How to Display the Data in a Textbox Using C#
Submitted by janobe on Sunday, September 1, 2019 - 22:27.
In this tutorial, I will teach you how to display the data in a textbox using c#. This method has the ability to retrieve the data in the database and display it in the textbox. It is very useful, especially when you want to retrieve specific data in the database. Hope this tutorial will help you to solve your current problem in programming.
The complete source code is included. You can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating a Database
Create a database in the “PHPMyAdmin” and named it “tuts_dbperson”. After that, execute the following query to create a table in the database that you have created.
Insert the data in the table.
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application in c#.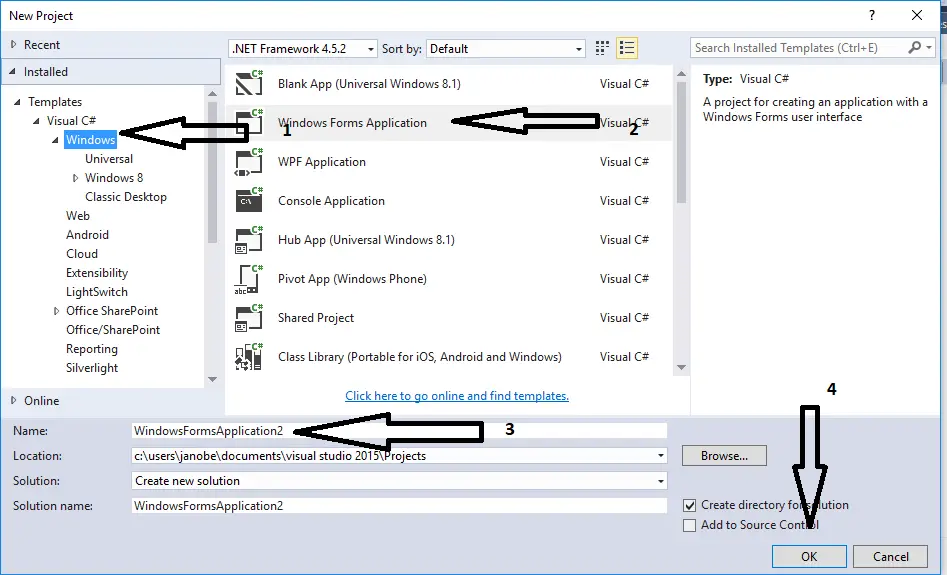
Step 2
Add two textboxes, and two labels after that do the form just like shown below.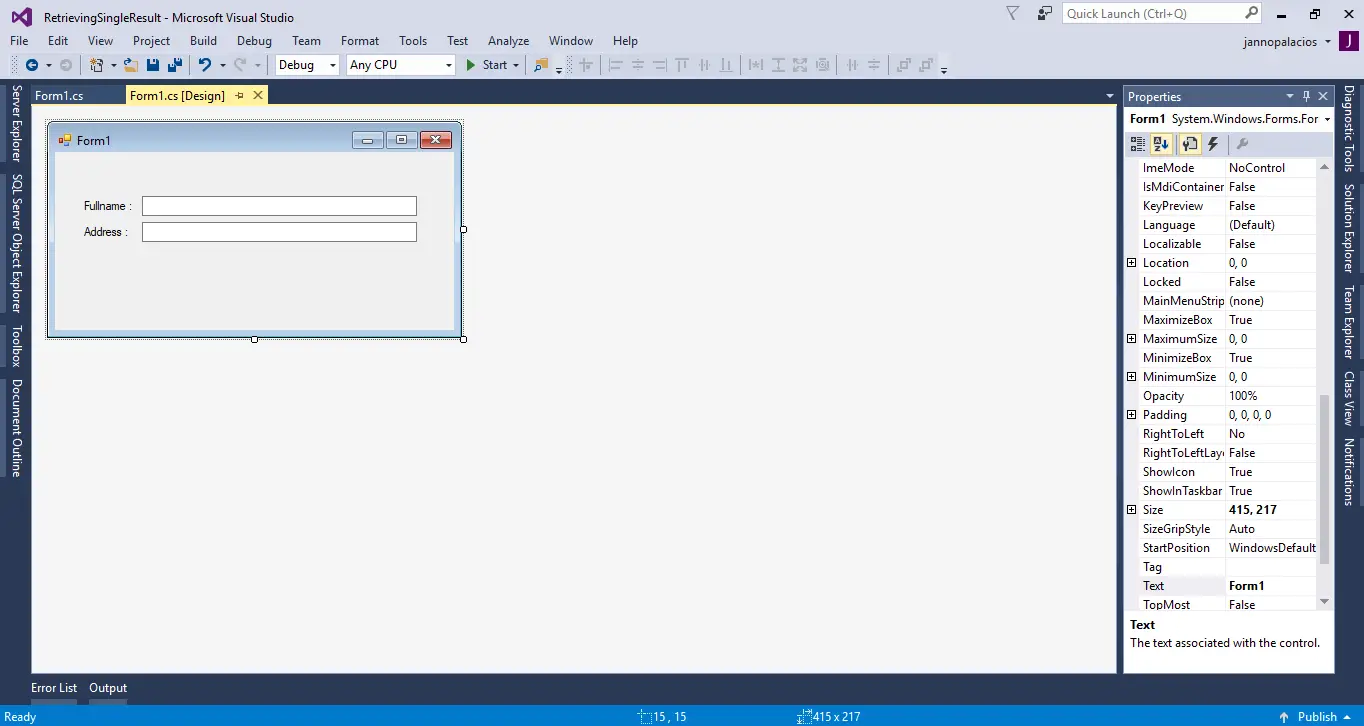
Step 3
Add MySQL.Data.dll as references.Step 4
Press F7 to open the code editor. In the code editor, add a namespace to accessMySQL
libraries.
- using MySql.Data.MySqlClient;
Step 5
Create a connection between c# and MySQL database and declare all the classes that are needed.- MySqlConnection con = new MySqlConnection("server=localhost;user id=root;password=;database=tuts_dbperson;sslMode=none");
- MySqlDataAdapter da;
- MySqlCommand cmd;
- DataTable dt;
- string sql;
Step 6
Create a method for retrieving data in the database and display it on the textbox.- private void RetrieveSingleResult(string sql)
- {
- try
- {
- con.Open();
- int maxrow;
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- maxrow = dt.Rows.Count;
- if(maxrow > 0)
- {
- textBox1.Text = dt.Rows[0].Field<string>("Fullname");
- textBox2.Text = dt.Rows[0].Field<string>("Address");
- }
- }
- catch(Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- con.Close();
- da.Dispose();
- }
- }
Step 7
Write the following codes to display the data in the textbox in the first load of the form.- private void Form1_Load(object sender, EventArgs e)
- {
- sql = "SELECT * FROM `tblperson` WHERE PersonID=2";
- RetrieveSingleResult(sql);
- }
Add new comment
- 1457 views