and saving records with search also.
Output:
The database is located inside the Debug folder of Bin.
Best Regards,
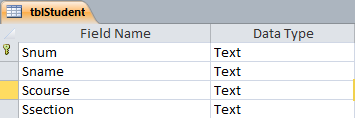
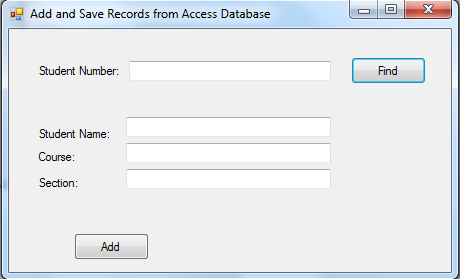
Imports System.Data.OleDb library
. This library package is for ms access database.
In your module connection, initialize the following variables.
- Module modConnection
- Public cn As New OleDb.OleDbConnection
- Public cm As New OleDb.OleDbCommand
- Public dr As OleDbDataReader
- Public Sub connection()
- cn = New OleDb.OleDbConnection
- With cn
- 'Provider must be Microsoft.Jet.OLEDB.4.0, find the access file, and test the connection
- .ConnectionString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" & Application.StartupPath & "\sample.mdb"
- .Open()
- End With
- End Sub
- Imports System.Data.OleDb
- Module modConnection
- Public cn As New OleDb.OleDbConnection
- Public cm As New OleDb.OleDbCommand
- Public dr As OleDbDataReader
- Public Sub connection()
- cn = New OleDb.OleDbConnection
- With cn
- .ConnectionString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" & Application.StartupPath & "\sample.mdb"
- .Open()
- End With
- End Sub
- End Module
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- Call connection()
- End Sub
- Try
- cm = New OleDb.OleDbCommand
- With cm
- .Connection = cn
- .CommandType = CommandType.Text
- .CommandText = "INSERT INTO tblStudent (Snum,Sname,Scourse,Ssection) VALUES (@Snum,@Sname,@Scourse,@Ssection)"
- .Parameters.Add(New System.Data.OleDb.OleDbParameter("@Snum", System.Data.OleDb.OleDbType.VarChar, 255, Me.txtSearch.Text))
- .Parameters.Add(New System.Data.OleDb.OleDbParameter("@Sname", System.Data.OleDb.OleDbType.VarChar, 255, Me.txtName.Text))
- .Parameters.Add(New System.Data.OleDb.OleDbParameter("@Scourse", System.Data.OleDb.OleDbType.VarChar, 255, Me.txtCourse.Text))
- .Parameters.Add(New System.Data.OleDb.OleDbParameter("@Ssection", System.Data.OleDb.OleDbType.VarChar, 255, Me.txtSection.Text))
- cm.Parameters("@Snum").Value = Me.txtSearch.Text
- cm.Parameters("@Sname").Value = Me.txtName.Text
- cm.Parameters("@Scourse").Value = Me.txtCourse.Text
- cm.Parameters("@Ssection").Value = Me.txtSection.Text
- cm.ExecuteNonQuery()
- Me.txtCourse.Text = ""
- Me.txtName.Text = ""
- Me.txtSearch.Text = ""
- Me.txtSection.Text = ""
- Exit Sub
- Catch ex As Exception
- End Try
- Public Class Form1
- Private Sub btnFind_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnFind.Click
- Dim found As Boolean
- Try
- cm = New OleDb.OleDbCommand
- With cm
- .Connection = cn
- .CommandType = CommandType.Text
- .CommandText = "SELECT * FROM tblStudent WHERE (Snum = '" & txtSearch.Text & "')"
- dr = .ExecuteReader
- End With
- While dr.Read()
- txtName.Text = dr("Sname").ToString
- txtCourse.Text = dr("Scourse").ToString
- txtSection.Text = dr("Ssection").ToString
- found = True
- End While
- Exit Sub
- Catch ex As Exception
- End Try
- End Sub
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- Call connection()
- End Sub
- Private Sub btnAdd_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnAdd.Click
- Try
- cm = New OleDb.OleDbCommand
- With cm
- .Connection = cn
- .CommandType = CommandType.Text
- .CommandText = "INSERT INTO tblStudent (Snum,Sname,Scourse,Ssection) VALUES (@Snum,@Sname,@Scourse,@Ssection)"
- .Parameters.Add(New System.Data.OleDb.OleDbParameter("@Snum", System.Data.OleDb.OleDbType.VarChar, 255, Me.txtSearch.Text))
- .Parameters.Add(New System.Data.OleDb.OleDbParameter("@Sname", System.Data.OleDb.OleDbType.VarChar, 255, Me.txtName.Text))
- .Parameters.Add(New System.Data.OleDb.OleDbParameter("@Scourse", System.Data.OleDb.OleDbType.VarChar, 255, Me.txtCourse.Text))
- .Parameters.Add(New System.Data.OleDb.OleDbParameter("@Ssection", System.Data.OleDb.OleDbType.VarChar, 255, Me.txtSection.Text))
- ' RUN THE COMMAND
- cm.Parameters("@Snum").Value = Me.txtSearch.Text
- cm.Parameters("@Sname").Value = Me.txtName.Text
