Submit PHP Form without Page Refresh
In this article, we are going to create on how to submit
Submit PHP Form without Page Refresh using jQuery and Ajax. We are going to use these two functions so we can submit PHP Forms without any refreshing in our page and we are going to use the $_POST request in PHP. Let's do this:
Creating Simple HTML Form
Kindly copy and paste to your BODY tag of your page. Save it as "index.php".
<div id="form" class="result_1">
<form method="post" id="registration_Form">
<td><input type="text" name="first_name" id="first_name" placeholder="Enter your First Name" autofocus="autofocus"/></td>
<td><input type="text" name="last_name" id="last_name" placeholder="Enter your Last Name" /></td>
<td><input type="text" name="user_name" id="user_name" placeholder="Enter your User Name" /></td>
<td><input type="text" name="password" id="password" placeholder="Enter your Password" /></td>
<td><input type="text" name="email" id="email" placeholder="Enter your Email" /></td>
Script
Copy and paste to your HEAD tag of your page in index.php.
<script type="text/javascript" src="js_code.js"></script>
<script type="text/javascript">
$(document).ready(function()
{
$(document).on('submit', '#registration_Form', function()
{
var data = $(this).serialize();
$.ajax({
type : 'POST',
url : 'submit.php',
data : data,
success : function(data)
{
$("#registration_Form").fadeOut(500).hide(function()
{
$(".result_1").fadeIn(500).show(function()
{
$(".result_1").html(data);
});
});
}
});
return false;
});
});
</script>
Result Page
Kindly copy and paste this source code and save it as "submit.php".
<?php
if($_POST)
{
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
$user_name = $_POST['user_name'];
$password = $_POST['password'];
$email = $_POST['email'];
?>
<td colspan="2" style="color:blue; font-size:30px; font-family:cursive; font-weight:bold;">Successfully Registered !!!
</td>
<td style="color:blue; font-size:18px; font-family:cursive; font-weight:bold;">First Name
</td>
<td style="color:red; font-size:18px; font-family:cursive; font-weight:bold;"><?php echo $first_name; ?></td>
<td style="color:blue; font-size:18px; font-family:cursive; font-weight:bold;">Last Name
</td>
<td style="color:red; font-size:18px; font-family:cursive; font-weight:bold;"><?php echo $last_name; ?></td>
<td style="color:blue; font-size:18px; font-family:cursive; font-weight:bold;">User Name
</td>
<td style="color:red; font-size:18px; font-family:cursive; font-weight:bold;"><?php echo $user_name; ?></td>
<td style="color:blue; font-size:18px; font-family:cursive; font-weight:bold;">User Name
</td>
<td style="color:red; font-size:18px; font-family:cursive; font-weight:bold;"><?php echo $password; ?></td>
<td style="color:blue; font-size:18px; font-family:cursive; font-weight:bold;">Email
</td>
<td style="color:red; font-size:18px; font-family:cursive; font-weight:bold;"><?php echo $email; ?></td>
<?php
}
?>
Result:
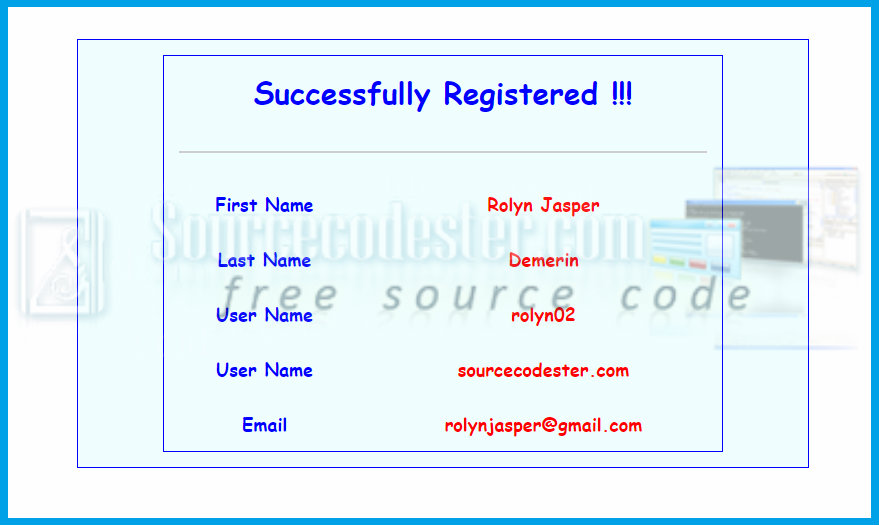
That's all, you can submit
PHP Forms without page refreshing.
Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at
[email protected]. Practice Coding. Thank you very much.