Fibonacci Number in Java
Submitted by GeePee on Saturday, May 23, 2015 - 22:30.
The following Java program application is a Fibonacci number. I will be using the JCreator IDE in developing the program.
To start in this tutorial, first open the JCreator IDE, click new and paste the following code
Sample Run:
The program works as follow:
The statement prompts the user for the first Fibonacci number that is, display the input dialog box to prompt the user for the second Fibonacci number.
The statement create output and append previous1 and previous2.
The statement displays the input dialog box to prompt the user for the desired Fibonacci number and store the nth Fibonacci into inputString.
The statement display the output dialog box showing the first two and nth Fibonacci number.
- import javax.swing.JOptionPane;
- public class FibonacciNumber
- {
- {
- //declaration of variables
- String inputString;
- String outputString;
- int previous1;
- int previous2;
- int current = 0;
- int counter;
- int nthFibonacci;
- inputString =
- inputString =
- outputString = "The first two numbers of the"
- + "Fibonacci sequence are: "
- + previous1 + "and" + previous2;
- inputString =
- + "number in the Fibonacci sequence: ");
- if (nthFibonacci == 1) //copies the value of previous1 into current
- current = previous1;
- else if (nthFibonacci == 2)//copies the value of previous2 to current
- current = previous2;
- else //calculate the desired Fibonacci number
- {
- counter = 3;
- while (counter <= nthFibonacci)
- {
- current = previous2 + previous1;
- previous1 = previous2;
- previous2 = current;
- counter++;
- }
- }
- outputString = outputString + "\nThe "
- + nthFibonacci
- + "th Fibonacci of "
- + "the sequence is: "
- + current;
- "Fibonacci Number",
- }
- }
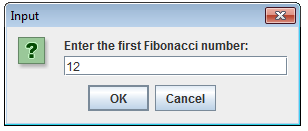
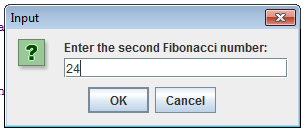
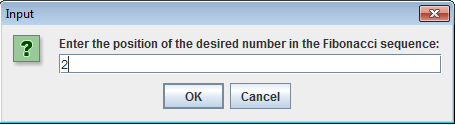
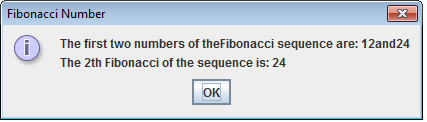
- inputString =
previous1
and it stores into inputString
.
The statement - inputString =
- outputString = "The first two numbers of the"
- + "Fibonacci sequence are: "
- + previous1 + "and" + previous2;
- inputString =
- + "number in the Fibonacci sequence: ");
- "Fibonacci Number",
Add new comment
- 445 views