TUTORIAL NO 3
Antivirus using MultiThreading
In this tutorial you will learn:
1. MultiThreading
2. Recursion
3. Event handling
4. Layout Manager
5. JAVA awt basics
6. JAVA swing basics
7. Adapters
8. Anonymous classes
Sometimes there is a very basic virus in your system i.e a file spread in all the directories of your disk drive. It’s totally an unwanted file (You can say a virus ) and it may be sometimes hidden but you can see it in the task manager. So today I am going to teach you how to make a simple antivirus for that and in the next tutorial I will teach you how to make that virus so stay tuned. The User Interface
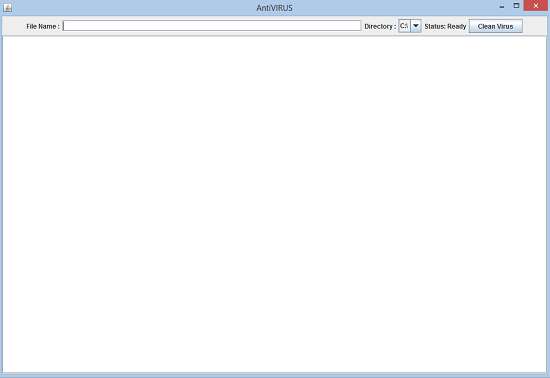
In this tutorial we will be working in JAVA SWING. The first thing that we are going to do is setting up a JFrame and adding the required panels and Components.
Basic step:
Download and install ECLIPSE and set up a JAVA PROJECT. Then create a new class and name it AntiVirus. Then follow the steps
1. IMPORT STATEMENTS
First of all write these import statements in your .java file
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
We require event import for mouse click , awt and swing imports for visual design. For this tutorial we will only be using one public class and we will be extending it from JFrame (i.e inheritance).
2.SETTING UP THE FRAME CLASS
int directories_count = 0;
JLabel file_label
= new JLabel("File Name :"); // for file name label
JLabel dir_label
= new JLabel("Directory :"); // for directory label
JLabel status
= new JLabel("Status: Ready"); // current status label
String file_name
; //for storing file name
String dir
; //for storing directory
boolean deleted = false; //for changing the status
create a public frame class by extending it from jframe and implementing the runnable interface for multithreading. Now create all the required variables. File array reference for getting the root directories then a counter to count the number of directories on your system. Then declaring all the text fields and labels according to the User Interface shown in the picture above. We need text fields and their labels. Other than that we need a label to set the status, scroll pane for the text area scroll bar, text area to show the traversed directories, combo box to show the directories on your system, then finally the cleanVirus button other than that we need to declare variables to store file name and directory and a Boolean to change the status whether the virus id found and deleted or not.
3. WRITING THE CONTSTUCTOR
We will do everything in a constructor so that the jframe gets setup whenever we create the object of that JFrame. Now writing the constructor.
AntiVirus() {
directories_count
= File.
listRoots().
length;//counting total disk drives
myfile
= File.
listRoots(); // a file object for storing root directories
for(int i=0;i<directories_count;i++)
jcb.addItem(myfile[i]); // adding directories in comboBox
add(up, "North"); //adding panel in north position
up.add(file_label); //first adding File Name label
up.add(t_field); // then text field to enter file name
up.add(dir_label); //directory label
up.add(jcb); // then comboBox
up.add(status); // status label
up.add(cleanVirus); // search button
setDefaultCloseOperation
(JFrame.
EXIT_ON_CLOSE);
setSize(1024, 700); //frame size
setVisible(true); //frame visibility
add(sp); // adding scroll pane
First of all we will count the number of directories and save the root directories in our file array. Then we will add all the directories name to the combo box. After that we will created another jpanel and add it to north position, then we added all the labels and the text fields as shown in the picture above in that panel. After that we setup the frame size and visibility.
public void mouseClicked
(MouseEvent me
) { //overriding
file_name = t_field.getText();// getting the text from text field
dir = jcb.getSelectedItem().toString(); // directory selected from combo box
dir +="\\"; //appending double slashes to dir e.g C:\\ status.setText("Finding Virus"); //setting the status
startThread(); //creating a new thread using this function
}
}); // end anonymous class and mouselistener
} // end constructor
In the mouselistener we created an anonymous MouseAdapter class (Remember: anonymous class is the one in which you only have to create one object). Now we override the mouse clicked function. The first thing we need to do is to get the file name from the text field and directory from the combo box. Then we set the status of our app. After that we called a function to start the thread and end the class ,listener and the constructor. The startThread() function is defined below.
public void startThread(){
t.start(); //starting thread
}
In this function we created an instance of thread class and pass it the runnable component of the class using this. Then we start the thread.
4. OVERRIDING THE RUN FUNCTION:
@Override
public void run() { // overriding of runnable interface
try{
DeleteVirus
(file_name,
new File(dir
)); //calling delete virus function
if (deleted == true)
status.setText("Virus Deleted"); // if file is deleted then set label
else
status.setText("No Virus found"); // otherwise no file is found }catch(Exception e){} //handling the exception
}//end run
After implementing the runnable interface we will override the run function and call the DeleteVirus function in it by passing the file name and directory to it’s parameters and enclosing it in a try catch block to catch any exception in the thread. We will define this function later. Now we need to set the status after all the directories are traversed so we set the status according to the value of our boolean variable, depending on the file if it’s deleted or not.
4. DEFINING THE DELETEVIRUS FUNCTION:
File[] list
= v.
listFiles(); //listing files
if (list != null) //checking so that nullpointerException cannot occur
{
for (File subfile
: list
) { //listing every subdirectory
t_area.append(""+v.getAbsolutePath()); //showing in text area
t_area.append("\n");
File v_file
= new File(v.
getAbsolutePath(), file_name
); // getting the path of file
if(v_file.isFile()) //v_file points to a file
{
v_file.delete(); // delete that file which has specified name
deleted = true; // turn boolean to true to set the label later
}
if (subfile.isDirectory()) {
DeleteVirus(v_dir, subfile); //if more directories then search in them
}
}//end for
}//end if
}//end DeleteVirus
Now we define the delete virus function it takes two parameters file directory and file reference. First of all we list the files in the directories and store it in our list of files array. Then if we check if the list of files in the directories in not equal to null so that we don’t get null pointer exception. Then we start going through all the files and keep appending their path to the text area. After that we created a temporary reference v_file for the loop with user defined directory and user defined file name and check if the current file matches that file or not. If does we delete that file otherwise we move on and check whether it’s a directory or not. If it is we move in that directory by calling the function again other wise we move on to are next iteration of the loop and check.
4. DEFINING THE MAIN FUNCTION
public static void main
(String args
[]) {
new AntiVirus(); // creating the object of antivirus frame class
}// end main
}// end filefinder class
In the main function we only created the object of the JFrame class and our application is complete.
DO READ THE NEXT TUTORIAL FOR THE VIRUS.
5. OUPUT: