PACMAN Animation Programming part2
Submitted by moazkhan on Thursday, July 3, 2014 - 21:05.
TUTORIAL NO 12
PACMAN Animation Programming part2
In this tutorial you will learn: 1. Game Programming 2. Swing Animations 3. Event handling 4. JAVA awt 5. JAVA swing 6. Adapters Now we will continue making our PacMan animation. In this tutorial we will write the functions and conditions for up , down ,left and right. Which we were calling in our detect collision function. If you haven’t read my previous tutorial please read that tutorial first http://www.sourcecodester.com/tutorials/java/7525/pacman-animation-programming-part1.html Basic step: Download and install ECLIPSE and set up a JAVA PROJECT. Then first go through my pacman tutorial part1 then follow this tutorial. Follow these steps 1.DIRECTION CONTROL FUNCTIONS: We will write the conditions for setting the directions according to the function name.- void UP() {
- if (s_angle >= 45 & s_angle < 90 & e_angle <= -270 & flag == 1) { // when mouth is open
- s_angle++; //increasing starting angle
- e_angle -= 2; // increasing ending angle
- if (s_angle == 90) {
- s_angle = 90;
- e_angle = -360;
- flag = 2; // changing flag when mouth is closed making the other condition true
- }
- }
- if (s_angle > 45 & s_angle <= 90 & e_angle >= -360 & flag == 2) { // when mouth is closed
- s_angle--; //decreasing starting angle
- e_angle += 2; // increasing ending angle
- if (s_angle <= 45) {
- flag = 1;
- e_angle = -270;
- }
- }
- } // UP
- void down() {
- if (s_angle >= 225 & s_angle < 270 & e_angle <= -270 & flag == 1) { // when mouth is open
- s_angle++; //increasing starting angle
- e_angle -= 2; //decreasing ending angle
- if (s_angle == 270) {
- s_angle = 270;
- e_angle = -360;
- flag = 2;//changing flag when mouth is closed making the other condition true
- }
- }
- if (s_angle > 225 & s_angle <= 270 & e_angle >= -360 & flag == 2) { // when mouth is closed
- s_angle--; //decreasing starting angle
- e_angle += 2; //increasing ending angle
- if (s_angle <= 225) {
- s_angle = 225;
- e_angle = -270;
- flag = 1; //changing flag when mouth is open making the mouth open condition true
- }
- }
- } // down
- void left() {
- if (s_angle >= 135 & s_angle < 180 & e_angle <= -270 & flag == 1) { // when mouth is open
- s_angle++; //increasing starting angle
- e_angle -= 2; //decreasing ending angle
- if (s_angle == 180) {
- e_angle = -360;
- flag = 2; //changing flag when mouth is closed making the other condition true
- }
- }
- if (s_angle > 135 & s_angle <= 180 & e_angle >= -360 & flag == 2) { // when mouth is closed
- s_angle--; //decreasing starting angle
- e_angle += 2; //increasing ending angle
- if (s_angle <= 135) {
- s_angle = 135;
- e_angle = -270;
- flag = 1; //changing flag when mouth is open making the mouth open condition true
- }
- }
- }
- void right() {
- if (s_angle <= 45 & s_angle > 0 & e_angle < 360 & flag == 1) { // when mouth is open
- s_angle--; //decreasing starting angle
- e_angle += 2; //increasing ending angle
- if (s_angle == 0) {
- s_angle = 0;
- e_angle = 360;
- flag = 2; //changing flag when mouth is closed making the other condition true
- }
- }
- if (s_angle >= 0 & s_angle < 45 & e_angle > 270 & flag == 2) { // when mouth is closed
- s_angle++; //increasing starting angle
- e_angle -= 2; //decreasing ending angle
- if (s_angle >= 45) {
- s_angle = 45;
- e_angle = 270;
- flag = 1; //changing flag when mouth is open making the mouth open condition true
- }
- }
- }// right
- }// class ends
- PacPanel p = new PacPanel();
- void setFrame() { // setting the frame and making it visible
- jf.setSize(800, 600);
- jf.setVisible(true);
- }
- void addComponents() { // adding panel and keylistener in frame
- jf.add(p);
- jf.addKeyListener(this);
- }
- @Override
- PacPanel.s_angle = 45; //changing angles and making up condition true in PacPanels class
- PacPanel.e_angle = -270;
- PacPanel.direction = "up";
- PacPanel.flag = 1;
- }
- PacPanel.s_angle = 225; //changing angles and making down condition true in PacPanels class
- PacPanel.e_angle = -270;
- PacPanel.direction = "down";
- PacPanel.flag = 1;
- }
- PacPanel.s_angle = 135; //changing angles and making left condition true in PacPanels class
- PacPanel.e_angle = -270;
- PacPanel.direction = "left";
- PacPanel.flag = 1;
- }
- PacPanel.s_angle = 45; //changing angles and making down condition true in PacPanels class
- PacPanel.e_angle = 270;
- PacPanel.direction = "right";
- PacPanel.flag = 1;
- }
- }
In the end we simply provide the null body implementation for the remaining functions of the keylistener interface and then write our main function in which we make our pacman object , set the frame and add the components in the frame. After that we close our main as followed by the class. Now our animation is complete
OUTPUT:
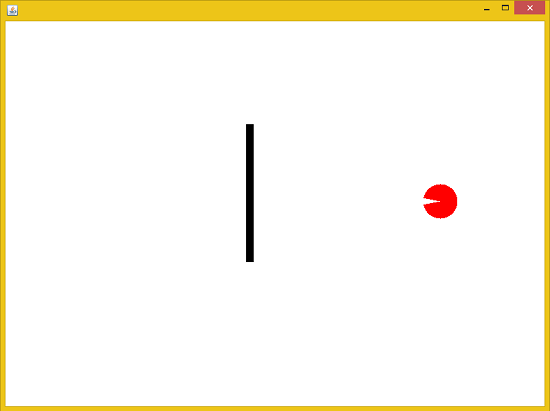