Sign Up Form Using Bootstrap with jQuery Validation
Submitted by alpha_luna on Thursday, May 26, 2016 - 15:05.
Sign Up Form Using Bootstrap with jQuery Validation
If you are looking for on how to create sign up form using bootstrap then you are at the right place. We all know using bootstrap is fabulous in term of designing and this is the front end framework of a website. Using bootstrap is for designing objective. In this tutorial, we are going to learn on how to create registration form using bootstrap with jQuery validation.Sign Up Form
This is the form field where the user enters their information to signup and the classes of bootstrap.- <div class="container">
- <div class="wrapper">
- <!-- form start -->
- <form method="post" role="form" id="register-form" autocomplete="off" action="submit.html">
- <div class="header_style">
- <div class="pull-right">
- </div>
- </div>
- <div class="body_style">
- <div class="alert alert-info" id="message" style="display:none;">
- Successfully Registered!!!
- </div>
- <div class="form-group">
- <div class="input-group">
- <input name="name" type="text" class="form-control" autofocus="autofocus" placeholder="User Name ....." style="border:1px solid blue;">
- </div>
- </div>
- <div class="form-group">
- <div class="input-group">
- <input name="email" type="text" class="form-control" placeholder="Email ....." style="border:1px solid blue;">
- </div>
- </div>
- <div class="row">
- <div class="form-group col-lg-6">
- <div class="input-group">
- <input name="password" id="password" type="password" class="form-control" placeholder="Password ....." style="border:1px solid blue;">
- </div>
- </div>
- <div class="form-group col-lg-6">
- <div class="input-group">
- <input name="cpassword" type="password" class="form-control" placeholder="Re-type Password ....." style="border:1px solid blue;">
- </div>
- </div>
- </div>
- </div>
- <div class="footer_style">
- <button type="submit" class="btn btn-success" style="border:1px solid blue;">
- </button>
- </div>
- </form>
- </div>
- </div>
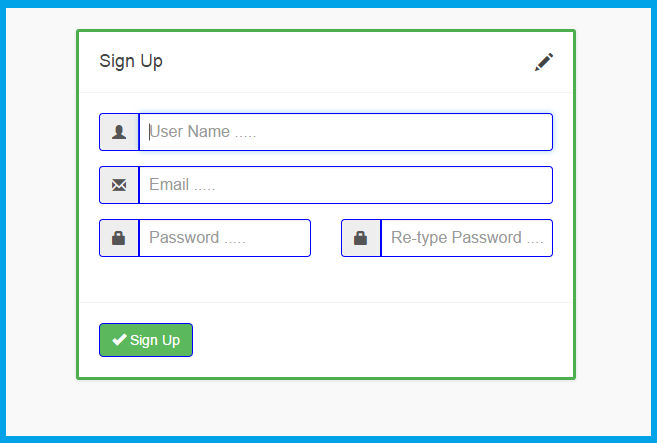
jQuery Validation
Here the links. Put this file before end of your BODY tag.- $('document').ready(function()
- {
- // name validation
- var nameregex = /^[a-zA-Z ]+$/;
- $.validator.addMethod("validname", function( value, element ) {
