Simple Facebook Wall
Submitted by rinvizle on Tuesday, August 2, 2016 - 17:14.
In this tutorial, we are going to create our own version of Simple Facebook Wall. Using jQuery and PHP. I will explain how I added code behind the “Share button” so that when clicked, it will post the content of the text area as wall post.
Sample Code
PHP and Html Form
For the Function of the message in the content.
For the Image and Title to display in the Content.
Hope that you learn in this project and enjoy coding. Don't forget to LIKE & SHARE this website.
- <!DOCTYPE html>
- <html>
- <head>
- <title>Simple Facebook Wall</title>
- <script src="js/jquery.min.js"></script>
- <script type="text/javascript" src="js/jquery.livequery.js"></script>
- <body>
- <nav class="navbar navbar-default">
- <d iv class="container-fluid">
- <div class="navbar-header">
- <a class="navbar-brand" href="index.php"><a><img src="image/icontexto-inside-facebook.png" width="50px" height="50px"></a> Facebook</a>
- </div>
- </div>
- </nav>
- <div id="wrapper">
- <form>
- <input name="current_img" id="current_img" type="hidden"/>
- <input name="ajax_flag" id="ajax_flag" type="hidden"/>
- </form>
- <div>
- <div id="fetched_data">
- <div id="loader"> </div>
- <div id="ajax_content"></div>
- </div>
- </div>
- <div id="textareaWrap">
- <textarea id="wall"></textarea>
- <div id="sharebtn"> <a class="button Share" style="" id="shareButton"> Share</a> </div>
- </div>
- <div id="wallz" class="fb_wall">
- <ul id="posts">
- <li> <img src="image/avatar.png" class="avatar">
- <div class="status">
- <h2><a href="#" target="_blank">Sourcecodester</a></h2>
- <p class="message">The Use of a New Bootstrap Modal</p>
- <div class="img_attachment"> <img class="external_pic" src="image/cover.jpg">
- <div class="data">
- <p class="name"><a href="http://www.sourcecodester.com/tutorials/other/10617/how-use-modal-bootstrap.html"_blank">How to use Modal Bootstrap</a></p>
- <p class="caption">www.sourcecodester.com</p>
- <p class="description">Good day everyone, today, we are going to tackle about Bootstrap Modals. It is the front-end designing purpose whatever you want to use it. You can use it to any confirmation, to add, edit, delete data, to view data in the modal form, and to maximize the space of your page when you using it...<a href="http://www.sourcecodester.com/tutorials/other/10617/how-use-modal-bootstrap.html">See More!</a></p>
- </div>
- </div>
- </div>
- <p class="likes"><a>25k Likes</a> - 1 hour ago</p>
- </li>
- <li> <img src="image/avatar.png" class="avatar">
- <div class="status">
- <h2><a href="#" target="_blank">Sourcecodester</a></h2>
- <p class="message">Linux Making The Computer Use Easier</p>
- <div class="img_attachment"> <img class="external_pic" src="image/linux.gif">
- <div class="data">
- <p class="name"><a href="http://www.sourcecodester.com/blog/10619/linux-making-computer-use-lot-easier.html" target="_blank">Linux On Making Computer Use A Lot Easier</a></p>
- <p class="caption">www.sourcecodester.com</p>
- <p class="description">Computer usage is like counting 1,2,3 for some while for others it's a whole new thing to be learned. Most especially to those who were not used to it, it's going to be a crack on the nutshell that a friendly operating system would really be a big help.
