In this tutorial I’m going to show you how to create an application that will display the selected row from the datagridview into textbox. To start with this application we will create first a database in Microsoft access 2003 and we will name it as “studentdb” for Student Database. Then create a table named “tblstudent” and create a field that will look like as shown below.
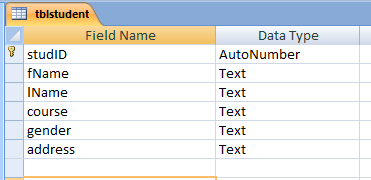
After this, we will create a new windows form project in C#, then save it as “stud_info”. Then we will add controls to the form such as button, textboxes, datagridview and labels and designed that looks like as shown below.
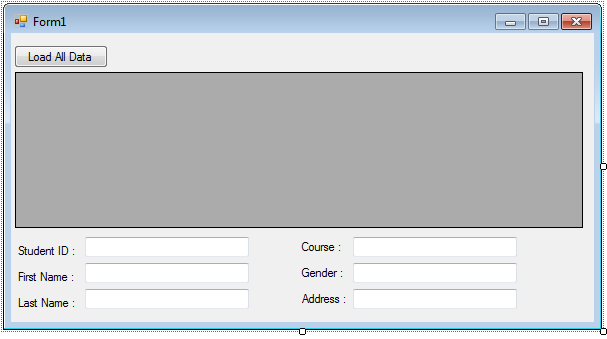
Before we proceed, make sure that our database is placed in our bin directory. At this time, we will add functionality to our application. First double click the form and add the following code above the public Form1() function.
//declare new variable named dt as New Datatable
DataTable dt
= new DataTable
();
//this line of code used to connect to the server and locate the database (studentdb.mdb)
static string connection = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source= " + Application.StartupPath + "/studentdb.mdb";
OleDbConnection conn
= new OleDbConnection
(connection
);
After this, we will add functionality to our “Load All Data” button. By adding these following codes.
This code below will get all the record found in the database table called “tblstudent” and display it into the datagridview.
//create a new datatable
//create our SQL SELECT statement
string sql = "Select * from tblstudent";
//then we execute the SQL statement against the Connection using OleDBDataAdapter
OleDbDataAdapter da
= new OleDbDataAdapter
(sql, conn
);
//we fill the result to dt which declared above as datatable
da.Fill(dt);
//then we populate the datagridview by specifying the datasource equal to dt
dataGridView1.DataSource = dt;
Then when you run the application and try to click the “Load All Data” button. It will display like as shown below.
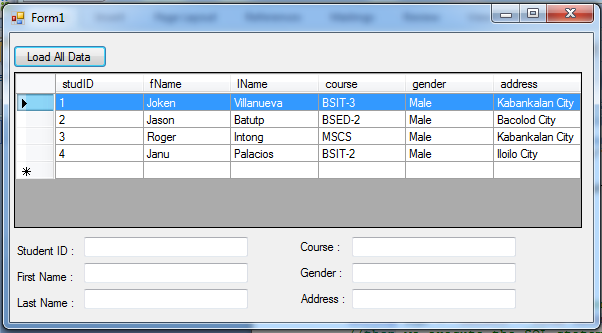
Next, we will add functionality when the user click the selected row and display to the corresponding textbox. First select the datagridview and click the events under the window of the properties and select “CellClick” that looks like as shown below.
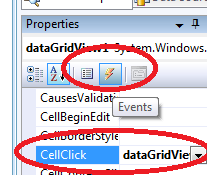
Then add the following code that will get the data from the selected row and display in the textbox provided.
//it checks if the row index of the cell is greater than or equal to zero
if (e.RowIndex >= 0)
{
//gets a collection that contains all the rows
DataGridViewRow row = this.dataGridView1.Rows[e.RowIndex];
//populate the textbox from specific value of the coordinates of column and row.
txtid.Text = row.Cells[0].Value.ToString();
txtfname.Text = row.Cells[1].Value.ToString();
txtlname.Text = row.Cells[2].Value.ToString();
txtcourse.Text = row.Cells[3].Value.ToString();
txtgender.Text = row.Cells[4].Value.ToString();
txtaddress.Text = row.Cells[5].Value.ToString();
}
After this, you test it by pressing the “F5” or clicking the start button. Then when you run this application, make sure that the user first click the “Load all Record” button, then that’s the time that’s the user can click the selected row to display in the textbox.
And running application is look like as shown below.
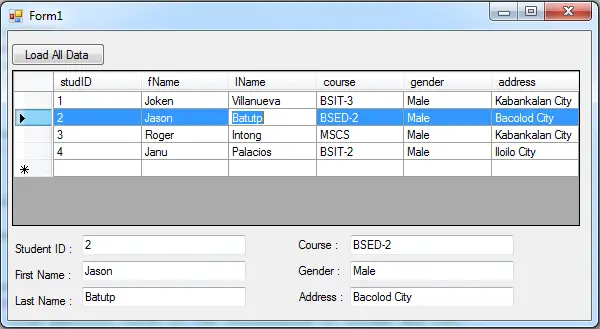
And here’s all the code used for this application.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.OleDb;
namespace student_info
{
public partial class Form1 : Form
{
//declare new variable named dt as New Datatable
DataTable dt
= new DataTable
();
//this line of code used to connect to the server and locate the database (usermgt.mdb)
static string connection = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source= " + Application.StartupPath + "/studentdb.mdb";
OleDbConnection conn
= new OleDbConnection
(connection
);
public Form1()
{
InitializeComponent();
}
private void btnload_Click(object sender, EventArgs e)
{
//create a new datatable
//create our SQL SELECT statement
string sql = "Select * from tblstudent";
//then we execute the SQL statement against the Connection using OleDBDataAdapter
OleDbDataAdapter da
= new OleDbDataAdapter
(sql, conn
);
//we fill the result to dt which declared above as datatable
da.Fill(dt);
//then we populate the datagridview by specifying the datasource equal to dt
dataGridView1.DataSource = dt;
}
private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
{
//it checks if the row index of the cell is greater than or equal to zero
if (e.RowIndex >= 0)
{
//gets a collection that contains all the rows
DataGridViewRow row = this.dataGridView1.Rows[e.RowIndex];
//populate the textbox from specific value of the coordinates of column and row.
txtid.Text = row.Cells[0].Value.ToString();
txtfname.Text = row.Cells[1].Value.ToString();
txtlname.Text = row.Cells[2].Value.ToString();
txtcourse.Text = row.Cells[3].Value.ToString();
txtgender.Text = row.Cells[4].Value.ToString();
txtaddress.Text = row.Cells[5].Value.ToString();
}
}
private void Form1_Load(object sender, EventArgs e)
{
}
}
}