Graphics Image Editor
Submitted by rinvizle on Friday, July 8, 2016 - 10:02.
Hello guys I will show you how to create a graphics image editor in Visual Basic.Net. This code is a image editor that can apply some effects like, zoom, sharpen, smooth, rotate, etc. All this using the .NET classes can operate pixel by pixel. The code shows also how to use clipboard class and open/save dialog and environments.
And for the Resize Script for image
This code is just an example, and you can use it freely as a base to build more applications. Enjoy Coding.
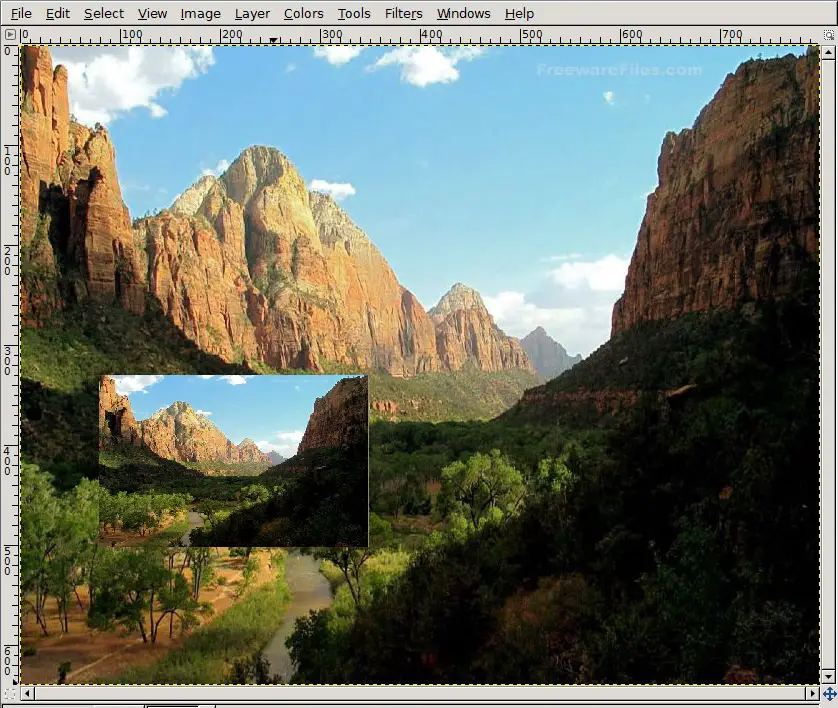
Sample Code
For the MDI Form Script- Begin
- VB.MDIForm MDIForm1
- BackColor = &H8000000C&
- Caption = "MDIForm1"
- ClientHeight = 3120
- ClientLeft = 75
- ClientTop = 405
- ClientWidth = 4650
- LinkTopic = "MDIForm1"
- End
- Attribute VB_Name = "MDIForm1"
- Attribute VB_GlobalNameSpace = False
- Attribute VB_Creatable = False
- Attribute VB_PredeclaredId = True
- Attribute VB_Exposed = False
- Private Sub MDIForm_Initialize()
- Me.Icon = LoadPicture(App.Path & "\p.ico")
- cx = GetSystemMetrics(SM_CXSCREEN)
- cy = GetSystemMetrics(SM_CYSCREEN)
- width = Form1.ScaleX(cx - 8, vbPixels, vbTwips)
- height = Form1.ScaleY(cy - 85, vbPixels, vbTwips)
- Form1.Move 0, 0, width, height
- MDIForm1.WindowState = 2
- End Sub
- Begin
- VB.Form resize
- BorderStyle = 1 'Fixed Single
- Caption = "Resize"
- ClientHeight = 2745
- ClientLeft = 45
- ClientTop = 375
- ClientWidth = 4155
- LinkTopic = "Form2"
- MaxButton = 0 'False
- MinButton = 0 'False
- ScaleHeight = 183
- ScaleMode = 3 'Pixel
- ScaleWidth = 277
- StartUpPosition = 1 'CenterOwner
- Begin VB.CommandButton Command2
- Caption = "Cancel"
- Height = 375
- Left = 1800
- TabIndex = 4
- Top = 2160
- Width = 975
- End
- Begin VB.CommandButton Command1
- Caption = "Ok"
- Height = 375
- Left = 360
- TabIndex = 3
- Top = 2160
- Width = 975
- End
- Begin VB.Frame Frame1
- Caption = "New Size"
- Height = 1815
- Left = 120
- TabIndex = 0
- Top = 240
- Width = 3855
- Begin VB.CheckBox Check1
- Caption = "Maintain Ascept Ratio"
- Height = 255
- Left = 240
- TabIndex = 10
- Top = 1320
- Value = 1 'Checked
- Width = 1935
- End
- Begin VB.TextBox Text3
- Height = 285
- Left = 2280
- Locked = -1 'True
- TabIndex = 9
- Top = 1320
- Width = 615
- End
- Begin VB.TextBox Text2
- Height = 375
- Left = 960
- MaxLength = 4
- TabIndex = 2
- Top = 720
- Width = 1335
