PHP - Disable Login In 3 Attempts
Submitted by razormist on Sunday, April 28, 2019 - 09:03.
In this tutorial we will create a Disable Login In 3 Attempts using PHP. This code will automatically disable a login button in a 3 consecutive invalid login attempts. The code use SESSION to store a number of attempts everytime the user login an invalid account. This is a user-friendly kind of program feel free to modify it.
We will be using PHP as a scripting language that manage a database server to handle a bulk of data per transaction. It describe as an advance technology that manage both server and control-block of your machine.
There you have it we successfully created Disable Login In 3 Attempts using PHP. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating Database
Open your database web server then create a database name in it db_attempt, after that click Import then locate the database file inside the folder of the application then click ok.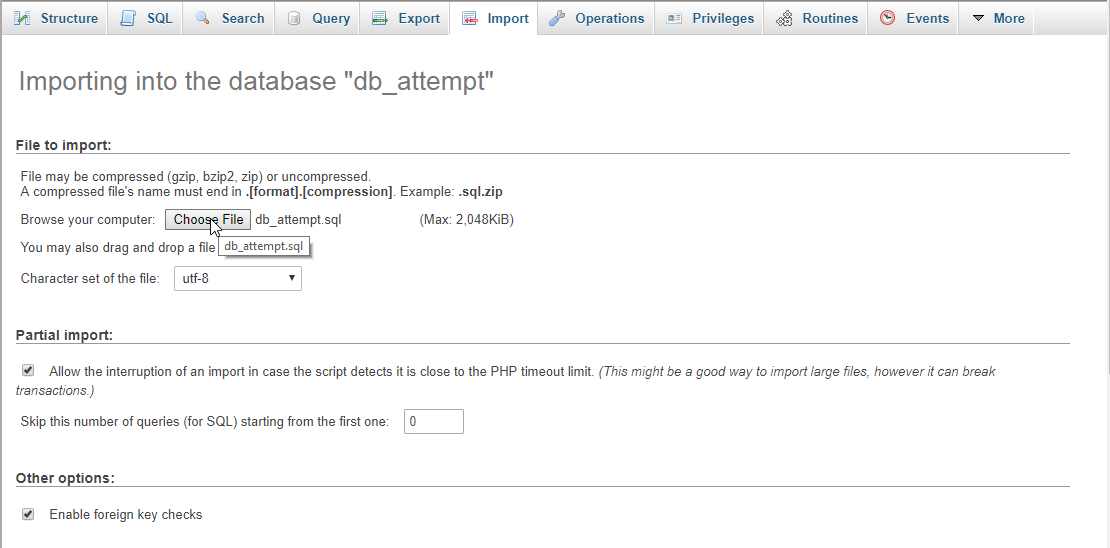
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Disable Login In 3 Attempts</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-3">
- <h5>Username: admin</h5>
- <h5>Password: admin</h5>
- </div>
- <div class="col-md-6">
- <form method="POST" action="">
- <div class="form-group">
- <label>Username</label>
- <input type="text" name="username" class="form-control" required="required"/>
- </div>
- <div class="form-group">
- <label>Password</label>
- <input type="password" name="password" class="form-control" required="required" />
- </div>
- <?php include 'login.php'?>
- <center><button class="btn btn-primary" name="login" <?php if(ISSET($_SESSION['msg'])){ echo $_SESSION['msg'];}?>><span class="glyphicon glyphicon-log-in"></span> Login</button> <a class="btn btn-success" href="reset.php"><span class="glyphicon glyphicon-refresh"></span> Reset</a></center>
- </form>
- </div>
- </div>
- </body>
- </html>
Creating Reset Function
This code contains the reset function of the application. This code will reset the entire sessions when the button is clicked. To do that just copy and write this block of codes inside the text editor, then save it as reset.php.- <?php
- ?>
Creating the Main Function
This code contains the main function of the application. This code will disable the login button in a 3 invalid logins. To make this just copy and write these block of codes below inside the text editor, then save it as login.php- <?php
- require 'conn.php';
- $username=$_POST['username'];
- $password=$_POST['password'];
- $query=mysqli_query($conn, "SELECT * FROM `user` WHERE `username`='$username' AND `password`='$password'") or die(mysqli_error());
- if($row > 0){
- echo "<center><label class='text-success'>Login success!</label></center>";
- }else{
- $_SESSION['attempt'] = 0;
- }
- $_SESSION['attempt'] += 1;
- if($_SESSION['attempt'] === 3){
- $_SESSION['msg'] = "disabled";
- }
- echo "<center><label class='text-danger'>Invalid username or password</label></center>";
- }
- }
- ?>
Add new comment
- 2974 views