- Linux has recently been reported as a system that provides freedom, security, and easy access. Way back in 1991, mixed-up with the GNU software of Richard Stallman, a man named Linus Torvalds made this free operating system come to possibility...<a href="http://www.sourcecodester.com/blog/10619/linux-making-computer-use-lot-easier.html">See More!</a></p>
- </div>
- </div>
- </div>
- <p class="likes"><a>11k Likes</a> - 5 hour ago</p>
- </li>
- </ul>
- </div>
- </div>
- </body>
- </html>
- $(document).ready(function () {
- $('#shareButton').livequery("click", function () {
- var textarea_content = $('textarea#wall').val();
- if (textarea_content != '') {
- var sitetitle = $('label.title').html();
- if (sitetitle == null) {
- sitetitle = ' ';
- }
- var siteurl = $('label.url').html();
- if (siteurl == null) {
- siteurl = ' ';
- }
- var sitedesc = $('label.desc').html();
- if (sitedesc == null) {
- sitedesc = ' ';
- }
- var current_image_id = $('input#current_img').val();
- if (current_image_id != '') {
- var current_image_url = $("img#" + current_image_id).attr("src");
- if (current_image_url != '') {
- var image_html = '<div class="img_attachment"> <img class="external_pic" width="90" height="67" src="' + current_image_url + '">';
- } else {
- var image_html = '';
- }
- } else {
- var image_html = '';
- }
- var wall_post = '<li> <img src="image/avatar.png" class="avatar"><div class="status"><h2><a href="#" target="_blank">Sourcecodester</a></h2><p class="message">' + textarea_content + '</p> ' + image_html + '<div class="data"><p class="name"><a href="' + siteurl + '" target="_blank">' + sitetitle + '</a></p><p class="caption">' + siteurl + '</p><p class="description">' + sitedesc + '</p></div></div> </div><p class="likes"><a>Like</a> - 1 second ago</p></li>';
- var message_wall = $('#message_wall').attr('value');
- $.ajax({
- type: "GET",
- data: "message_wall=" + wall_post,
- success: function () {
- $('ul#posts').prepend(wall_post);
- }
- });
- $('textarea#wall').val('');
- $('#ajax_content').empty();
- $('#fetched_data').hide();
- } else {
- alert('Enter some text ! ');
- }
- });
- });
- <?php
- echo '<div class="images">';
- $y = $i + 1;
- echo '<img style="display: none;" src="' . $images_url[$i] . '" id="' . $y .
- '" width="100"/>';
- }
- echo '<input name="total_images" id="total_images" value="' . count($images_url) .'" type="hidden"/>';
- echo '</div>';
- }
- echo '<div class="info">';
- echo ' <label class="title"> ' . $title . ' </label>';
- }
- echo ' <br clear="all"/>';
- echo '<label class="url"> ' . $url . ' </label>';
- echo '<br clear="all"/>';
- }
- echo ' <br clear="all"/>';
- echo '</div>';
- ?>
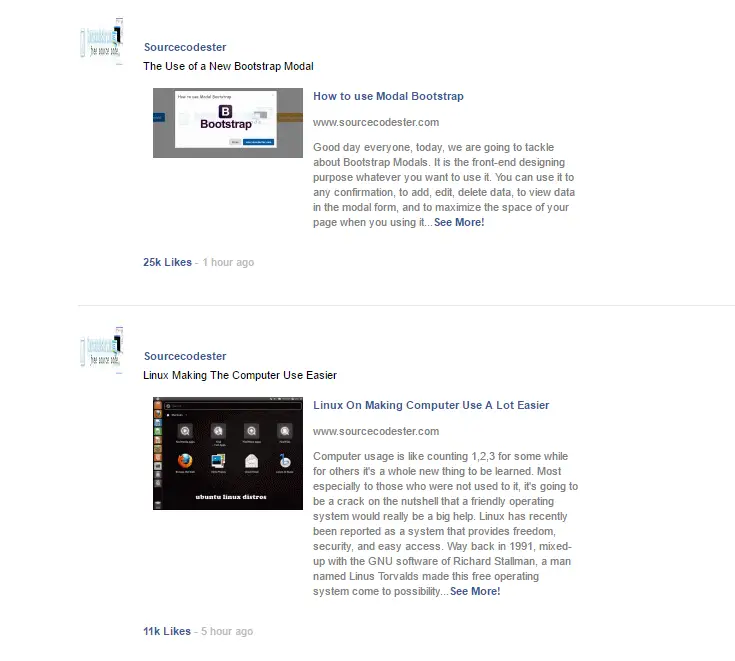