OverlayLayout as Layout Manager in Java
Submitted by donbermoy on Thursday, December 4, 2014 - 13:09.
This tutorial is about the OverlayLayout as Layout Manager in Java. An OverlayLayout is a layout where it arranges components over the top of each other or behind each other.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of overlayLayout.java.
2. Import following package library:
3. Initialize your variables in your Main, variable frame for JFrame, variable panel as JPanel, variable overlay for OverlayLayout and put inside the JPanel on it, and variable button labeled Small as JButton as we will create buttons on the top of each other.
4. Now, we will create three buttons using a single variable for the JButton. That is to have these buttons to be in top of each other.
We will create the first button. To set its size we will use the setMaximumSize method with the dimension class that has the width and height of the button. Set also the background color of the button by using the setBackground method. Lastly add the button using the add method of the panel. Note that this button is for the button labeled as "Small".
To create another button from the variable of the JButton named button, we will instantiate again the button but has different label, namely "Medium". Have all its size, background color, and add the button to the panel.
5. Lastly, add the panel to the frame with a default BorderLayout of Center position, set its size and visibility, and its close operation.
Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*; // used to access Color and Dimension class
- import javax.swing.*; //used to access JButton,JFrame,JPanel, and OverlayLayout class
Repeat the instruction to create other button and name this new button as "Large".
Now, set the OverlayLayout as your Layout Manager in the panel not on the frame.
- panel.setLayout(overlay);
- frame.getContentPane().add(panel, "Center");
- frame.setSize(400, 300);
- frame.setVisible(true);
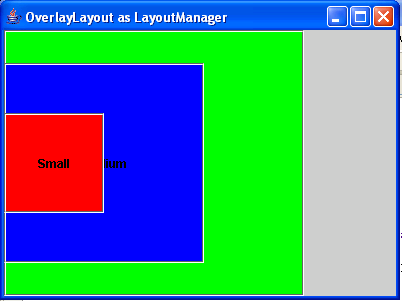
- import java.awt.*; // used to access Color and Dimension class
- import javax.swing.*; //used to access JButton,JFrame,JPanel, and OverlayLayout class
- public class overlayLayout {
- panel.add(button);
- panel.add(button);
- panel.add(button);
- panel.setLayout(overlay);
- frame.getContentPane().add(panel, "Center");
- frame.setSize(400, 300);
- frame.setVisible(true);
- }
- }