Advance Computer Shutdown using Visual Basic.Net
Submitted by joken on Thursday, September 19, 2013 - 14:36.
In this tutorial we’re going to create a simple application that enable a user to shutdown, restart and log off the computer using visual basic. To do this open visual basic and create a new project and name it as “Shutdown”.
Designing the user interface:
The user interface in this project looks like as shown below.
To do this, we need to add three buttons and change their Text property into “Shutdown”,”Restart” and “Log Off”. Add a Label, and change the name into “lblmsg” and the Text property into “Computer will Shutdown in:”. Then add a textbox, and change the font size into “36”,Style into “Bold” and Text into “10” and the backcolor into "Black", then the forecolor to “lime”. And finally add three Timer.
This time, we need to add functionality to our application. To do this, double click the timer1 and add the following code:
It checks if the value of textbox1 is equal to zero then it will stop the timer and perform the shutting down of computer else, if the value of textbox1 is not equal to zero then it will decrement the value.
Next for timer2, add the following code:
Here in timer2 it simply does the restarting of a computer.
Next for timer3, add the following code:
Then for “Shutdown” button, add the following code:
It sets the text “lblmsg” to “Computer will Shutdown in:”, then it start the timer1.
Then for “Restart” button, add the following code:
It sets the text “lblmsg” to “Computer will Restart in:"then it start the timer2.
Then for “Log off” button, add the following code:
It sets the text “lblmsg” to “Computer will Log off in:"then it start the timer3.
After adding a code, you can start testing the program by pressing “F5”.
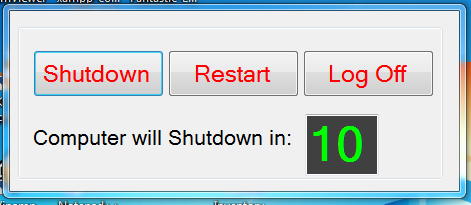
- Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick
- If TextBox1.Text = 0 Then
- Timer1.Stop()
- Shell("Shutdown -s")
- Else
- TextBox1.Text -= 1
- End If
- End Sub
- Next for timer2, add the following code:
- Here in timer2 it simply does the restarting of a computer.
- Private Sub Timer3_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer3.Tick
- If TextBox1.Text = 0 Then
- Timer3.Stop()
- Shell("Shutdown -l")
- Else
- TextBox1.Text -= 1
- End If
- End Sub
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- lblmsg.Text = "Computer will Shutdown in:"
- Timer1.Start()
- End Sub
- Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
- lblmsg.Text = "Computer will Restart in:"
- Timer2.Start()
- End Sub
- Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
- lblmsg.Text = "Computer will Log off in:"
- Timer3.Start()
- End Sub