How to Get Armstrong Numbers Between 1 to 1000 in VB.Net
Submitted by janobe on Monday, October 22, 2018 - 19:05.
If you wonder how to get Armstrong numbers in VB.Net, simply follow the procedure below to see if a number is an Armstrong number. But, before that let’s learn first what Armstrong number is? Is a number that when you raise each digit of the number to the power of the number of digits and add them up you still get the original number. It will be easier with an example, take a look at this. For example, 153 is an Armstrong number since (1^3) + (5^3) + (3^3) =153.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application.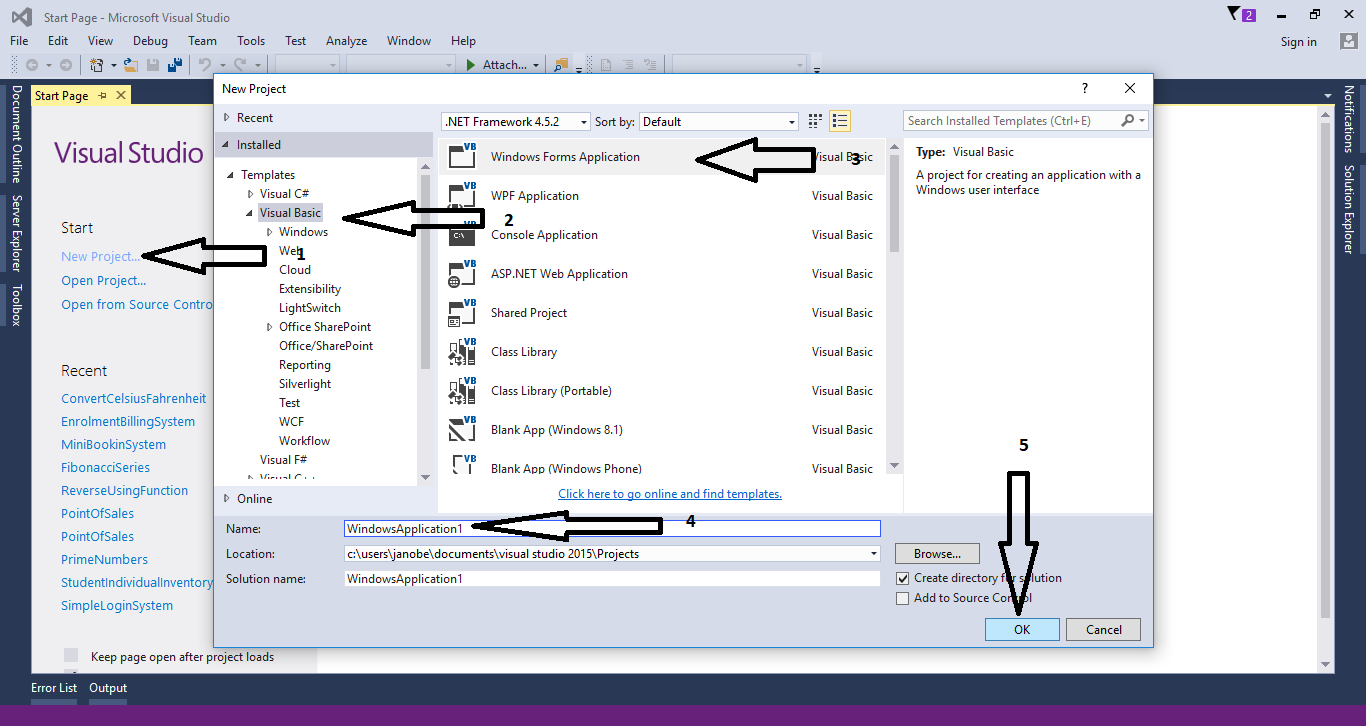
Step 2
Do the Form just like this.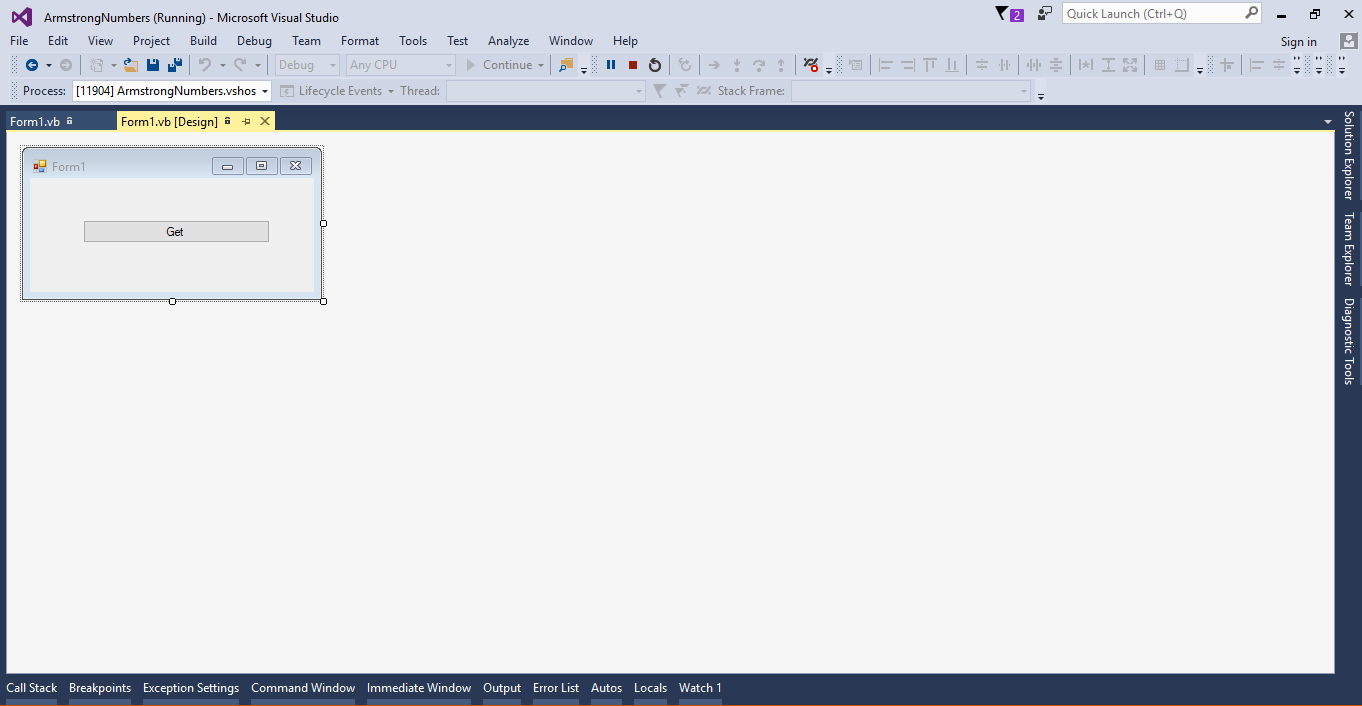
Step 3
Go to the code view and make a function for getting the Armstrong numbers.- Public Function displayArmstrongNumbers()
- Dim i, num As Integer
- Dim res As Integer
- Dim s As Integer
- For i = 2 To 1000
- num = i
- s = 0
- While num > 0
- res = num Mod 10
- s = s + (res * res * res)
- num = num \ 10
- End While
- If i = s Then
- Return i
- End If
- Next
- Return displayArmstrongNumbers()
- End Function
Step 4
Do the following code to display the Armstrong number in the message box when the button is clicked.- Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
- MsgBox(“Armsrtong Numbers betwwen 1 To 1000 are : ” & displayArmstrongNumbers())
- End Sub