Creating a Login and Registration Form using PHP PDO Tutorial
In this tutorial we will create a PDO Login and Registration using PHP. PHP is a server-side scripting language designed primarily for web development. PDO stands for PHP Data Objects. It is a lean and consistent way to access databases. This means developers can write portable code much easier. It is mostly used by a newly coders for its user-friendly environment.
So Let's do the coding...
Before we started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. Then, Open the XAMPP's Control Panel and start Apache
and MySQL
. Also I used Bootstrap for this tutorial, go to https://getbootstrap.com/ to download.
Creating Database
Open your database web server [i.e. http://localhost/phpmyadmin
] then create a database name in it db_login
.
To create the members table in the database. Click the SQL tab and copy/paste
the code below. Click the Go
button to submit the SQL Code.
Import the provided SQL
along with the working source code. To do this, click Import
tab in then locate the SQL file inside the database folder then click ok.
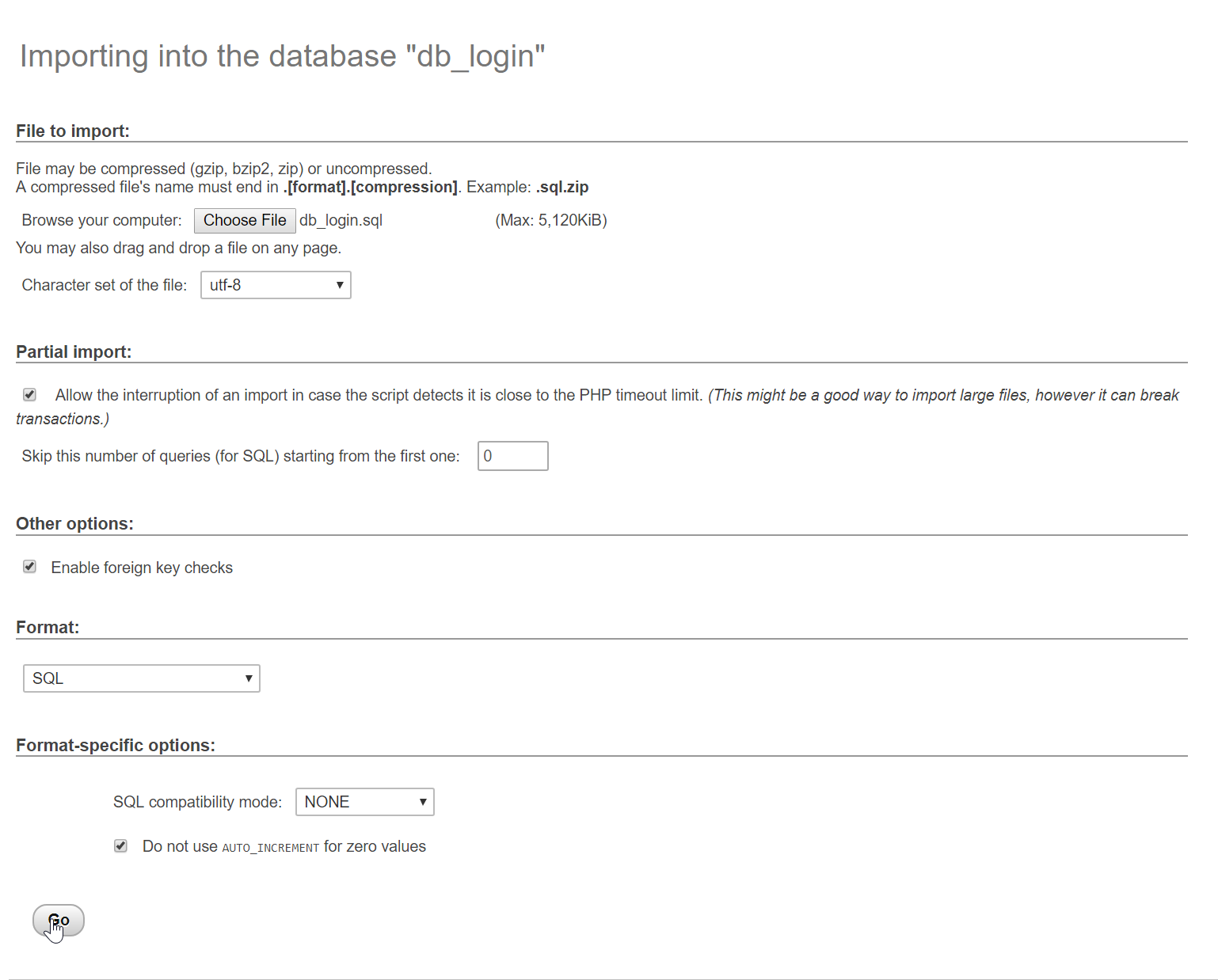
Creating the database connection
Open your any kind of text editor(notepadd++, etc..). Then just copy/paste
the code below then save it as conn.php
.
- <?php
- $db_username = 'root';
- $db_password = '';
- $conn = new PDO( 'mysql:host=localhost;dbname=db_login', $db_username, $db_password );
- if(!$conn){
- }
- ?>
Creating The Mark Up/Interface
This is where we will create a simple form for our application. To create the forms simply copy and paste it into you text editor, then save them as shown below.
index.php
This page is the default page when browsing the application. This shows the login form.
