How to Populate Listview with Data from Database using Visual Basic.Net
Submitted by joken on Wednesday, May 14, 2014 - 14:58.
In Windows from application, one of the most choice of a programmer used to display vast amount of data on the screen aside from datagridview is Listview control.Because Listview control provides the infrastructure to display a set of data items in different layouts or views. In this tutorial, i'm going to show you how to populate listview control with data from Microsoft Access database using Visual Basic.Net. to start with let’s a new project in Visual Basic called “Listview”. Then we will add a listview control from toolbox. Next, add a column Header that looks like as shown below.
At this, lets’ create a new Database in Microsoft Access 2007 and we will save it as “studinfo”. Then create a table named “students”. And here’s the design for this table.
And here’s the sample data content for this table which we are going to display it in our listview control later on.
At this time, lets save this database inside the debug folder of our project. Then we will go back to our application. to start adding functionality to our application, double click the form, and add the following declaration outside any “private sub”. And here’s the following code:
As we observe to code above, we have assigned values to variable “con” from a function called “jokendb()” which is returing new set of values containing the provider and the data source of our database. and here’s the following code for this function:
And finally, when the form loads at start up. It will loads all the data from the database to listview control. And here’s the following code:
Then you can run the application and test it, it should look like as shown below:
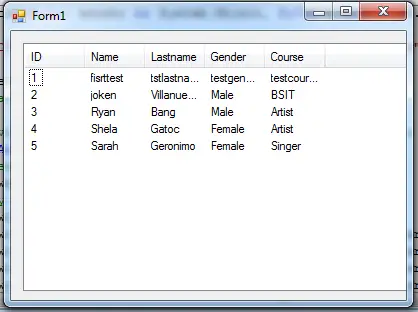
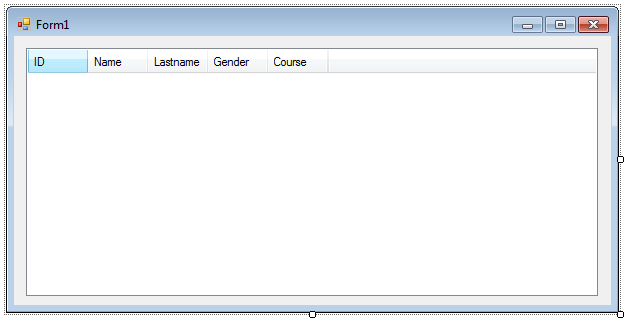
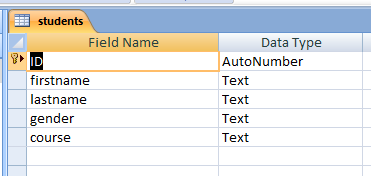
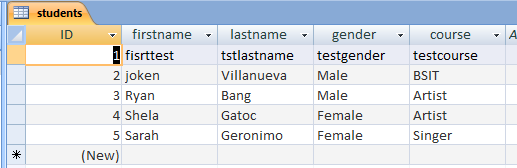
- 'this will serve as an open connection to our data source.
- Public con As OleDb.OleDbConnection = jokendb()
- 'this is some set of commands and a database connection that are use to fill later our datatable
- Dim da As New OleDb.OleDbDataAdapter
- 'this will represent as our table in memory
- Dim dt As New DataTable
- 'this holds our SQL Statements
- Dim sql As String
- Public Function jokendb() As OleDb.OleDbConnection
- Return New OleDb.OleDbConnection("Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" & Application.StartupPath & "\studinfo.accdb")
- End Function
- Try
- sql = "SELECT ID,firstname,lastname,gender,course FROM students"
- con.Open()
- da = New OleDb.OleDbDataAdapter(sql, con)
- 'fill the data to datatable
- da.Fill(dt)
- 'declare a variable as DataRow which is represent a row of data
- Dim newrow As DataRow
- 'loop from each row based on the number of rows stored in dt
- For Each newrow In dt.Rows
- 'display the results in listview
- ListView1.Items.Add(newrow.Item(0)) 'this is for ID
- ListView1.Items(ListView1.Items.Count - 1).SubItems.Add(newrow.Item(1)) 'this is for Name
- ListView1.Items(ListView1.Items.Count - 1).SubItems.Add(newrow.Item(2)) 'this is for Lastname
- ListView1.Items(ListView1.Items.Count - 1).SubItems.Add(newrow.Item(3)) 'this is for gender
- ListView1.Items(ListView1.Items.Count - 1).SubItems.Add(newrow.Item(4)) ' this is for course
- Next
- Catch ex As Exception
- End Try
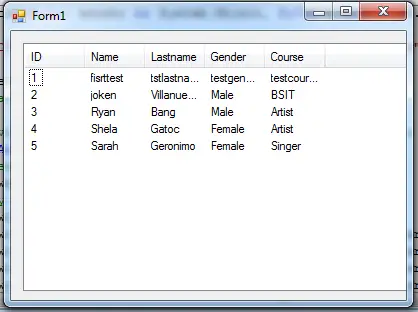
Add new comment
- 2451 views