- End
- Begin VB.TextBox Text1
- CausesValidation= 0 'False
- Height = 375
- Left = 960
- MaxLength = 4
- TabIndex = 1
- Top = 240
- Width = 1335
- End
- Begin VB.Label Label5
- Caption = "to 1"
- Height = 255
- Left = 3120
- TabIndex = 11
- Top = 1320
- Width = 375
- End
- Begin VB.Label Label4
- Caption = "Pixels"
- Height = 255
- Left = 2400
- TabIndex = 8
- Top = 840
- Width = 495
- End
- Begin VB.Label Label3
- Caption = "Pixels"
- Height = 255
- Left = 2400
- TabIndex = 7
- Top = 360
- Width = 615
- End
- Begin VB.Label Label2
- Caption = "Height"
- Height = 255
- Left = 240
- TabIndex = 6
- Top = 720
- Width = 495
- End
- Begin VB.Label Label1
- Caption = "Width"
- Height = 255
- Left = 240
- TabIndex = 5
- Top = 240
- Width = 495
- End
- End
- End
- Attribute VB_Name = "resize"
- Attribute VB_GlobalNameSpace = False
- Attribute VB_Creatable = False
- Attribute VB_PredeclaredId = True
- Attribute VB_Exposed = False
- Option Explicit
- Dim aratio As Single
- Private Sub Command1_Click()
- Form1.Picture1.Picture = LoadPicture()
- Form1.Picture1.width = Val(Text1.Text)
- Form1.Picture1.height = Val(Text2.Text)
- StretchBlt Form1.Picture1.hdc, 0, 0, Val(Text1.Text), Val(Text2.Text), Form1.Picture2.hdc, 0, 0, picwidth, picheight, vbSrcCopy
- picwidth = Val(Text1.Text)
- picheight = Val(Text2.Text)
- loading
- Unload Me
- End Sub
- Private Sub Command2_Click()
- Unload Me
- End Sub
- Private Sub Form_Load()
- Text1.Text = picwidth
- Text2.Text = picheight
- If picheight <> 0 Then aratio = picwidth / picheight: Text3.Text = aratio
- End Sub
- Private Sub Text1_KeyPress(KeyAscii As Integer)
- If KeyAscii = vbKeyBack Then
- ElseIf KeyAscii > Asc("9") Or KeyAscii < Asc("0") Then KeyAscii = 0
- End If
- End Sub
- Private Sub Text1_KeyUp(KeyCode As Integer, Shift As Integer)
- If Check1.Value = 1 Then Text2.Text = CInt(Val(Text1) / aratio)
- End Sub
- Private Sub Text1_MouseDown(Button As Integer, Shift As Integer, X As Single, Y As Single)
- If Button = vbRightButton Then Button = vbLeftButton
- End Sub
- Private Sub Text2_KeyPress(KeyAscii As Integer)
- If KeyAscii = vbKeyBack Then
- ElseIf KeyAscii > Asc("9") Or KeyAscii < Asc("0") Then KeyAscii = 0
- End If
- End Sub
- Private Sub Text2_KeyUp(KeyCode As Integer, Shift As Integer)
- If Check1.Value = 1 Then Text1.Text = CInt(Val(Text2) * aratio)
- End Sub
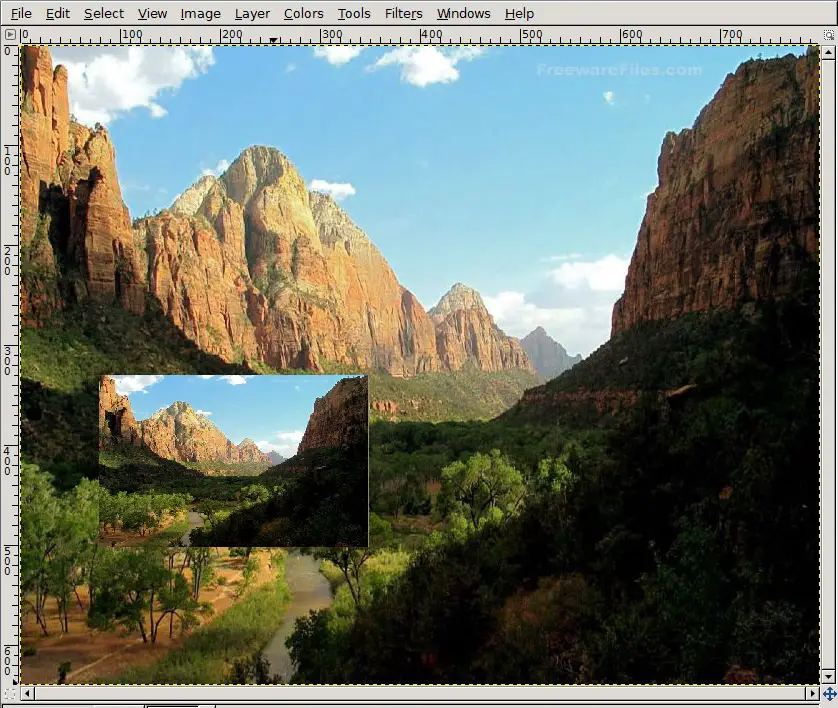
Add new comment
- 1489 views