How to Create an Image Slide Show in C#
Submitted by janobe on Thursday, August 15, 2019 - 22:06.
In this tutorial, I will teach you how to create an image slide show in c#. This method has the ability to preview the list of images in a picture box one at a time. This is a simple method that can be used in a school project. See the procedure below to see how it works.
Note : The location of pictures is in the bin folder of the project.
The complete source code is included. You can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel fre
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.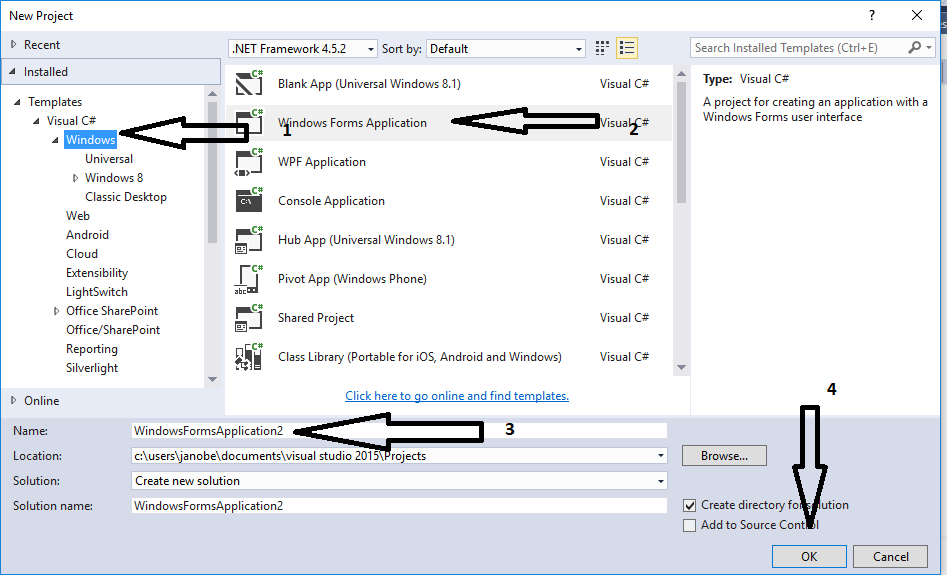
Step 2
Add a picture box, a timer and two buttons inside the form. Then, do the form just like shown below.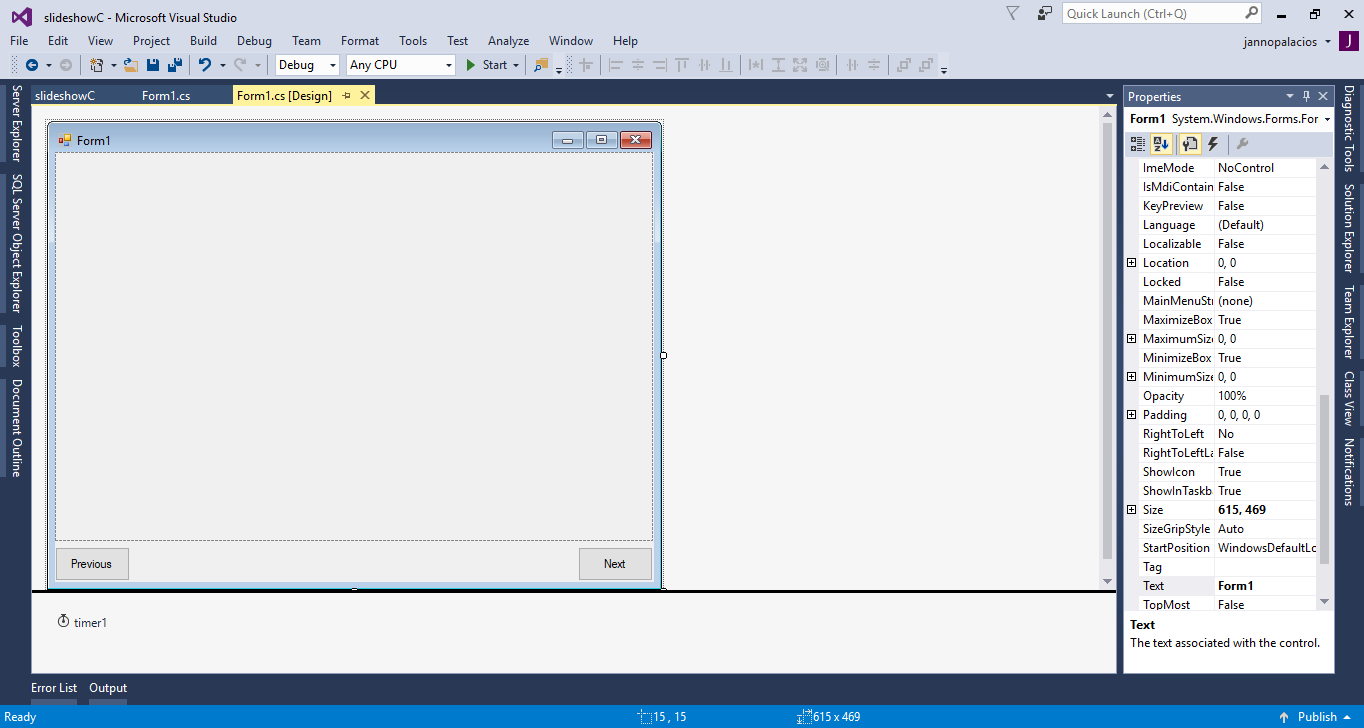
Step 3
Press F7 to open the code editor. In the code editor, declare a string array and an integer that equals to zero.- int i = 0;
Step 4
Double click the form and do the following code for placing the first image in the picture box in the first load of the form.- pictureBox1.Image = Image.FromFile(Application .StartupPath + "//images//" + pics[0]);
Step 5
Write the following codes for the slide show of pictures when timer is start.- private void timer1_Tick(object sender, EventArgs e)
- {
- //'Set this formula to increment i by 1
- i += 1;
- //'Set a validation of pictures
- if(pics.Length == i)
- {
- i = 0;
- }
- //'Set to Load the image.
- pictureBox1.Image = Image.FromFile(Application.StartupPath + "//images//" + pics[i]);
- }
Step 6
Write the following code for the next button- private void button2_Click(object sender, EventArgs e)
- {
- //'Disable timer to prevent the next image from loading..
- timer1.Enabled = false;
- //'Set this formula to increment i by 1
- i += 1;
- //'Set a validation of pictures
- if( pics.Length == i)
- {
- i = 0;
- }
- //'Set to Load the image.
- pictureBox1.Image = Image.FromFile(Application.StartupPath + "//images//" + pics[i]);
- //'Re-Enable timer to resets the image loads in 5 seconds..
- timer1.Enabled = true;
- timer1.Interval = 5000;
- }
Step 7
Write the following code for the previous button.- private void button1_Click(object sender, EventArgs e)
- {
- //'Disable timer to prevent the next image from loading..
- timer1.Enabled = false;
- //'Set this formula to increment i by 1
- i -= 1;
- //'Set a validation of pictures
- if (pics.Length == i)
- {
- i = 0;
- }
- //'Set to Load the image.
- pictureBox1.Image = Image.FromFile(Application.StartupPath + "//images//" + pics[i]);
- //'Re-Enable timer to resets the image loads in 5 seconds..
- timer1.Enabled = true;
- timer1.Interval = 5000;
- }
Add new comment
- 3132 views