Today, i will teach you how to create a program that converts binary into text using C#. This is a continuation of my other tutorial in C# entitled
Text to Binary Conversion using C#.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to
File, click
New Project, and choose
Windows Application. Name the program
Convert Binary to text.
2. Next, add two TextBox named
TextBox1 to input a binary digit and
TextBox2 for displaying the text conversion of the binary you have inputted. Add also a button named
Button1. Design your layout like this one below:
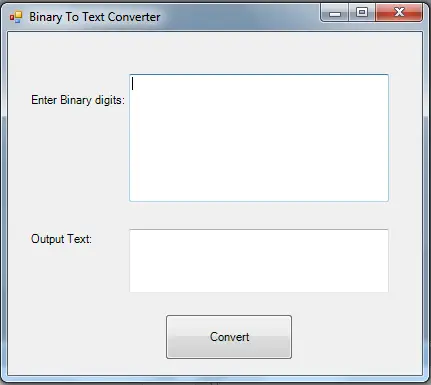
3. Put this code to your Button1_Click. This will display the conversion of the binary to text.
Initialize tempString as String to nothing and a Character as String that holds a regular expression of the textbox1 to 1 or 0 only as our System.Text.RegularExpressions.Regex as we use the Replace method.
string tempString = "";
string Character = System.Text.RegularExpressions.Regex.Replace(TextBox1.Text, "[^01]", "");
Next, we declare a Bytes variable that have the length of 8 bits as we have the code ((Character.Length / 8) - 1).
byte[] Bytes
= new byte[(Character
.Length / 8) - 1 + 1];
Then we will have a For Next loop that we initialized an Index variable as an integer to start from 0 to the Bytes variable length minus 1 because it is an array of bytes (Note: array starts at 0 so we minus 1 it). Next, the Bytes array index will be equal to the conversion of bytes from the characters inputted in textbox1.
for (int Index = 0; Index <= Bytes.Length - 1; Index++)
{
Bytes[Index] = Convert.ToByte(Character.Substring(Index * 8, 8), 2);
}
The tempString variable will get the equivalent string of the variable Bytes. And then the value of the binary inputted will now be equal to the variable tempString and will be displayed in textbox2.
tempString = (string) (System.Text.ASCIIEncoding.ASCII.GetString(Bytes));
TextBox2.Text = tempString;
}
Full source code:
using System.Diagnostics;
using System;
using System.Windows.Forms;
using System.Collections;
using System.Drawing;
using System.Data;
using System.Collections.Generic;
namespace Convert_Binary_to_text
{
public partial class Form1
{
public Form1()
{
InitializeComponent();
}
public void Button1_Click(System.Object sender, System.EventArgs e)
{
string tempString = "";
string Character = System.Text.RegularExpressions.Regex.Replace(TextBox1.Text, "[^01]", "");
byte[] Bytes
= new byte[(Character
.Length / 8) - 1 + 1]; for (int Index = 0; Index <= Bytes.Length - 1; Index++)
{
Bytes[Index] = Convert.ToByte(Character.Substring(Index * 8, 8), 2);
}
tempString = (string) (System.Text.ASCIIEncoding.ASCII.GetString(Bytes));
TextBox2.Text = tempString;
}
}
}
Output:
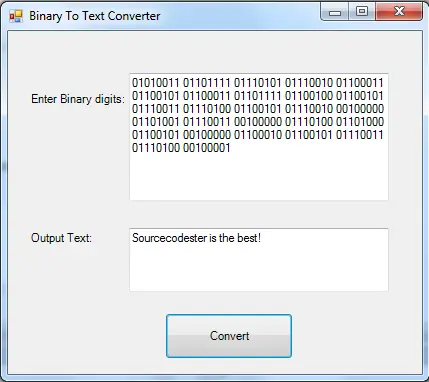
Download the code and try it! :)
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:
[email protected]
Add and Follow me on Facebook:
https://www.facebook.com/donzzsky
Visit and like my page on Facebook at:
https://www.facebook.com/BermzISware