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - PDO Login and Registration</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-2"></div>
- <div class="col-md-8">
- <div class="alert alert-<?php echo $_SESSION['message']['alert'] ?> msg"><?php echo $_SESSION['message']['text'] ?></div>
- <script>
- (function() {
- // removing the message 3 seconds after the page load
- setTimeout(function(){
- document.querySelector('.msg').remove();
- },3000)
- })();
- </script>
- <?php
- endif;
- // clearing the message
- ?>
- <form action="login_query.php" method="POST">
- <h4 class="text-success">Login here...</h4>
- <hr style="border-top:1px groovy #000;">
- <div class="form-group">
- <label>Username</label>
- <input type="text" class="form-control" name="username" />
- </div>
- <div class="form-group">
- <label>Password</label>
- <input type="password" class="form-control" name="password" />
- </div>
- <br />
- <div class="form-group">
- <button class="btn btn-primary form-control" name="login">Login</button>
- </div>
- <a href="registration.php">Registration</a>
- </form>
- </div>
- </div>
- </body>
- </html>
registration.php
This page shows the registration form.
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - PDO Login and Registration</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-2"></div>
- <div class="col-md-8">
- <form action="register_query.php" method="POST">
- <h4 class="text-success">Register here...</h4>
- <hr style="border-top:1px groovy #000;">
- <div class="form-group">
- <label>Firstname</label>
- <input type="text" class="form-control" name="firstname" />
- </div>
- <div class="form-group">
- <label>Lastname</label>
- <input type="text" class="form-control" name="lastname" />
- </div>
- <div class="form-group">
- <label>Username</label>
- <input type="text" class="form-control" name="username" />
- </div>
- <div class="form-group">
- <label>Password</label>
- <input type="password" class="form-control" name="password" />
- </div>
- <br />
- <div class="form-group">
- <button class="btn btn-primary form-control" name="register">Register</button>
- </div>
- <a href="index.php">Login</a>
- </form>
- </div>
- </div>
- </body>
- </html>
home.php
This will be the page where user will be redirected after logging in.
- <!DOCTYPE html>
- <?php
- require 'conn.php';
- }
- ?>
- <html lang="en">
- <head>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - PDO Login and Registration</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-2"></div>
- <div class="col-md-8">
- <h3>Welcome!</h3>
- <br />
- <?php
- $id = $_SESSION['user'];
- $sql = $conn->prepare("SELECT * FROM `member` WHERE `mem_id`='$id'");
- $sql->execute();
- $fetch = $sql->fetch();
- ?>
- <center><h4><?php echo $fetch['firstname']." ". $fetch['lastname']?></h4></center>
- <a href = "logout.php">Logout</a>
- </div>
- </div>
- </body>
- </html>
Creating the Main Function
This code contains the specific script for the process of queries in the database. This code is consists of different functionalities, such as logout, login, and registration. To do that write these blocks of codes inside the Text editor and save them accordingly as shown below.
register_query.php
This is where the code register all the data in the input to the database server. PDO create a request then it will gather all the data that been collected ,and will store afterwards.
- <?php
- require_once 'conn.php';
- if($_POST['firstname'] != "" || $_POST['username'] != "" || $_POST['password'] != ""){
- try{
- $firstname = $_POST['firstname'];
- $lastname = $_POST['lastname'];
- $username = $_POST['username'];
- // md5 encrypted
- // $password = md5($_POST['password']);
- $password = $_POST['password'];
- $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- $sql = "INSERT INTO `member` VALUES ('', '$firstname', '$lastname', '$username', '$password')";
- }catch(PDOException $e){
- echo $e->getMessage();
- }
- $conn = null;
- }else{
- echo "
- <script>alert('Please fill up the required field!')</script>
- <script>window.location = 'registration.php'</script>
- ";
- }
- }
- ?>
login_query.php
This where the code can login the user. PDO send a request to the database server then receive some confirmation if the user account is a valid to access the data.
- <?php
- require_once 'conn.php';
- if($_POST['username'] != "" || $_POST['password'] != ""){
- $username = $_POST['username'];
- $password = $_POST['password'];
- $sql = "SELECT * FROM `member` WHERE `username`=? AND `password`=? ";
- $query = $conn->prepare($sql);
- $row = $query->rowCount();
- $fetch = $query->fetch();
- if($row > 0) {
- $_SESSION['user'] = $fetch['mem_id'];
- } else{
- echo "
- <script>alert('Invalid username or password')</script>
- <script>window.location = 'index.php'</script>
- ";
- }
- }else{
- echo "
- <script>alert('Please complete the required field!')</script>
- <script>window.location = 'index.php'</script>
- ";
- }
- }
- ?>
logout.php
This where the code can logout the user account. The user will be will force to logout when the logout button is click.
- <?php
- ?>
DEMO
There you have it we successfully created a PDO Login and Registration using PHP. You can now test your work if it runs properly or the way we intend to create it in this tutorial. If there's an error occurred on your end, kindly recheck the code or download the working source code to differentiate it from your work.
I hope that this simple tutorial helps you to what you are looking for. For more updates and tutorials just kindly visit this site.
Enjoy Coding!!!