Sending E-mail with Attachment
Submitted by donbermoy on Wednesday, March 5, 2014 - 09:33.
Last month, I had lectured on how to make a program that can send an e-mail using visual basic.net. Now, we will create a program that can send also e-mails but has an attachment. For this tutorial we will use the System.Net.Mail namespace as it contains classes used for sending e-mails to a Simple Mail Transfer Protocol (SMTP) server for delivery.
Now, let's start this sending an e-mail with attachment tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add three TextBoxes named TextBox1 for inputting the e-mail receiver of the message, TextBox2 for inputting the e-mail used as the source, and TextBox3 to use as the body message of the e-mail. Add a picture box named PictureBox1 and then import an image to the resources so that we can attach this image file in our e-mail. Add also a Button named Button1.You must design your layout like this:
3. Put this code in your topmost part of your code module.
Imports System.Net.Mail - this namespace has all the library of methods for sending an email.
4. Now put add this code for your code module. This code is for Button1_Click:
Following are some commonly used properties of the SmtpClient class:
Property Description
1. ClientCertificates Specifies which certificates should be used to establish the Secure Sockets Layer (SSL) connection.
2. Credentials Gets or sets the credentials used to authenticate the sender.
3. EnableSsl Specifies whether the SmtpClient uses Secure Sockets Layer (SSL) to encrypt the connection.
4. Host Gets or sets the name or IP address of the host used for SMTP transactions.
5. Port Gets or sets the port used for SMTP transactions.
6. Timeout Gets or sets a value that specifies the amount of time after which a synchronous Send call times out.
7. UseDefaultCredentials Gets or sets a Boolean value that controls whether the DefaultCredentials are sent with requests.
The tutorial demonstrates how to send mail using the SmtpClient class. Following points are to be noted in this respect:
• You must specify the SMTP host server that you use to send e-mail. The Host and Port properties will be different for different host server. We will be using gmail server.
• You need to give the Credentials for authentication, if required by the SMTP server
• You should also provide the email address of the sender and the e-mail address or addresses of the recipients using the MailMessage.From and MailMessage.To properties, respectively.
• You should also specify the message content using the MailMessage.Body property.
• The Mail.Attachment here is our main method for attaching a file from our directories in e-mail. Attach the file for you to send the email.
Output:
Download the source code below and try it! :)
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below and hire me.
Best Regards,
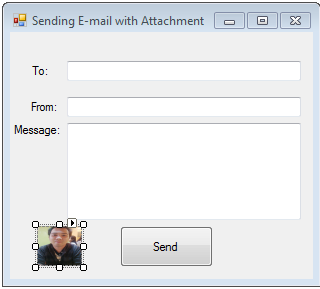
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- Dim mail As New MailMessage()
- Dim SmtpServer As New SmtpClient("smtp.gmail.com")
- mail.[To].Add(TextBox1.Text) 'to_address
- mail.Body = TextBox3.Text ' mail with attachment
- Dim attachment As System.Net.Mail.Attachment
- attachment = New System.Net.Mail.Attachment("C:\Users\don\Desktop\POSTS\post49\bag.o\email with attachment\email with attachment\Resources\1389415093339.jpg")
- mail.Attachments.Add(attachment)
- SmtpServer.Port = 587
- SmtpServer.Credentials = New System.Net.NetworkCredential("username", "password")
- SmtpServer.EnableSsl = True
- SmtpServer.Send(mail)
- MessageBox.Show("mail Send")
Output:
Download the source code below and try it! :)
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below and hire me.
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz