Create XML File in VB.NET
Submitted by donbermoy on Monday, July 14, 2014 - 10:12.
Today in VB.NET, I’m going to teach you how to create a program that also creates an XML file using VB.NET. We all know that XML (Extensible Markup Language) is an easy way to create common information formats and share both the format and the data on the World Wide Web or elsewhere.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in VB.NET for this tutorial by following the following steps in Microsoft Visual Studio 2010: Go to File, click New Project, and choose Windows Application and name your program as XMLSample.
2. Next, add only one Button named Button1 and labeled it as "Create XML". You must design your interface like this:
3. Import System.Xml namespace to access all the xml class.
4. Create a method named createNode that has this parameters (string pID, string pName, string pPrice, XmlTextWriter writer). The XmlTextWriter class here is used to write xml file.
5. For Button1 for writing an XML File, put this code below.
Full source code:
Output:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
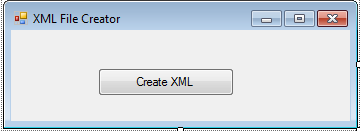
- Imports System.Xml
- Private Sub createNode(ByVal pID As String, ByVal pName As String, ByVal pPrice As String, ByVal writer As XmlTextWriter)
- 'write Product as a Start Element
- writer.WriteStartElement("Product")
- 'write Product_id as a Start Element as a content of Product
- writer.WriteStartElement("Product_id")
- 'write a string for Product_id
- writer.WriteString(pID)
- ' end the element of Product_id using /
- writer.WriteEndElement()
- 'write Product_name as a Start Element as a content of Product
- writer.WriteStartElement("Product_name")
- 'write a string for Product_name
- writer.WriteString(pName)
- 'end the element of Product_name using /
- writer.WriteEndElement()
- 'write Product_price as a Start Element as a content of Product
- writer.WriteStartElement("Product_price")
- ' write a string for Product_price
- writer.WriteString(pPrice)
- 'end the element of Product_price using /
- writer.WriteEndElement()
- 'end the element of Product using /
- writer.WriteEndElement()
- End Sub
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- 'instatantiate XmlTextWriter that the file name of the xml file will be product.xml
- Dim writer As New XmlTextWriter("product.xml", System.Text.Encoding.UTF8)
- 'set WriteStartDocument to true
- writer.WriteStartDocument(True)
- 'the xml formatting has indention
- writer.Formatting = Formatting.Indented
- '2 indentions
- writer.Indentation = 2
- 'Make now the first element into "Table"
- writer.WriteStartElement("Table")
- 'we will use the createNode method with the parameters of product id, product name, product price, and the writer variable for XmlTextWriter
- createNode(1, "Product 1", "1000", writer)
- createNode(2, "Product 2", "2000", writer)
- createNode(3, "Product 3", "3000", writer)
- createNode(4, "Product 4", "4000", writer)
- 'end the element with the word table and using /
- writer.WriteEndElement()
- 'end the file
- writer.WriteEndDocument()
- 'close the file
- End Sub
- Imports System.Xml
- Public Class Form1
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- Dim writer As New XmlTextWriter("product.xml", System.Text.Encoding.UTF8)
- writer.WriteStartDocument(True)
- writer.Formatting = Formatting.Indented
- writer.Indentation = 2
- writer.WriteStartElement("Table")
- createNode(1, "Product 1", "1000", writer)
- createNode(2, "Product 2", "2000", writer)
- createNode(3, "Product 3", "3000", writer)
- createNode(4, "Product 4", "4000", writer)
- writer.WriteEndElement()
- writer.WriteEndDocument()
- End Sub
- Private Sub createNode(ByVal pID As String, ByVal pName As String, ByVal pPrice As String, ByVal writer As XmlTextWriter)
- writer.WriteStartElement("Product")
- writer.WriteStartElement("Product_id")
- writer.WriteString(pID)
- writer.WriteEndElement()
- writer.WriteStartElement("Product_name")
- writer.WriteString(pName)
- writer.WriteEndElement()
- writer.WriteStartElement("Product_price")
- writer.WriteString(pPrice)
- writer.WriteEndElement()
- writer.WriteEndElement()
- End Sub
- End Class
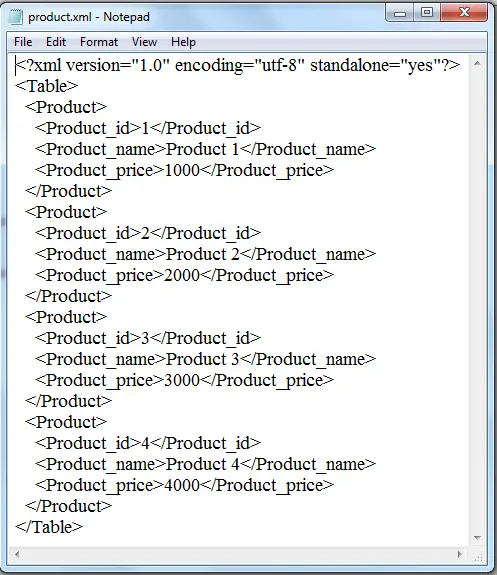