How to Read and Write Text Files in VB.NET
Submitted by donbermoy on Thursday, February 6, 2014 - 14:07.
Hi! this is my other tutorial in VB.Net in how to read and write a file.
In this tutorial, we will learn how to manage data that is stored as a text file. Using text file is an easy way to manage data, although it is not as sophisticated as full fledged database management software such as SQL, Microsoft Access, and Oracle. VB.NET allows the user to create a text file, save the text file as well as read the text file.
Reading and writing to a text file in VB.Net required the use of the StreamReader class and the StreamWriter class respectively. StreamReader is a tool that enables the streaming of data by moving it from one location to another so that it can be read by the user. For example, it allows the user to read a text file that is stored in a hard drive. On the other hand, the StreamWriter class is a tool that can write data input by the use to a storage device such as the hard drive.
To achieve that, first of all we need to include the following statement in the program code:
Now, type the following code below:
When you click the save button, the program will prompt you to key in a file name and the text will be save as a text file. Finally, you can combine the two programs together and create a text editor that can read and write text file. When clicking the save button, this image like this below will be shown. But First type "Hi" in the textbox.
When clicking the save button, it will look like this:
Save the file in a filename "Hi.txt". and then open it by clicking the Open button. This image below is the image after clicking on the Open Button.
and then it will display the contents now of our Hi.txt.
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Imports System.IO
- This line has to precede the whole program code as it is higher in hierarchy than the StreamReader Class. In Fact, this is the concept of object oriented programming where StreamReader is part of the namespace System.IO.
