The Easiest Way to Create a Textbox Programmatically in C#
Submitted by janobe on Thursday, March 28, 2019 - 16:44.
In this tutorial, I will teach you how to create a textbox programmatically in c#. This method has the ability to add an object in the form by clicking a button. It is also demonstrating how to get the collection of Controls within the control. Let's begin.
Step 6
Write the following code for creating a textbox when the button is clicked.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.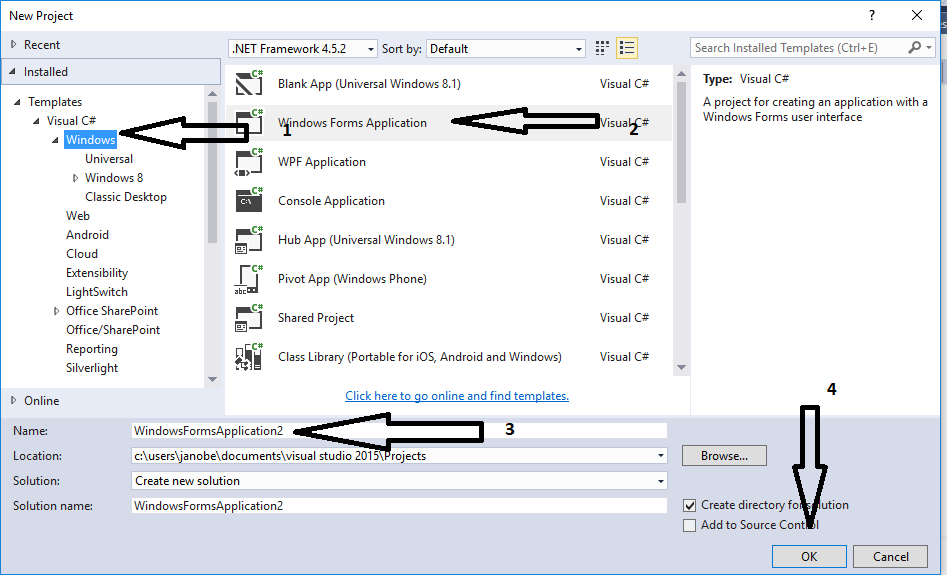
Step 2
Do the form just like shown below.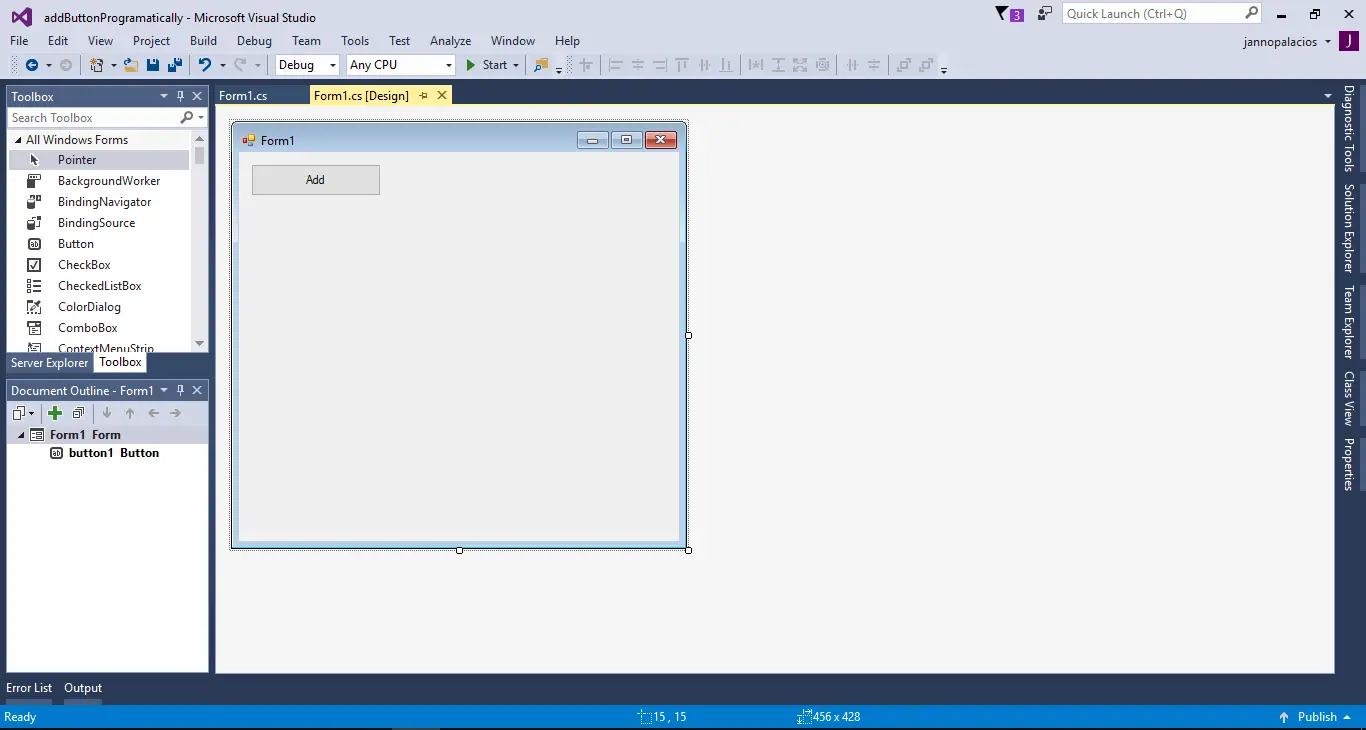
Step 3
Press F7 to open the code editor. In the code editor, declare private variables for the location and count control.- private int count_control = 0;
Step 4
Create a method for creating a textbox.- private void createTexbox()
- {
- // 'INCREMENT THE COUNT_CONTROL.
- count_control += 1;
- //'CHECKING IF THE TEXTBOX HAS REACHED TO 6 OR NOT
- if (count_control <= 6)
- {
- // initialize a new object
- //set a object properties
- txt_new.Name = "txt " + count_control.ToString();
- txt_new.Text = "textbox " + count_control.ToString();
- txt_new.Width = 350;
- location_control.Y += txt_new.Height + 10;
- // create a textbox
- Controls.Add(txt_new);
- }else
- {
- DialogResult result = MessageBox.Show("You've reached 6 controls. Do you want to clear controls to start again?", "Proceed", MessageBoxButtons.OKCancel, MessageBoxIcon.Information);
- if(result==DialogResult.OK)
- {
- //clear the controls
- Controls.Clear();
- //control return to 0
- count_control = 0;
- // set a new point for controls
- //create a new textbox
- createTexbox();
- // add button again
- Controls.Add(button1);
- }
- }
- }s
Step 5
Call the method that you have created in the first load of the form.- private void Form1_Load(object sender, EventArgs e)
- {
- createTexbox();
- }
- private void button1_Click(object sender, EventArgs e)
- {
- createTexbox();
- }
Add new comment
- 558 views