- cm.Parameters("@Scourse").Value = Me.txtCourse.Text
- cm.Parameters("@Ssection").Value = Me.txtSection.Text
- cm.ExecuteNonQuery()
- Me.txtCourse.Text = ""
- Me.txtName.Text = ""
- Me.txtSearch.Text = ""
- Me.txtSection.Text = ""
- Exit Sub
- End With
- Catch ex As Exception
- End Try
- End Sub
- End Class
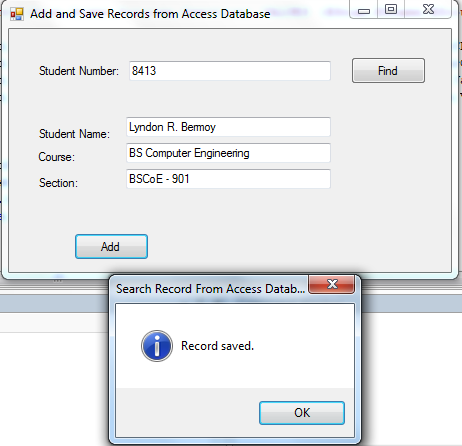

Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz
Comments
Error
i trying to add my text box data to ms access database using vb2010 , i connected my database with vb using connection wizard . but when i add data to databse first set of data is corectily savied in database but net time i try to add new data to database it showing error
pls help me to corect the same
here iam attaching the code and the error msg
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
'TODO: This line of code loads data into the 'Masterdata_mdbDataSet.Machine' table. You can move, or remove it, as needed.
Me.MachineTableAdapter.Fill(Me.Masterdata_mdbDataSet.Machine)
End Sub
Private Sub Add_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Add.Click
MachineBindingSource.AddNew()
End Sub
Private Sub Save_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Save.Click
MachineBindingSource.EndEdit()
MachineTableAdapter.Update(Masterdata_mdbDataSet.Machine)
End Sub
Msg is Concurrency violation: the UpdateCommand affected 0 of the expected 1 records.
Save and upload code
I wrote this code but ut show an error message ("ExecuteNonQuery requires an open and available connection.The connection's current state is closed")
everything went well expect
everything went well expect if i search for the student and then want to search for another student it wouldnt change the info.
also i have another question how can i share the database in local network? for example i have 5 pcs ill install the program on all of them but i want them to save in the same database
please advise
Call connection()
copy Call connection() on form load and past it on the button that execute the search records. the problem is that the collection has closed after executing the first command.
Why is this error" Microsoft
Why is this error" Microsoft.Jet.OLEDB.4.0" keep showing even though i follow every steps? Can you fix it?
Cause the database your
Cause the database your updating is on debug, it reset the database whenever you finish you running your code so user will be easily to experiment on the database. If you want to permanent save it, use the database file outside debug. but its a hassle if you want to delete some data every time you try to run the program
How to update database
Hi, can you also give us a code for updating database using also parameterized query? Its just doesn' work for me, I mean, its working but the database doesn't update at all
Thanks for sharing such huge…
Thanks for sharing such huge content keep posting further.... Feel free to visit our website ePub Conversion Services
Connection is closed or open
Its nice that it uses parameter in adding Data to a Database but you need to fix the connection error
Thanks for this content
hey there, Get Updated with whats going around with The Blog 101.
Find out to determine Client Life time Worth utilizing DCF & inn
Find out to determine Client Life time Worth utilizing DCF & & innovative designs & & utilize it to boost the business’s productivity
What you will certainly discover
Find out to determine client’s life time worth under various circumstances and also utilize it to boost the business’s productivity.
Integrate the effect of price cut price and also retention price to determine client worth
Run Monte-Carlo Simulation to design uncertainity in Market
This is really an interesting blog.Keep sharing i really appreciate the work
THOSE WHO HAVE PROBLEM IN…
THOSE WHO HAVE PROBLEM IN Microsoft.Jet.OLEDB.4.0' provider is not registered on the local machine, JUST CHANGE 4.0 TO 12.0