TUTORIAL NO 7
JAVA Catch the Eggs Game Programming part2
In this tutorial you will learn:
1.Game Programming
2.CardLayout
3.Changing screens in the game
4.Internal Classes
5.Swing Animations
6.Event handling
7.JAVA awt
8.JAVA swing
9.Adapters
In this tutorial I will continue coding my previous tutorial and write the GamePanel class which we left empty in our previous tutorial. Please go to the previous tutorial using this link
http://www.sourcecodester.com/tutorials/java/7488/java-catch-eggs-game-programming-part1.html
1.IMPORT STATEMENTS
First of all write these import statements in your .java file
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.event.*;
import java.util.*; // new statement added in this tutorial
We require event import for mouse click and keyboard buttons, awt and swing imports for visual design and animation. Till now we have already setup our classes and linked everything together right now we need to write our gamePanel class to make the main game. As the name suggests you need to catch the eggs in order to gain points within 59seconds. So lets start writing our GamePanel class.
2.SETTING UP THE GAMEPANEL CLASS
class GamePanel
extends JPanel{
Image gameOverbkg
= new ImageIcon("images\\gameOverbkg.png").
getImage();
Image tempbkg
; //temporary background
int x_basket,y_basket; //basket x and y coordinates
int x_egg,y_egg; // x and y coord of egg
Random rand
= new Random(); // for randomizing xcoord of eggs
int pointsCount = 0; //for counting points
int timeleft = 59; //to show remaining time
int counter = 0; //to decrement time slowly
boolean gameOver = false; //to check if the game is over
First of all we need to include the required images that we need in this panel. We need game background image. Basket image to catch the eggs and an egg image to show the egg. Other than that we need a gameOver image to show when the game is over. So we first include the required images from our images folder. Then we created a temporary image object for displaying the background image according to the status of the game. After that we declared variables for x and y coordinates of basket and egg and a random class object to randomize the falling of the eggs. After that we declared the counter for points and time (Remember counter variable is just for slowing down the time). Here we could have used a proper timer class but to keep things simple we are not going into that right now.
•WRITING THE CONSTRUCTOR
GamePanel(){
setLayout(null);
setFocusable(true);
tempbkg = gamebkg;
x_basket = 450; y_basket = 600;
x_egg = (int)rand.nextInt(1000);
y_egg = 0;
time
= new JLabel("Time: 59");
time.setBounds(20, 10, 50, 20); //setting the time label on screen
points
= new JLabel("Points: 0");
points.setBounds(100,10,100,20);
/*adding both components in jpanel*/
add(time);
add(points);
if(ke.getKeyCode() == ke.VK_LEFT & x_basket>10){
x_basket-=10;
repaint(); // redraw at new position
}
if(ke.getKeyCode() == ke.VK_RIGHT & x_basket<1000){
x_basket+=10; // redraw at new position
repaint();
}
}//end keypressed
});
}//end constructor
In the constructor we first set the Layout to null and get the focus on the panel for the first time. After that we set the background object to show the required background. Then we set the values of x and y coordinates of eggs and basket. Then we set the labels on the screen and in the end we added the keyListener to the panel. In the key listener we check which key is pressed and increment and decrement our x axis accordingly to move the basket. After that we repaint the screen to draw everything at new position.
•
WRITING THE FALLEGG FUNCTION
void fallEgg(){
if(y_egg >=650){ //when one egg has completely fallen
y_egg = 0; //set the y cord of next egg to 0
x_egg = rand.nextInt(1000); // randomize next eggs x coord
}
else
y_egg++; //otherwise fall the egg down
}//end fallEgg
Here we wrote the function for the egg to fall from top to the button i.e 650px then if the previous eggs coordinate is greater than 650 we call the next egg by setting the y coordinate to zero and randomizing the x coordinate. Other wise we will increment y axis of egg to fall it down.
•WRITING THE UPDATETIME
void updateTime(){
counter++;
if(counter == 100) //we count to 60 and then dec timeleft by 1 for slowing speed
{
timeleft--; //dec time left after 60 counts
counter = 0; //reset counter
}
time.setText("Time:"+timeleft);
}//end updateTime
In this function we simply update decrement the time left according to the counter value and set the time to the label. Here the counter is just to slow down the speed of the timer (NOTE: this is not an actual second for that you need either timer class or sleep function).
•
WRITING THE DETECTCOLLISION FUNCTION
void detectCollision(){
Rectangle basketRect
= new Rectangle(x_basket,y_basket,
100,
65); //making a rectangle on the basket
if(eggRect.intersects(basketRect)){
pointsCount+=5; // give 5 points on each catch
points.setText("Points:"+pointsCount); // set the count
y_egg = 0; // for next egg
x_egg = rand.nextInt(1000); // again randomizing x axis of egg
}
}//end collision detection
In this function we simply created invisible rectangles of the size of egg and basket and passed them exactly same coordinates and sizes. After that we just checked for collision of those invisible rectangles by the intersects function. In the if statement we simply incremented the points and started the fall for the next egg.
•
WRITING THE GAMEOVER FUNCTION
void checkGameOver(){
if(timeleft <= 0)
{
JLabel yourScore
= new JLabel("Your SCORE :" + pointsCount
);
tempbkg = gameOverbkg;
yourScore.setBounds(400, 400, 200, 100);
gameOver = true;
yourScore.
setForeground(Color.
RED);
add(yourScore);
}
}//end gameOver
In this function we simply check for the time left and set the label and value of gameOver Boolean variable accordingly.
•WRITING THE PAINTCOMPONENT FUNCTION
super.paintComponent(g);
g2d.drawImage(tempbkg,0,0,null); //game background
checkGameOver();
if(gameOver == false){
setFocusable(true);
grabFocus();
updateTime();
fallEgg();
detectCollision();
g2d.drawImage(egg, x_egg, y_egg,null); //drawing egg at new position
g2d.drawImage(basket, x_basket, y_basket, null); //drawing basket
}
repaint();
}//end paintComponent
}//end class
In the game panel first of all we draw the background image by using the temporary variable which sets according to the status of the game. Then we check for the game over if that is false we proceed and set the focus for the key listener. Then call our functions of update time , grab focus , egg fall and collision detection. After that we simply draw the basket and the egg according to the set coordiantes and re draw the screen. Here our GamePanel is complete. Now we can play our very simple catch the eggs game in java.
OUTPUT:
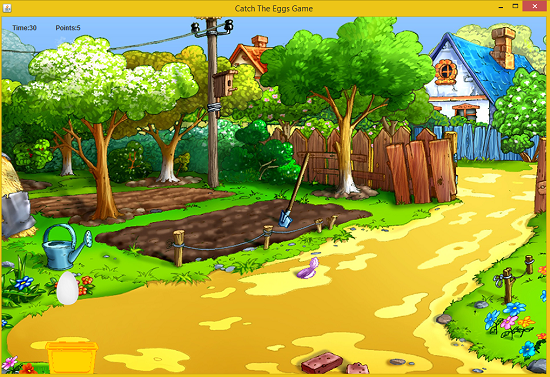