JCheckBox Component in Java
Submitted by donbermoy on Monday, November 17, 2014 - 17:47.
This is a tutorial in which we will going to create a program that has the JCheckBox Component using Java. The JCheckBox is used to let the user select one or more options. It is also a component that an item can be selected or deselected, and which displays its state to the user.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jCheckBoxComponent.java.
2. Import the following packages:
3. Initialize your variable in your Main, variable frame for creating JFrame, and variable letter a to f for the checkboxes.
5. Now, to select the item to checkbox after running it, use the setSelected method and return to true.
6. Add a JLabel and a JCheckBox component to the frame using the add method, then pack the frame so that the component/control will be seen directly to the frame. Also set its visibility to true and have it the close operation to exit.
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*; //used to access the FlowLayout class
- import java.awt.event.*; //used to access the ItemListener and ItemEvent class
- import javax.swing.*; //used to access the JFrame,JLabel, and JCheckBox class
As you can see the above code, I created 6 variables for the JCheckBox component. Variable a for Bird, variable b for Chicken, variable c for Pig, variable d for Cow, variable e for Dog, and variable f for Cat. I used the final keyword for variable c as checkbox because I want to create an inner class within it from the ItemEvent and ItemListener class.
4. Add ItemListener to JCheckBox variable c with the itemStateChanged method. This will trigger to check if the checkbox is checked or not using the isSelected method. Have this code below:
- }
- });
- a.setSelected(true);
- frame.getContentPane().add(a);
- frame.getContentPane().add(b);
- frame.getContentPane().add(c);
- frame.getContentPane().add(d);
- frame.getContentPane().add(e);
- frame.getContentPane().add(f);
- frame.pack();
- frame.setVisible(true);
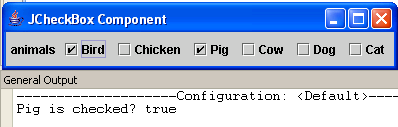
- import java.awt.*; //used to access the FlowLayout class
- import java.awt.event.*; //used to access the ItemListener and ItemEvent class
- import javax.swing.*; //used to access the JFrame,JLabel, and JCheckBox class
- public class jCheckBoxComponent {
- }
- });
- a.setSelected(true);
- frame.getContentPane().add(a);
- frame.getContentPane().add(b);
- frame.getContentPane().add(c);
- frame.getContentPane().add(d);
- frame.getContentPane().add(e);
- frame.getContentPane().add(f);
- frame.pack();
- frame.setVisible(true);
- }
- }