List Management in C#
Submitted by donbermoy on Saturday, July 26, 2014 - 09:57.
Today in C#, I will teach you how to create a simple tool in C# to manage lists and listboxes.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio 2010: Go to File, click New Project, and choose Windows Application.
2. Next, insert one textbox named TextBox1, Four buttons named Button1 for adding items in listbox, Button2 for importing lists, Button3 for exporting lists, and Button4 for removing items in Listbox. Add also one listbox named ListBox1. You must design your interface like this:
3. Write a function that will take a file input from the user which will hold a list of information (one per line). We will then make the function verify the file is there, and is not nothing/empty/null, followed by writing each line to a listbox.
4. For Button2_Click, put this code below for importing the lists.
5. Now, welet the user select a saving text file, then use a new streamwriter (not streamreader like before) to write each item within the listbox to the file on a new line, using the .writeline function to import the lists.
6. Now, call the export function in Button3_Click.
7. For Button1_Click to add items in the listbox, put this code below.
8. For Button4_Click to remove items in the listbox, put this code below.
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
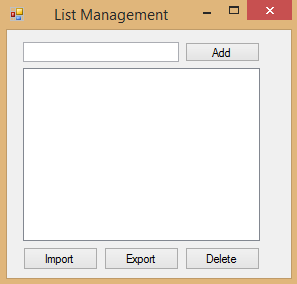
- private object import()
- {
- {
- fo.Filter = "Text Files | *.txt"; //Set properties for OpenFileDialog, fo.
- fo.FilterIndex = 1;
- fo.RestoreDirectory = true;
- fo.ShowDialog();
- if (fo.FileName != null&& fo.CheckFileExists)
- {
- using (System.IO.StreamReader sr = new System.IO.StreamReader(fo.FileName)) //Create new streamreader to selected file
- {
- while (sr.Peek != -1) //While not at end of file
- {
- ListBox1.Items.Add(sr.ReadLine()); //Add line to listbox
- }
- }
- }
- }
- }
- private void Button2_Click(object sender, EventArgs e)
- {
- import();
- }
- private object export()
- {
- {
- fs.RestoreDirectory = true; //Set properties for savefiledialog, fs.
- fs.ShowDialog();
- if (fs.FileName != null&& fs.CheckFileExists && fs.CheckPathExists)
- {
- using (System.IO.StreamWriter sw = new System.IO.StreamWriter(fs.FileName)) //Create new streamwriter to selected file
- {
- foreach (string item in ListBox1.Items) //Iterate through listbox1.items
- {
- sw.WriteLine(item); //Write line
- }
- }
- }
- }
- }
- private void Button3_Click(object sender, EventArgs e)
- {
- export();
- }
- private void Button1_Click(object sender, EventArgs e)
- {
- ListBox1.Items.Add(TextBox1.Text);
- }
- private void Button4_Click(object sender, EventArgs e)
- {
- ListBox1.Items.RemoveAt(ListBox1.SelectedIndex);
- }
Add new comment
- 113 views