JWindow and JFrame Component in Java
Submitted by donbermoy on Wednesday, November 26, 2014 - 09:58.
This is a tutorial in which we will going to create a program that will have a JWindow and JFrame Component in Java. We will first differentiate the two components. The JFrame can be a container of other components, has title and border, and has buttons for minimize, maximize, and close. Unlike JFrame, a JWindow can also hold other components but it has no title and border, and no minimize, maximize, and close button.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jWindowComponent.java.
2. Import the following packages:
3. Initialize your variable in your Main, variable frame for JFrame, variable window for JWindow, and variable panel for JPanel.
Now, we will create a FlowLayout for the panel and a Label inside the panel.
5. Lastly, we will set the size and location of the Frame and the Window.
Then, we will set the visibility to True for the two components to be seen by the user.
Frame:
Window:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*; // used to access the JFrame, JWindow, and JPanel class
- import java.awt.*; // used to access the FlowLayout class
4. We will add the JPanel component into the window, and it will have a default BorderLayout of Center position.
- window.getContentPane().add(panel, "Center");
- frame.setSize(300, 300);
- frame.setLocation(100, 100);
- window.setSize(300, 300);
- window.setLocation(500, 100);
- frame.setVisible(true);
- window.setVisible(true);
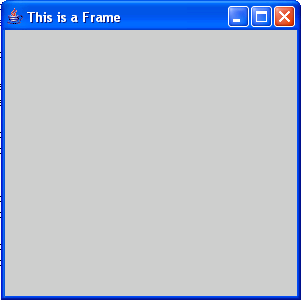
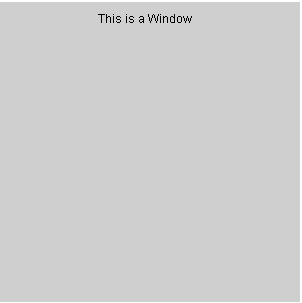
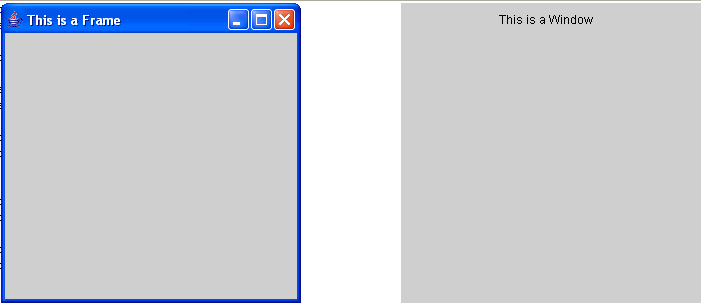
- import javax.swing.*; // used to access the JFrame, JWindow, and JPanel class
- import java.awt.*; // used to access the FlowLayout class
- public class jWindowComponent {
- window.getContentPane().add(panel, "Center");
- frame.setSize(300, 300);
- frame.setLocation(100, 100);
- window.setSize(300, 300);
- window.setLocation(500, 100);
- frame.setVisible(true);
- window.setVisible(true);
- }
- }