Bar Chart using ChartJS, AngularJS and PHP/MySQLi
Submitted by nurhodelta_17 on Thursday, January 18, 2018 - 15:04.
Getting Started
I've used CDN for Bootstrap, Angular JS and Chart JS so you need internet connection for them to work.Creating our Database
First, we're going to create our MySQL Database where we fetch data to supply to our chart. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as angular. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.- (17, 50, '2018-01-01 16:00:00'),
- (18, 600, '2018-02-01 16:00:00'),
- (19, 50, '2018-02-04 16:00:00'),
- (20, 700, '2018-03-02 16:00:00'),
- (21, 350, '2018-04-03 16:00:00'),
- (22, 100, '2018-05-04 16:00:00'),
- (23, 650, '2018-06-05 16:00:00'),
- (24, 250, '2018-07-06 16:00:00'),
- (25, 200, '2018-08-07 16:00:00'),
- (26, 450, '2018-09-08 16:00:00'),
- (27, 750, '2018-10-09 16:00:00'),
- (28, 800, '2018-11-10 16:00:00'),
- (29, 150, '2018-12-11 16:00:00'),
- (30, 50, '2018-01-06 16:00:00'),
- (31, 50, '2018-01-16 16:00:00'),
- (32, 40, '2018-01-01 16:00:00'),
- (33, 10, '2018-01-21 16:00:00');
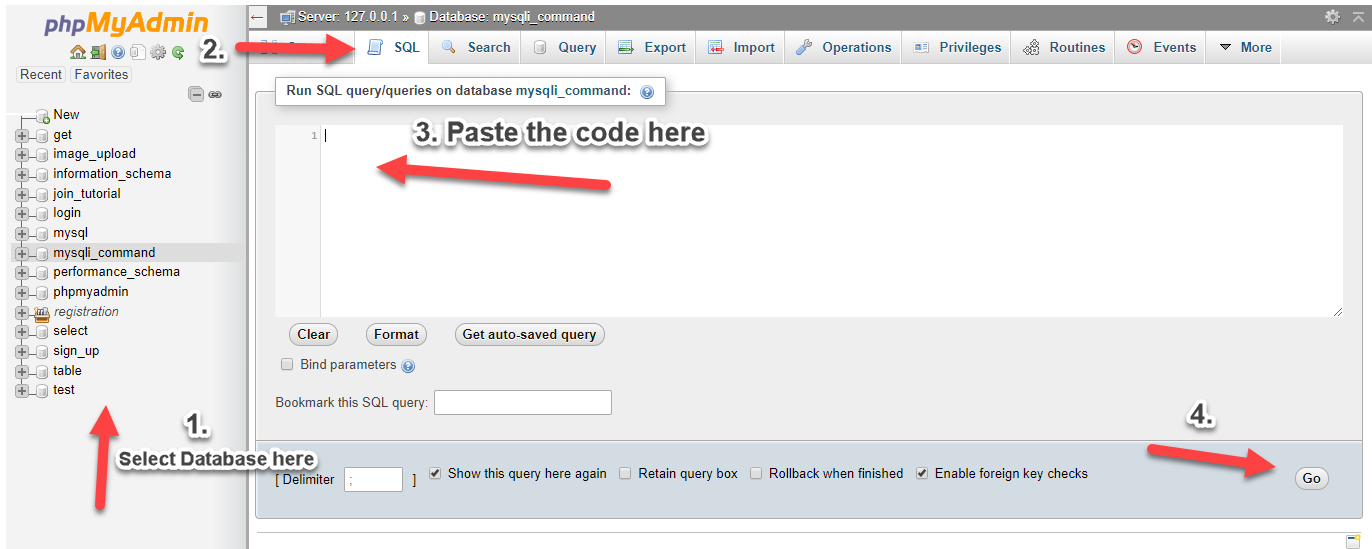
index.html
This is our index which contains our add data form as well as our chart.- <!DOCTYPE html>
- <html ng-app="app">
- <head>
- <meta charset="utf-8">
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- <style type="text/css">
- canvas{
- margin:auto;
- }
- .alert{
- margin-top:20px;
- }
- </style>
- </head>
- <body ng-controller="myCtrl">
- <div class="container">
- <div class="row">
- <div class="col-sm-3 col-md-offset-1" ng-init="fetchfruit()">
- <div class="form-group">
- <input type="text" class="form-control" ng-model="buy.amount">
- </div>
- <div class="form-group">
- <input type="date" class="form-control" ng-model="buy.date">
- </div>
- <div class="alert alert-success text-center" ng-show="success">
- {{ message }}
- </div>
- <div class="alert alert-danger text-center" ng-show="error">
- {{ message }}
- </div>
- </div>
- <div class="col-sm-7" ng-init="fetchsales()">
- <canvas id="dvCanvas" height="200" width="300"></canvas>
- </div>
- </div>
- </div>
- </body>
- </html>
app.js
This contains our angular js scripts.- var app = angular.module('app', []);
- app.controller('myCtrl', function ($scope, $http) {
- $scope.error = false;
- $scope.success = false;
- $scope.purchase = function(){
- $http.post('purchase.php', $scope.buy)
- .success(function(data){
- if(data.error){
- $scope.error = true;
- $scope.success = false;
- $scope.message = data.message;
- }
- else{
- $scope.success = true;
- $scope.error = false;
- $scope.message = data.message;
- $scope.fetchsales();
- $scope.buy = '';
- }
- });
- }
- //this fetches the data for our table
- $scope.fetchsales = function(){
- $http.get('fetchsales.php').success(function(data){
- var ctx = document.getElementById("dvCanvas").getContext('2d');
- var myChart = new Chart(ctx, {
- type: 'bar',
- data: {
- labels: ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"],
- datasets: [{
- label: 'Total Purchase',
- data: data,
- borderWidth: 1
- }]
- },
- options: {
- scales: {
- yAxes: [{
- ticks: {
- beginAtZero:true
- }
- }]
- }
- }
- });
- });
- }
- $scope.clear = function(){
- $scope.error = false;
- $scope.success = false;
- }
- });
purchase.php
This is our PHP api/code in adding new data into our MySQL Database.- <?php
- $conn = new mysqli("localhost", "root", "", "angular");
- $amount = $data->amount;
- $sql = "INSERT INTO sales (amount, sale_date) VALUES ('$amount', '$date')";
- $query = $conn->query($sql);
- if($query){
- $out['message'] = "Purchase added successfully";
- }
- else{
- $out['error'] = true;
- $out['message'] = "Cannot add purchase";
- }
- ?>
fetchsales.php
Lastly, this is our PHP api that fetches data from our database to use in our graph.- <?php
- $conn = new mysqli("localhost", "root", "", "angular");
- //set timezone
- //date_default_timezone_set('Asia/Manila');
- for ($month = 1; $month <= 12; $month ++){
- $sql="SELECT sum(amount) AS total FROM sales WHERE month(sale_date)='$month' AND year(sale_date)='$year'";
- $query=$conn->query($sql);
- $row=$query->fetch_array();
- $out[]=$row['total'];
- }
- ?>
Add new comment
- 605 views