Java Animation: Color Fading Tutorial
Submitted by donbermoy on Sunday, November 9, 2014 - 08:51.
In this tutorial, we will create a java animation program that has the fading color effects.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of ColorFading.java.
2. Import the following packages:
3. Instantiate a variable for 2D Rectangle and the alpha/fade rectangle.
4. Create the paint method for the graphics to create the 2D Rectangle and fill it with color and the fading effects.
5. Create the run method for the fading effects with the use of the thread.
8. In your Main, create a JFrame component that will hold all other components. This will set the size, location, title, and visibility.
Here's the full code of this tutorial:
Hope this helps!
Best regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*; //this will be used to access graphics,color, and alpha composite
- import java.awt.geom.Rectangle2D;// used to create a 2-Dimensional Rectangle
- import javax.swing.*;//used to have JPanel and JFrame
- private float fade_rectangle = 1f;
- super.paint(g); //paint the frame
- g2d.setComposite(AlphaComposite.getInstance(AlphaComposite.SRC_OVER, fade_rectangle)); //fading effects of the rectangle
- g2d.fill(rect);//fill the rectangle
- }
6. Create another class named RectRunnable that implements Runnable to call the thread to run.
7. Create a constructor and have it an instantiation of the class RectRunnable to run the fading.
- public ColorFading() {
- new RectRunnable();
- }
Output:
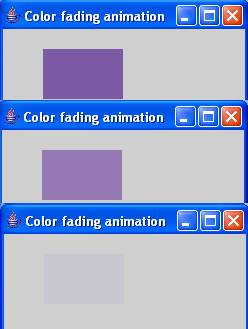
- import java.awt.*; //this will be used to access graphics,color, and alpha composite
- import java.awt.geom.Rectangle2D;// used to create a 2-Dimensional Rectangle
- import javax.swing.*;//used to have JPanel and JFrame
- private float fade_rectangle = 1f;
- public ColorFading() {
- new RectRunnable();
- }
- super.paint(g); //paint the frame
- g2d.setComposite(AlphaComposite.getInstance(AlphaComposite.SRC_OVER, fade_rectangle)); //fading effects of the rectangle
- g2d.fill(rect);//fill the rectangle
- }
- frame.getContentPane().add(new ColorFading());
- frame.setSize(250, 150);
- frame.setVisible(true);
- }
- public RectRunnable() {
- runner.start();
- }
- public void run() {
- while (fade_rectangle >= 0) {
- repaint();
- fade_rectangle += -0.01f;
- if (fade_rectangle < 0) {
- fade_rectangle = 0;
- }
- try {
- }
- }
- }
- }
- }