Now, start a new project and name it in whatever name you wish. Now, insert the OpenFileDialog control into the form because we will use it to read the file from the storage device. The default name of the OpenFileDialog control is OpenFileDialog1, you can use this name or you can rename it with a more meaningful name. The OpenFileDialog control will return a DialogResult value which can determine whether the user clicks the OK button or Cancel button . We will also insert a command button and change its displayed text to 'Open'. It will be used by the user to open and read a certain text file.
It will be the same with saving the file, insert the SaveFileDialog control into the form because we will use it to read the file from the storage device. The SaveFileDialog control will return a DialogResult value which can determine whether the user clicks the OK button or Cancel button . We will also insert a command button and change its displayed text to 'Save'. It will be used by the user to save and write a certain text file.
Next, we insert a textbox and set its Multiline property to true. It is used for displaying the text from a text file. In order to read the text file, we need to create a new instant of the streamReader and connect it to a text file with the following statement:
FileReader = New StreamReader(OpenFileDialog1.FileName)
In addition, we need to use the ReadToEnd method to read the entire text of a text file. The syntax is:
TextBox1.Text = FileReader.ReadToEnd()
Your design will be like this one below:
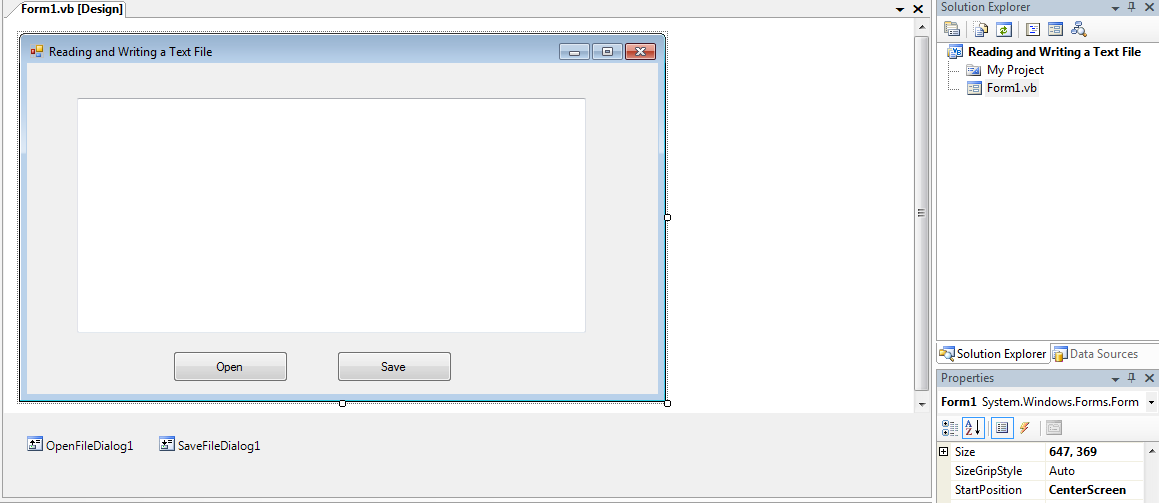
- Imports System.IO
- Public Class Form1
- Private Sub BtnOpen_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles BtnOpen.Click
- Dim FileReader As StreamReader
- Dim results As DialogResult
- results = OpenFileDialog1.ShowDialog
- If results = DialogResult.OK Then
- FileReader = New StreamReader(OpenFileDialog1.FileName)
- TextBox1.Text = FileReader.ReadToEnd()
- End If
- End Sub
- Private Sub btnSave_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnSave.Click
- Dim FileWriter As StreamWriter
- Dim results As DialogResult
- results = SaveFileDialog1.ShowDialog
- If results = DialogResult.OK Then
- FileWriter = New StreamWriter(SaveFileDialog1.FileName, False)
- FileWriter.Write(TextBox1.Text)
- End If
- End Sub
- End Class
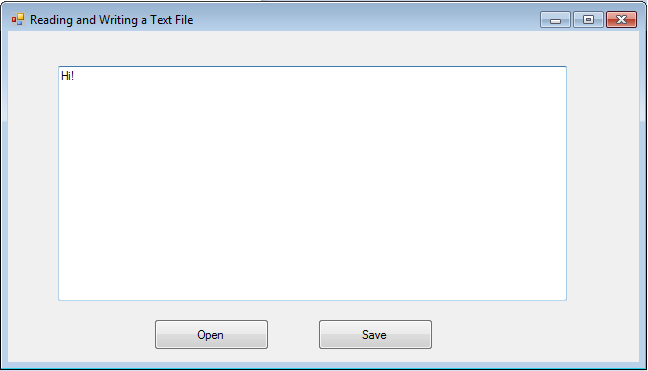
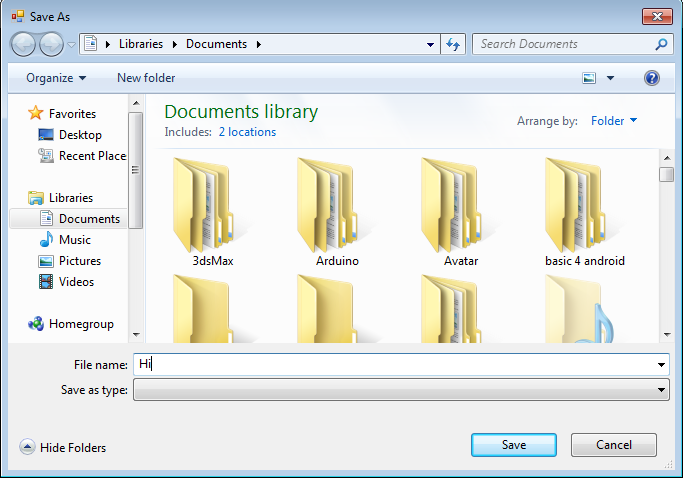
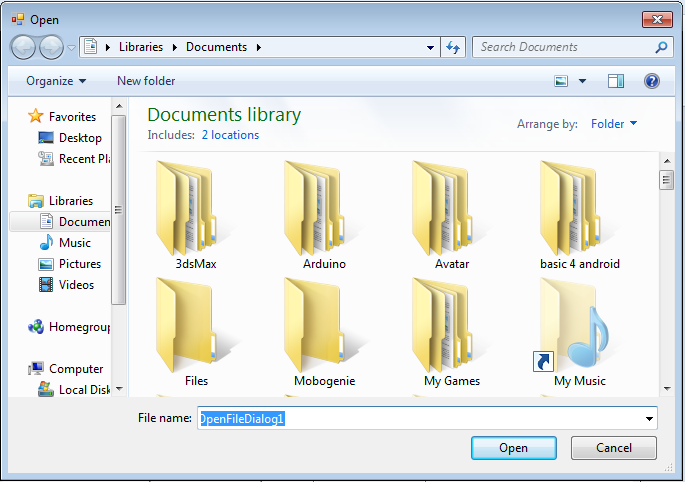
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz