In this tutorial I will show you how to create a
Login User and
User Registration Form using
Visual Basic 2008 and
MySQL Database. In this features, you can
Register and
Login what you’ve
Registered in the
User Registration Form.
Login Form is very
Important in making a
System because this serves as a
Protection in your
System from
Invaders. It will also filter the
Users on what
functionalities they are going to use.
So lets’ begin:
First, create a table in the
MySQL Database named
“userdb”.
After that, create a
Registration and
Login in a
Form, and it will look like this.
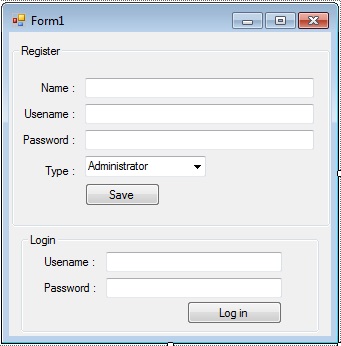
Then double click the
Form and set up the connection of
MySQL Database and
Visual Basic 2008 in global.
Imports MySql.Data.MySqlClient
Public Class Form1
'create a public function and set your MySQL Connection .
Public Function mysqlconnection() As MySqlConnection
'return new connection.
Return New MySqlConnection("server=localhost;user id=root;database=userdb")
End Function
'put the value of mysqlconnection() as MySQL connection to con.
Public con As MySqlConnection = mysqlconnection()
End Class
Declare all the
Classes that you needed.
'declaring the classes
'a set of command in MySQL
Dim cmd As New MySqlCommand
'represent a new spicific table in the database
Public dt As New DataTable
'serve as the bridge of mysql database and the datatable for saving and retrieving data.
Public da As New MySqlDataAdapter
Create a
Sub Procedure for
Registration.
'create a sub procedure named "create" and the parameter's type is string
Public Sub create(ByVal sql As String)
Try
'open the connection
con.Open()
'set your command
With cmd
'holds the data to be executed
.Connection = con
.CommandText = sql
'execute the data
result = cmd.ExecuteNonQuery
'coditioning the result whether succesfull or failed when it is executed.
If result = 0 Then
'the execution of data is failed
MsgBox("Registration failed!")
Else
'the executed data is succesfull
MsgBox("You are now registered.")
End If
End With
Catch ex As Exception
End Try
End Sub
Create a
Sub Procedure for
login.
'create a sub procedure named "loginUser" and the parameter's type is string
Public Sub loginUser(ByVal sql As String)
Try
'declaring the variable as integer
Dim maxrow As Integer
'open the connection
con.Open()
'it will set a new specific table in the database
dt = New DataTable
'set a command
With cmd
'holds the data
.Connection = con
.CommandText = sql
End With
'retriving the data
da.SelectCommand = cmd
da.Fill(dt)
'put the max value of rows in the table to a variable(maxrow)
maxrow = dt.Rows.Count
'conditioning the total value of rows in the table
'if the total value of rows in the table is greater than 0 then the result is true
' and if not, the result is false
If maxrow > 0 Then
'appearing the record of the current row and the current column in the current table.
MsgBox(dt
.Rows(0).Item(1) & " , " & dt
.Rows(0).Item(4))
Else
'the result is false
MsgBox("Account does not exist.")
End If
Catch ex As Exception
End Try
da.Dispose()
End Sub
Go back to
Design Views, double click the
Save Button and do this.
Private Sub btnsave_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnsave.Click
'put the string value to sql
sql = "INSERT INTO `users` (`name`, `username`, `Pass`, `type` ) VALUES ('" _
& txtname.Text & "','" & txtuser.Text & "','" & txtpass.Text & "','" & cbotype.Text & "')"
'call your sub name and put the sql in the parameters list.
create(sql)
End Sub
Go back to
Design Views, double click the
Login Button and do this.
Private Sub btnlogin_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnlogin.Click
'put the string value to sql
sql = "SELECT * from users WHERE username = '" & username.Text & "' and Pass = '" & password.Text & "'"
'call your sub name and put the sql in the parameters list.
loginUser(sql)
End Sub
Run your project.
The complete Source Code is included. Download and run it on your computer.