- return this.optional( element ) || nameregex.test( value );
- });
- // valid email pattern
- var eregex = /^([a-zA-Z0-9_\.\-\+])+\@(([a-zA-Z0-9\-])+\.)+([a-zA-Z0-9]{2,4})+$/;
- $.validator.addMethod("validemail", function( value, element ) {
- return this.optional( element ) || eregex.test( value );
- });
- $("#register-form").validate({
- rules:
- {
- name: {
- required: true,
- validname: true,
- minlength: 4
- },
- email: {
- required: true,
- validemail: true
- },
- password: {
- required: true,
- minlength: 8,
- maxlength: 15
- },
- cpassword: {
- required: true,
- equalTo: '#password'
- },
- },
- messages:
- {
- name: {
- required: "<b style='font-family:cursive; font-size:18px; color:red;'>Please Enter Correct User Name</b>",
- validname: "<b style='font-family:cursive; font-size:18px; color:red;'>Name must contain only alphabets and space</b>",
- minlength: "<b style='font-family:cursive; font-size:18px; color:red;'>Your Name is Too Short</b>"
- },
- email: {
- required: "<b style='font-family:cursive; font-size:18px; color:red;'>Please Enter Correct Email Address</b>",
- validemail: "<b style='font-family:cursive; font-size:18px; color:red;'>Enter Valid Email Address</b>"
- },
- password:{
- required: "<b style='font-family:cursive; font-size:18px; color:red;'>Please Enter Correct Password</b>",
- minlength: "<b style='font-family:cursive; font-size:18px; color:red;'>Password at least have 8 characters</b>"
- },
- cpassword:{
- required: "<b style='font-family:cursive; font-size:18px; color:red;'>Please Re-type Your Password</b>",
- equalTo: "<b style='font-family:cursive; font-size:18px; color:red;'>Password Did not Match !</b>"
- }
- },
- errorPlacement : function(error, element) {
- $(element).closest('.form-group').find('.help-block').html(error.html());
- },
- highlight : function(element) {
- $(element).closest('.form-group').removeClass('has-success').addClass('has-error');
- },
- unhighlight: function(element, errorClass, validClass) {
- $(element).closest('.form-group').removeClass('has-error').addClass('has-success');
- $(element).closest('.form-group').find('.help-block').html('');
- },
- }
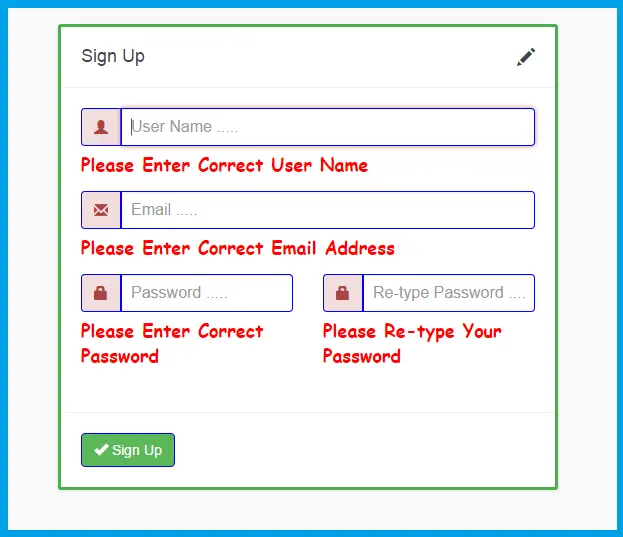
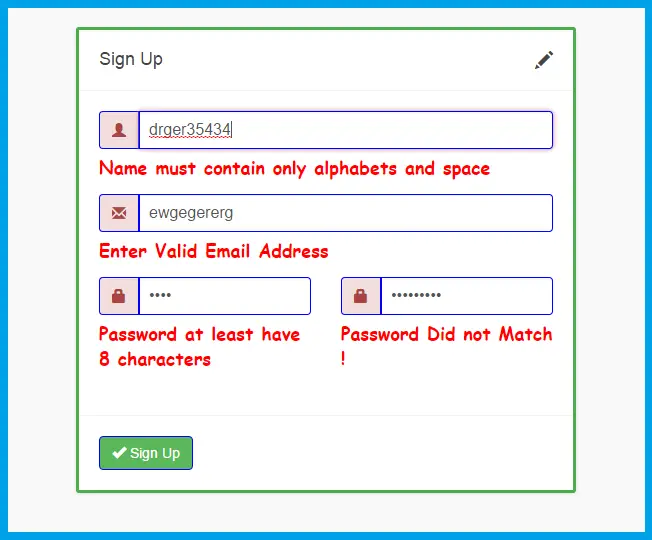
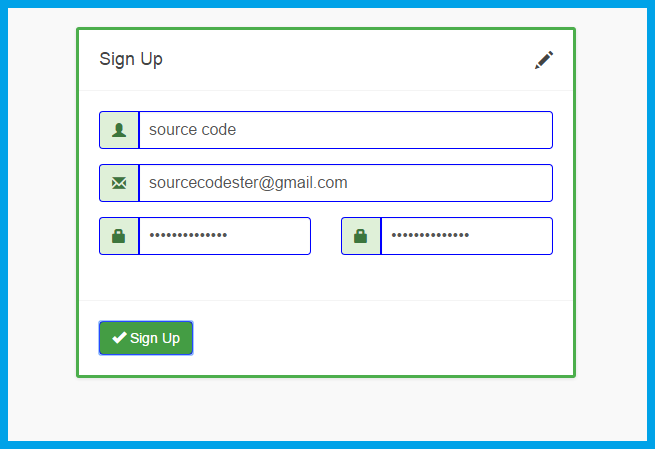